How Selenium works(elements status/2:Chrome)
Google Chrome
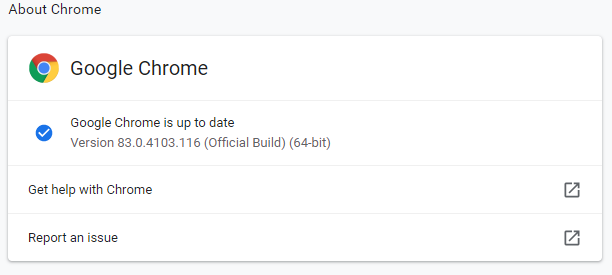
Button
Click
First, enter the id of the element in the locator field and press the “id" button. Next, press the “click" button.
If successful, the executed code of Python is displayed.
Normal
It is clickable.
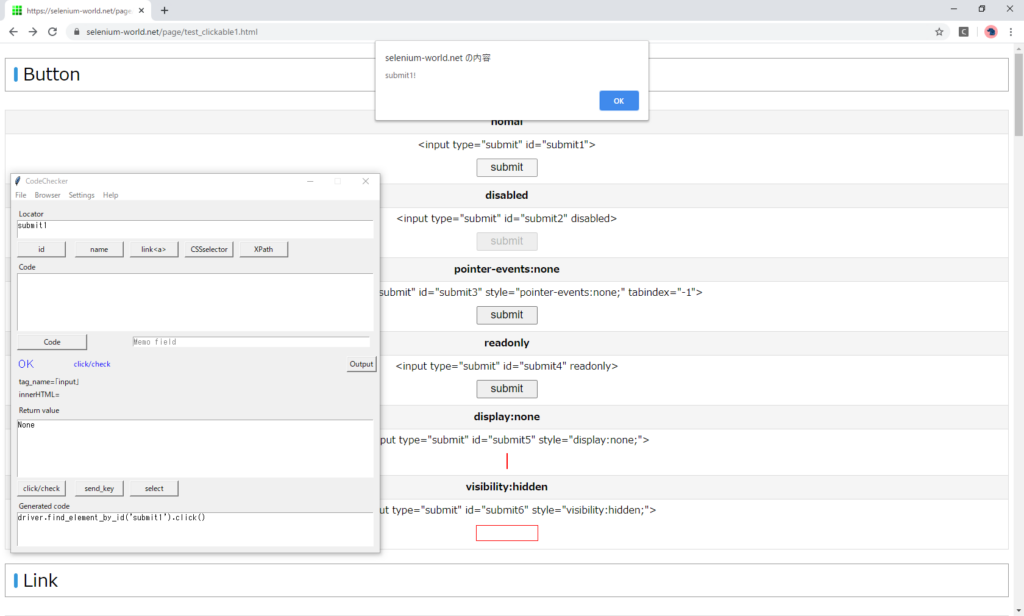
Inactive(disabled)
It is clickable, but the screen does not respond.
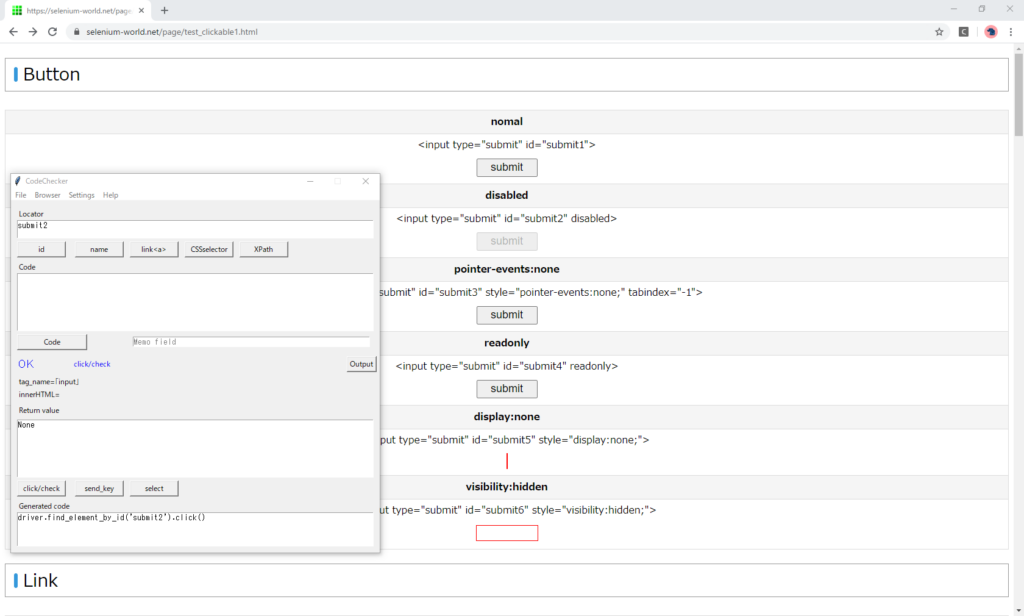
Inactive(pointer-events:none)
Exception occurred.
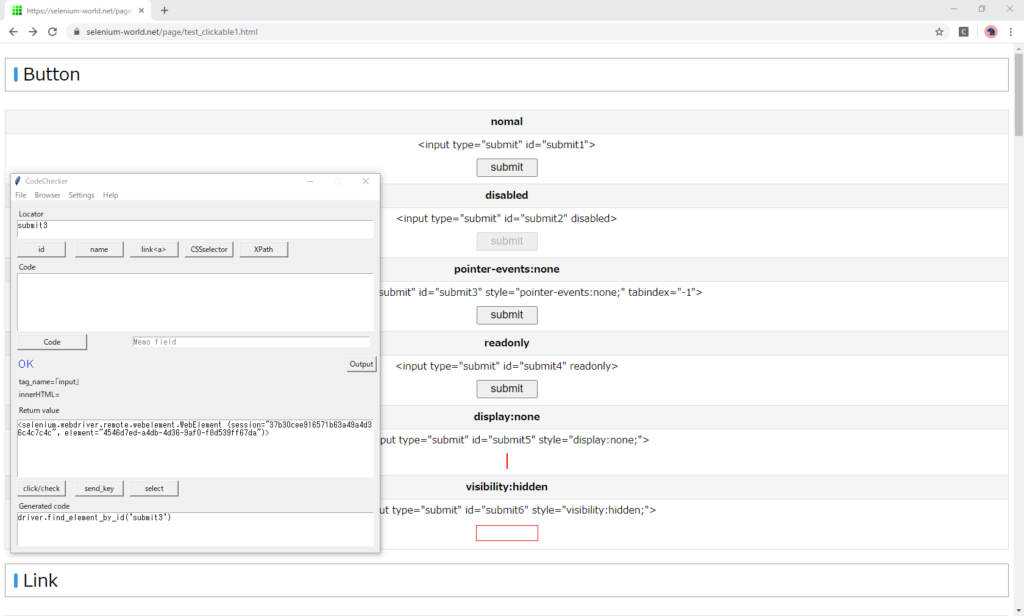
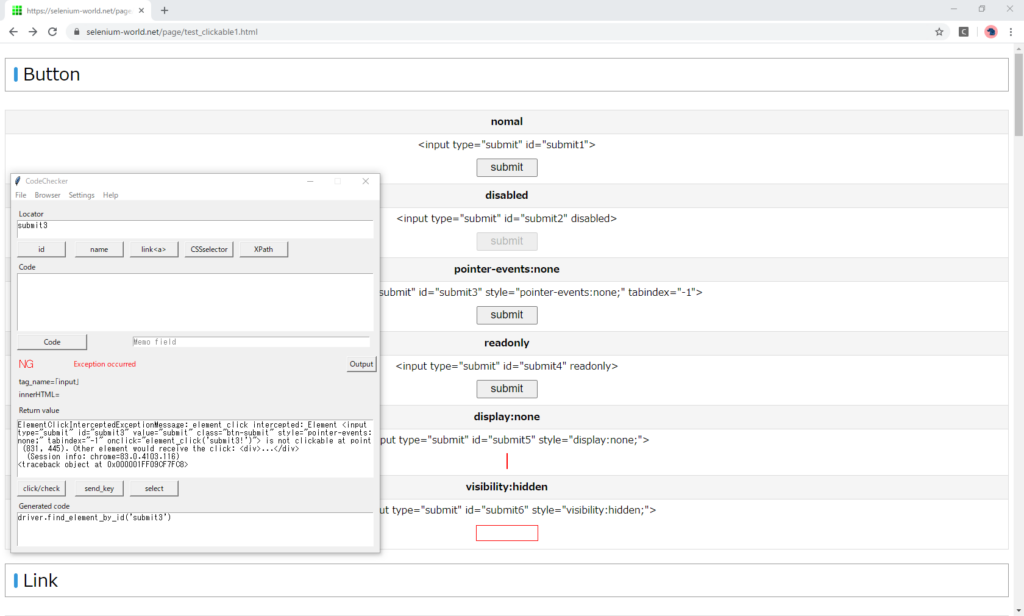
Message
ElementClickInterceptedExceptionMessage: element click intercepted: Element <input type="submit" id="submit3" value="submit" class="btn-submit" style="pointer-events:none;" tabindex="-1" onclick="element_click('submit3!')"> is not clickable at point (831, 445). Other element would receive the click: <div>...</div>
(Session info: chrome=83.0.4103.116)
<traceback object at 0x0000019291713EC8>
Read-only(readonly)
A click event occurs.
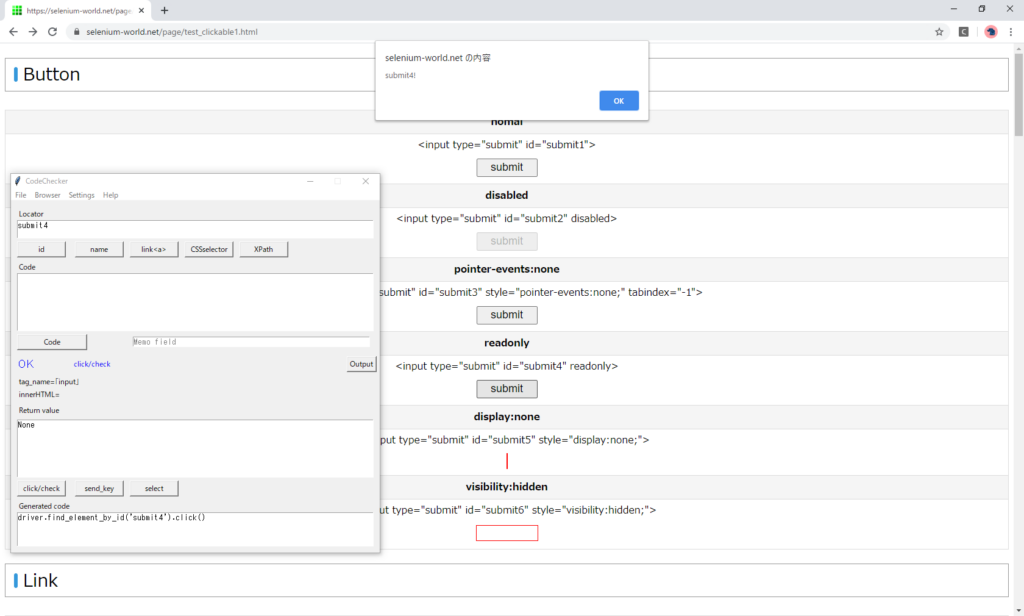
Hidden(display:none)
Exception occurred.
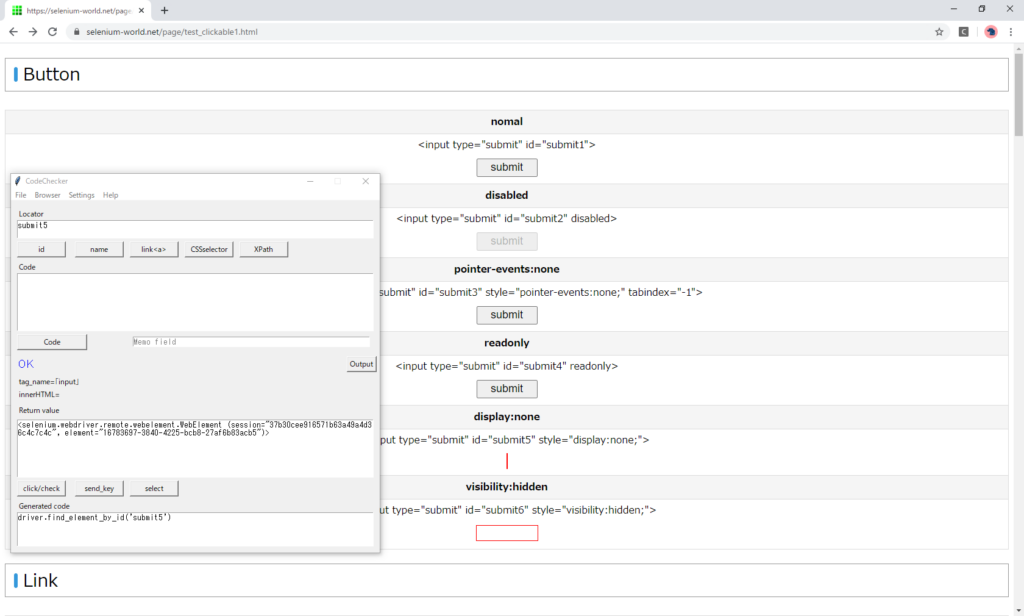
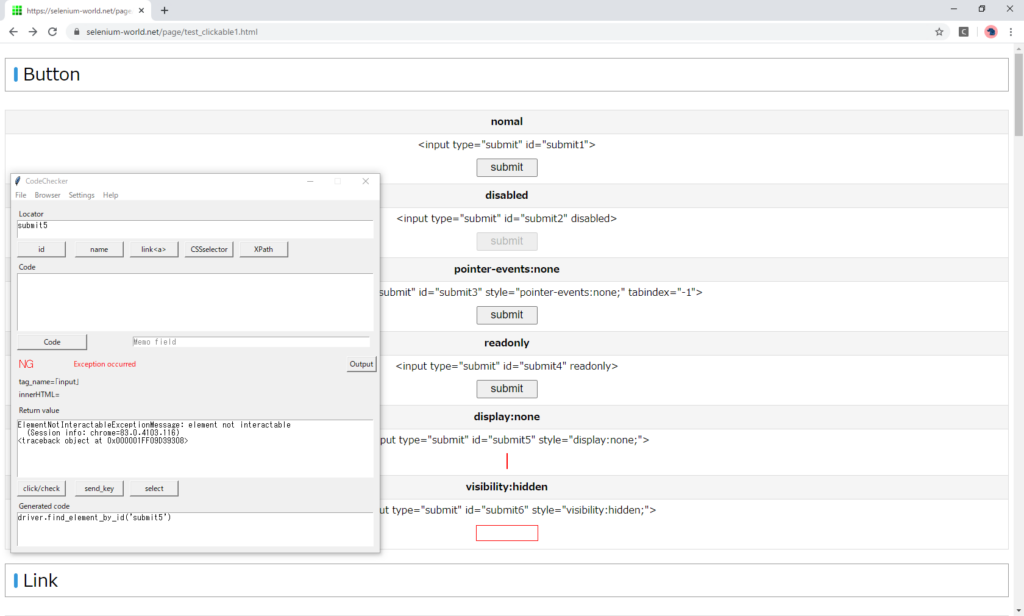
Message
ElementNotInteractableExceptionMessage: element not interactable
(Session info: chrome=83.0.4103.116)
<traceback object at 0x000001929171CB48>
Hidden(visibility:hidden)
Exception occurred, just like “display:none".
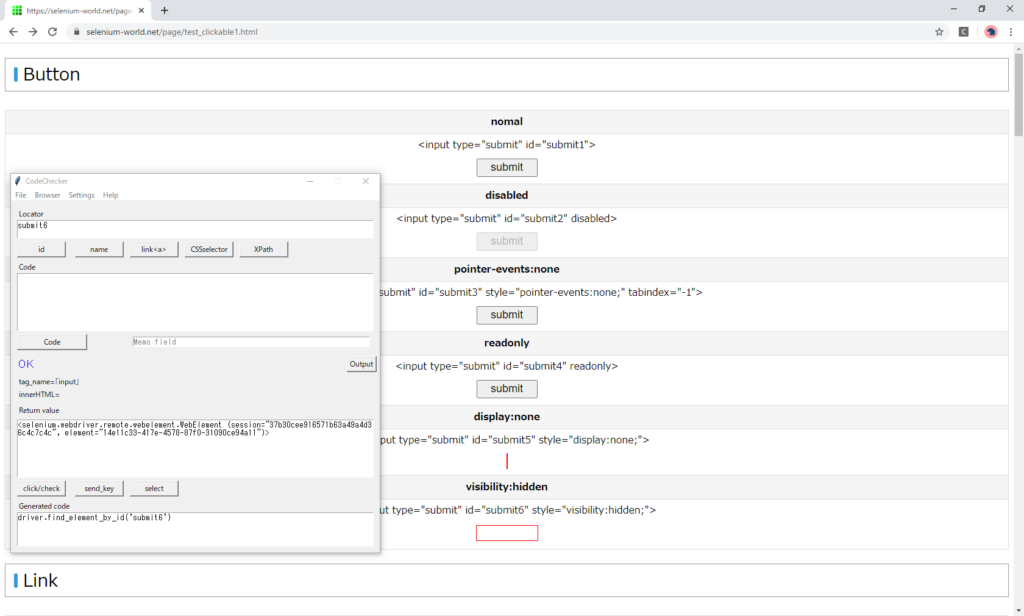
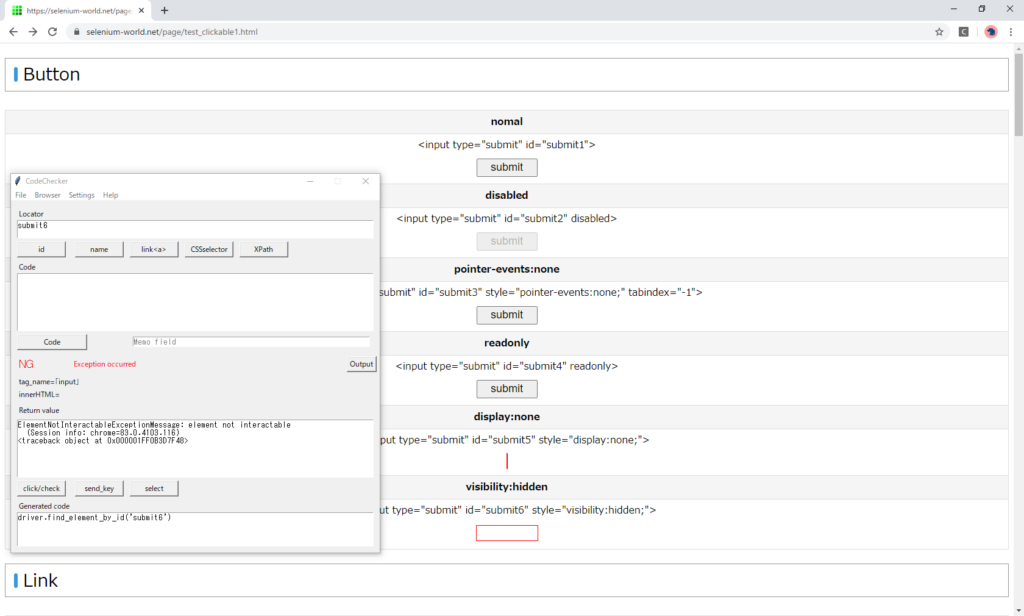
Result
Button | click | note |
---|---|---|
normal | OK | |
disabled | NG | No response |
pointer-events:none | Ex | |
readonly | OK | |
display:none | Ex | |
visibility:hidden | Ex |
Even if it is operated manually, the click event will occur in “normal" and “readonly", which matches the Selenium results.
Note
If you try to manipulate the following elements before closing the dialog, an Selenium exception occurs.
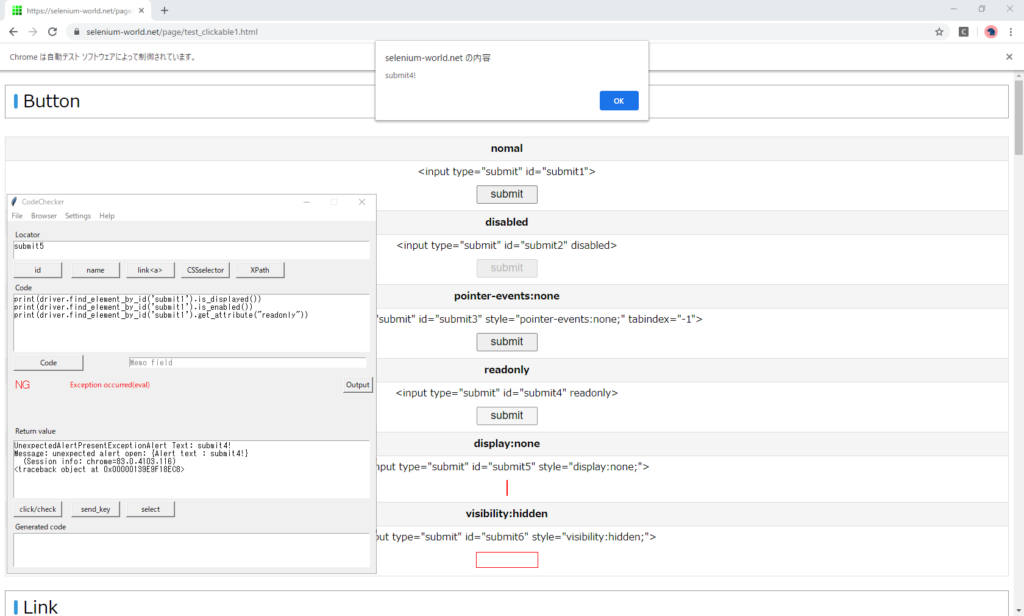
Message
UnexpectedAlertPresentExceptionAlert Text: submit4!
Message: unexpected alert open: {Alert text : submit4!}
(Session info: chrome=83.0.4103.116)
<traceback object at 0x00000139E9F18EC8>
Get Attribute
Get the visibility, the active state (enabled/disabled), and the read-only attributes of the element.
Normal
Input the following codes, and press the “Code" button.
print(driver.find_element_by_id('submit1').is_displayed())
print(driver.find_element_by_id('submit1').is_enabled())
print(driver.find_element_by_id('submit1').get_attribute("readonly"))
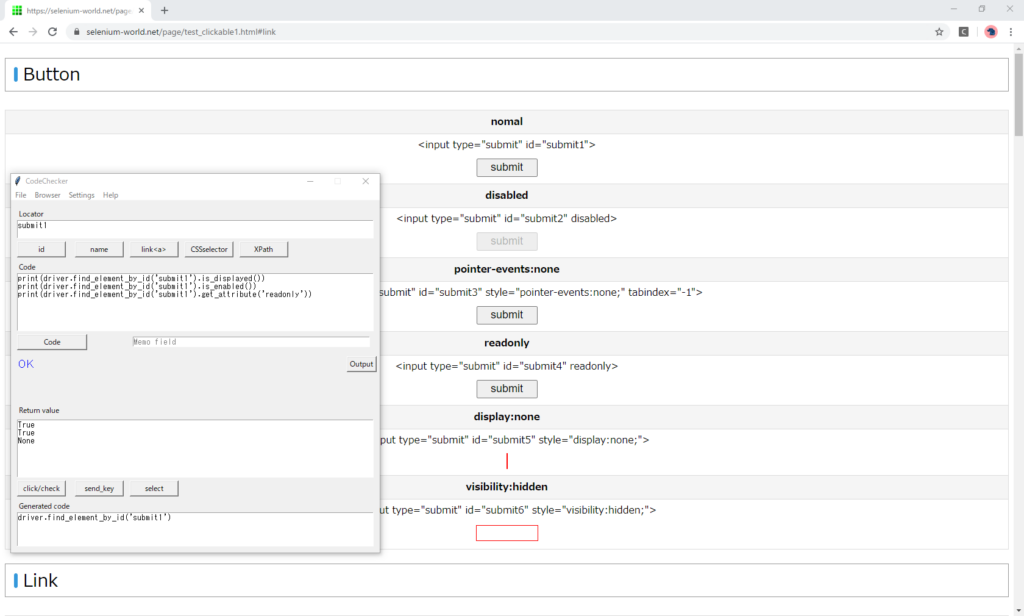
Inactive(disabled)
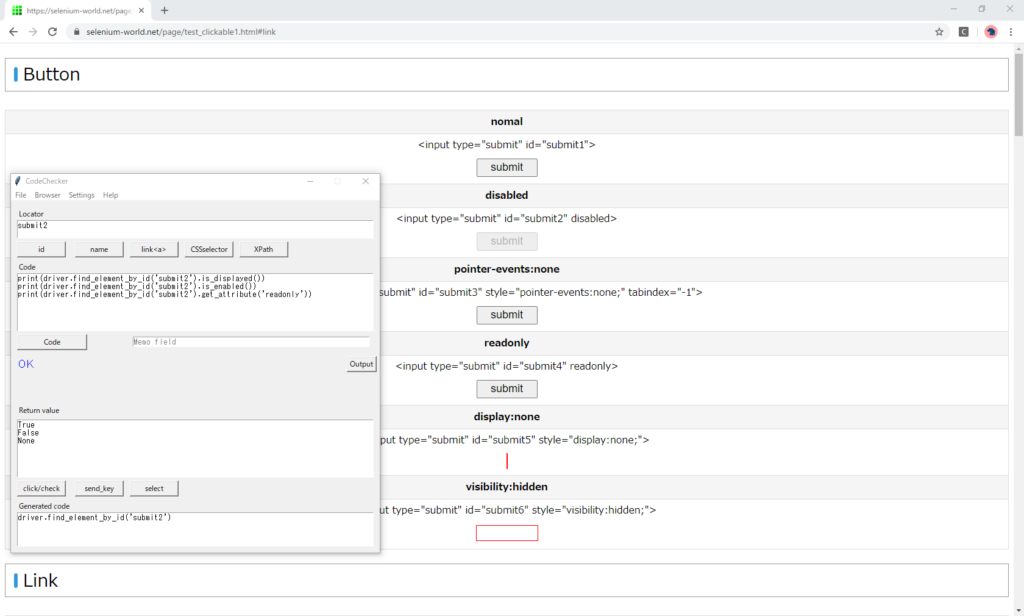
Inactive(pointer-events:none)
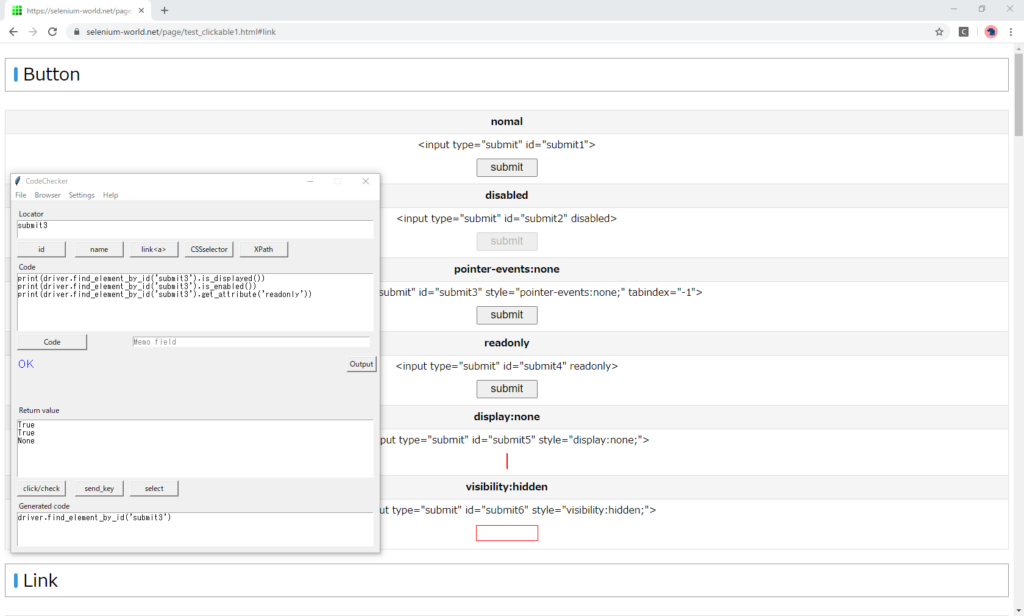
Read-only(readonly)
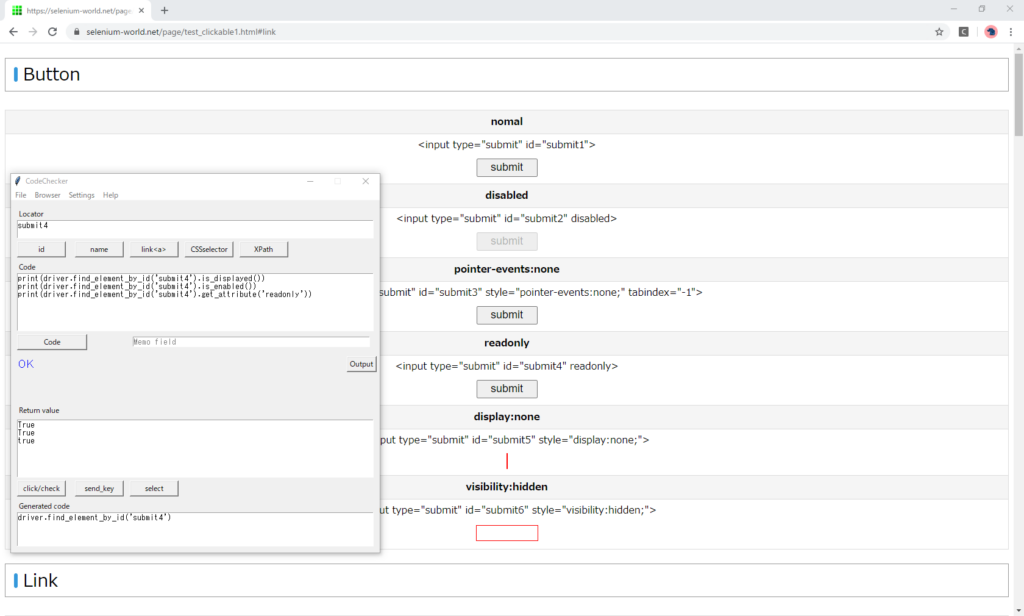
Hidden(display:none)
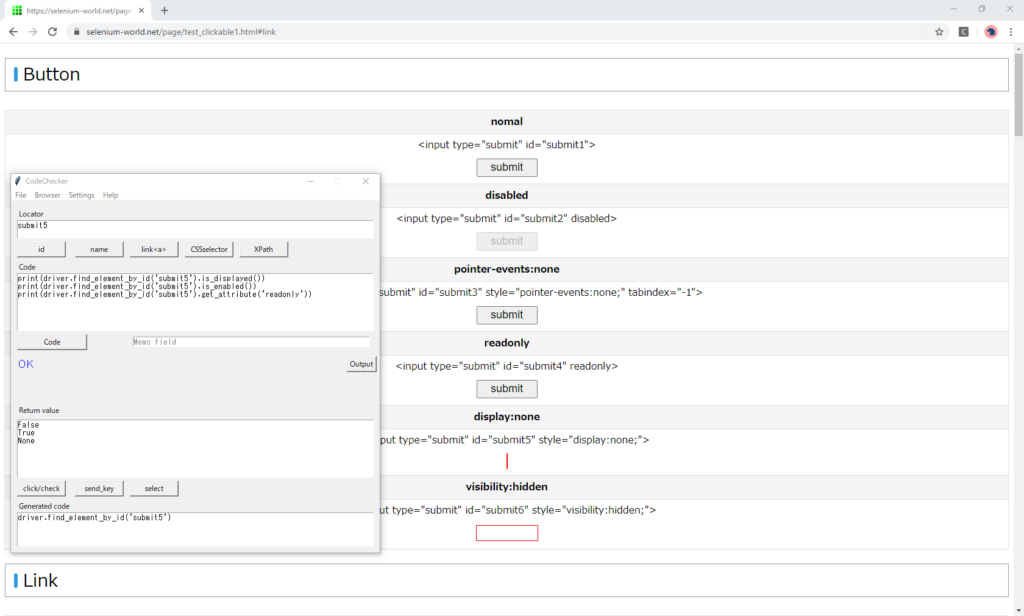
Hidden(visibility:hidden)
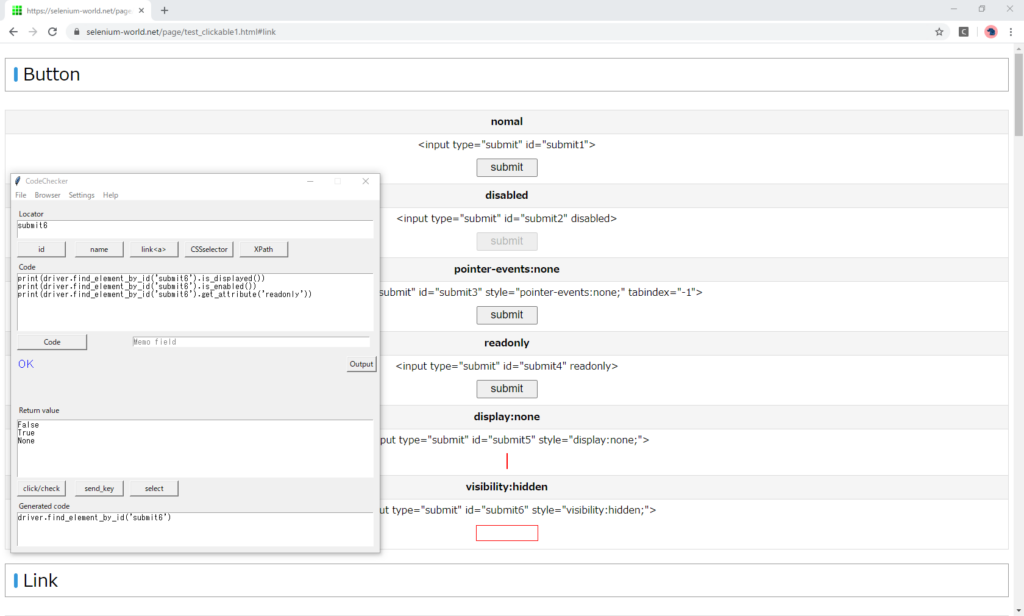
Result
Button | is_displayed() | is_enabled() | readonly |
---|---|---|---|
normal | True | True | None |
disabled | True | False | None |
pointer-events:none | True | True | None |
readonly | True | True | true |
display:none | False | True | None |
visibility:hidden | False | True | None |
- “readonly" is an HTML attribute, so the return value is “true(lowercase)" or “None".
- It is “is_displayed() =False" in “display:none" and “visibility:hidden", but the active state is “is_enabled() =True".
Link
Click
Normal
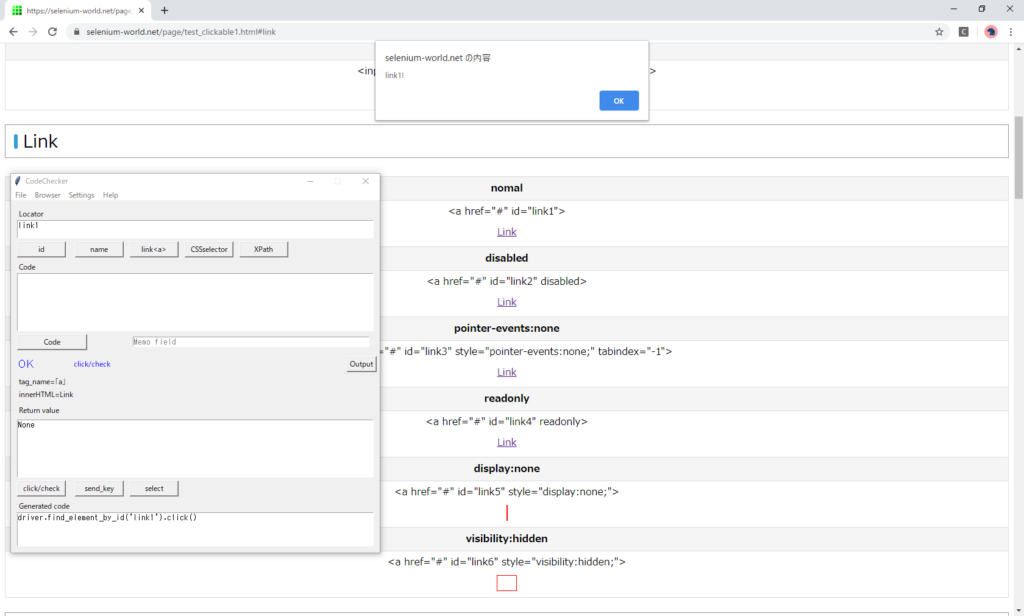
Inactive(disabled)
There is no “disabled" attribute for <a> tag. That is invalid.
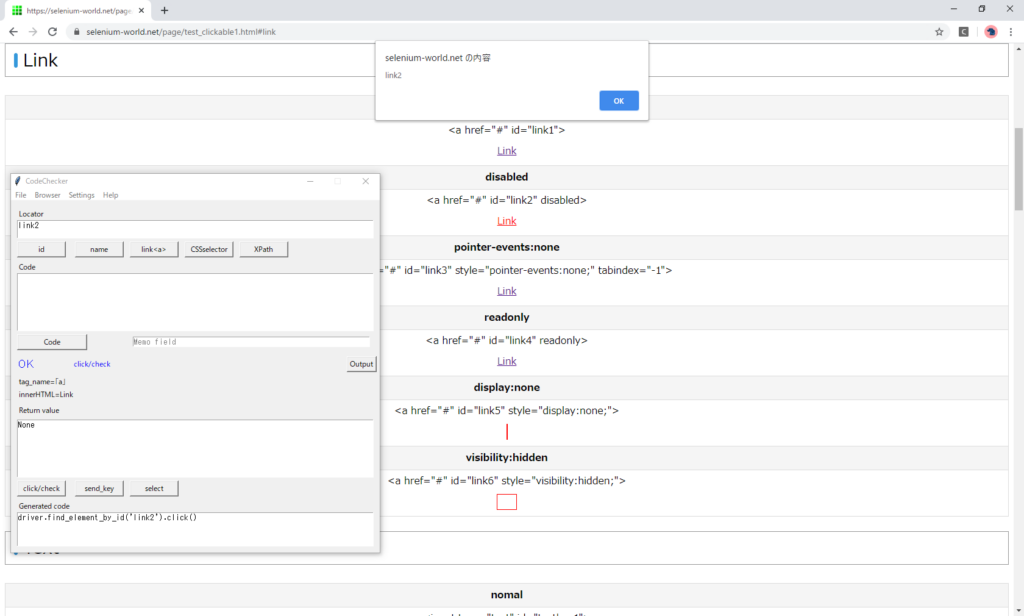
You can also click it manually.
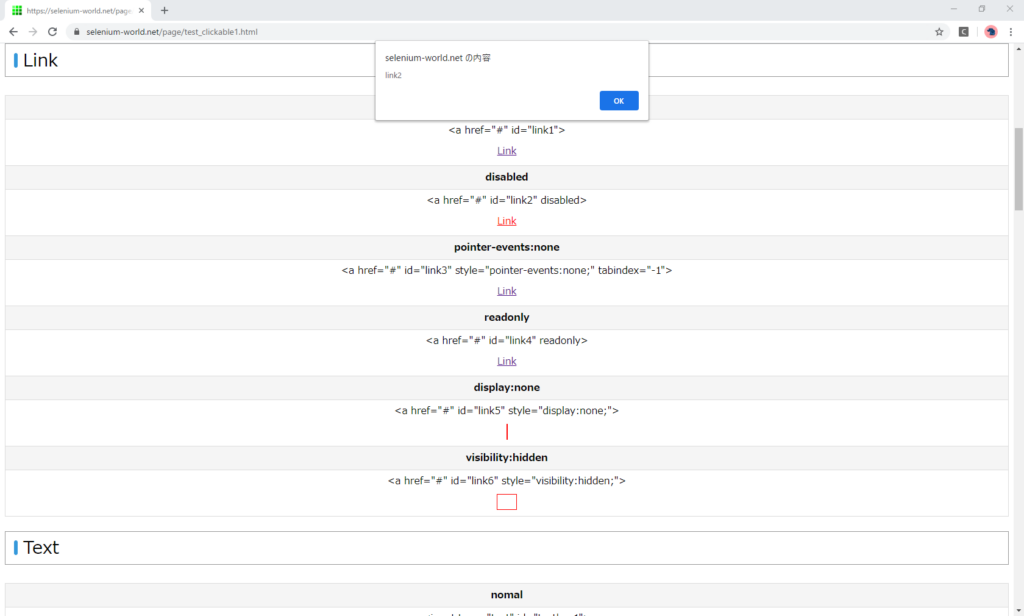
Inactive(pointer-events:none)
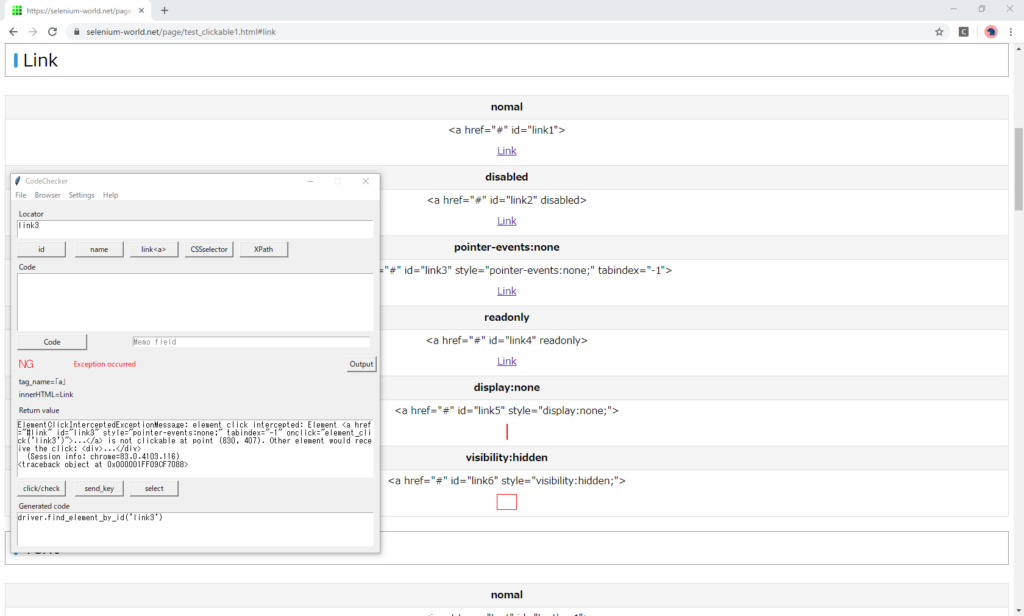
Read-only(readonly)
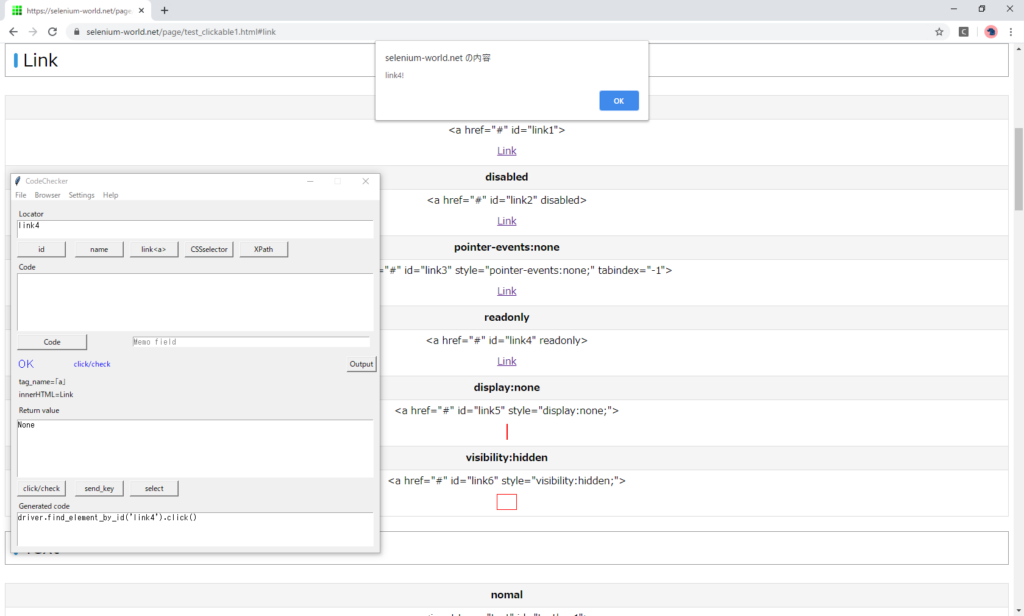
Hidden(display:none)
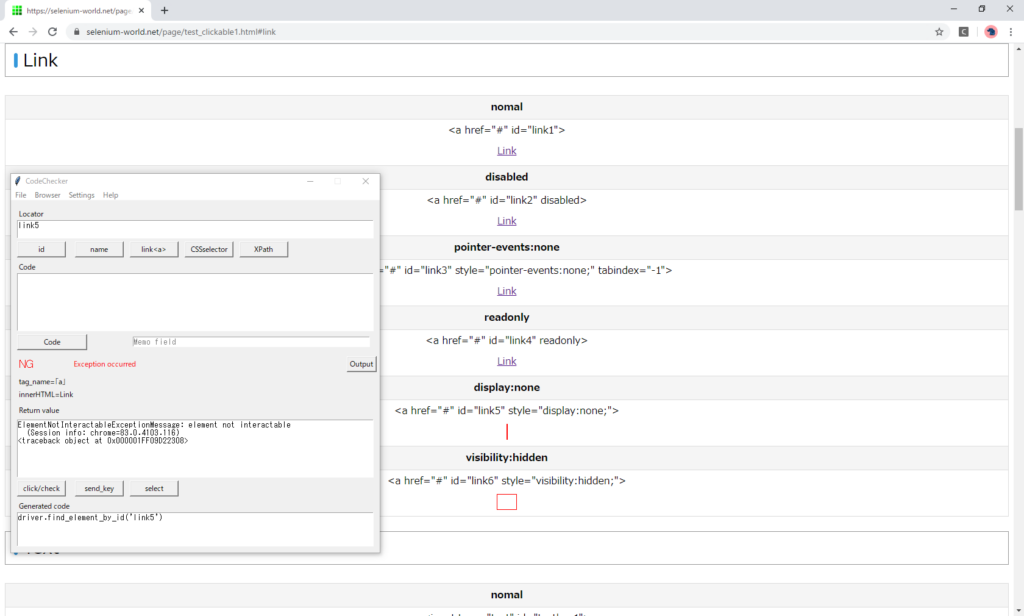
Hidden(visibility:hidden)
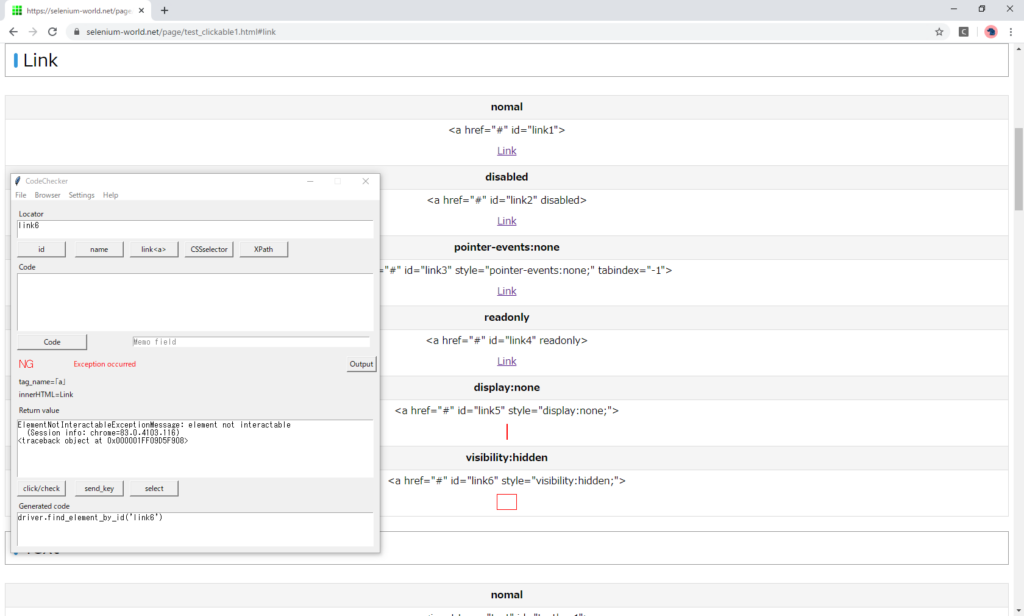
Result
Link | click | note |
---|---|---|
normal | OK | |
disabled | OK | |
pointer-events:none | Ex | |
readonly | OK | |
display:none | EX | |
visibility:hidden | Ex |
Get Attribute
Normal
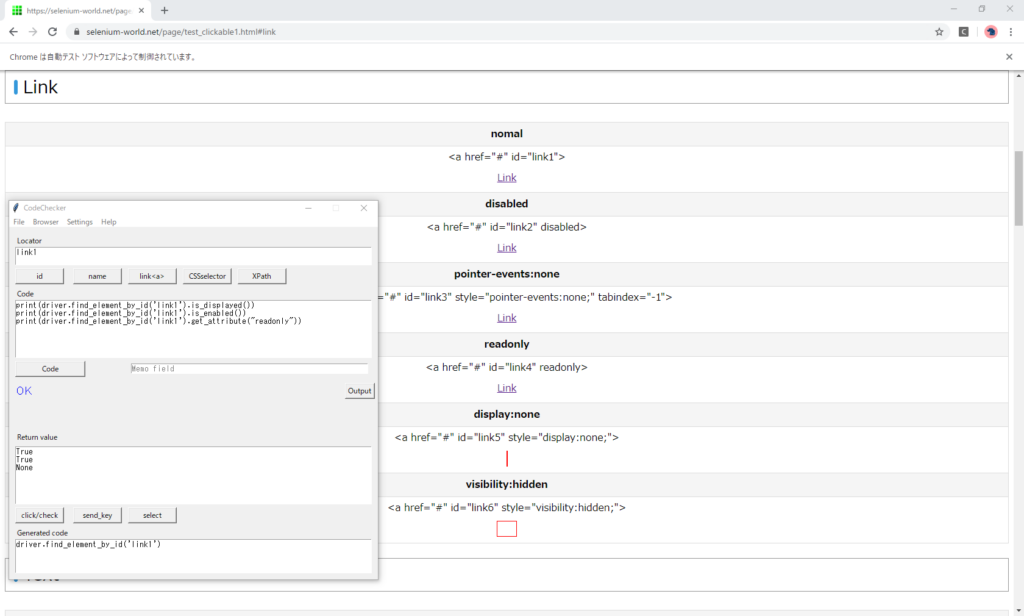
Inactive(disabled)
“is_enabled() = True": There is no “disabled" attribute for tag.
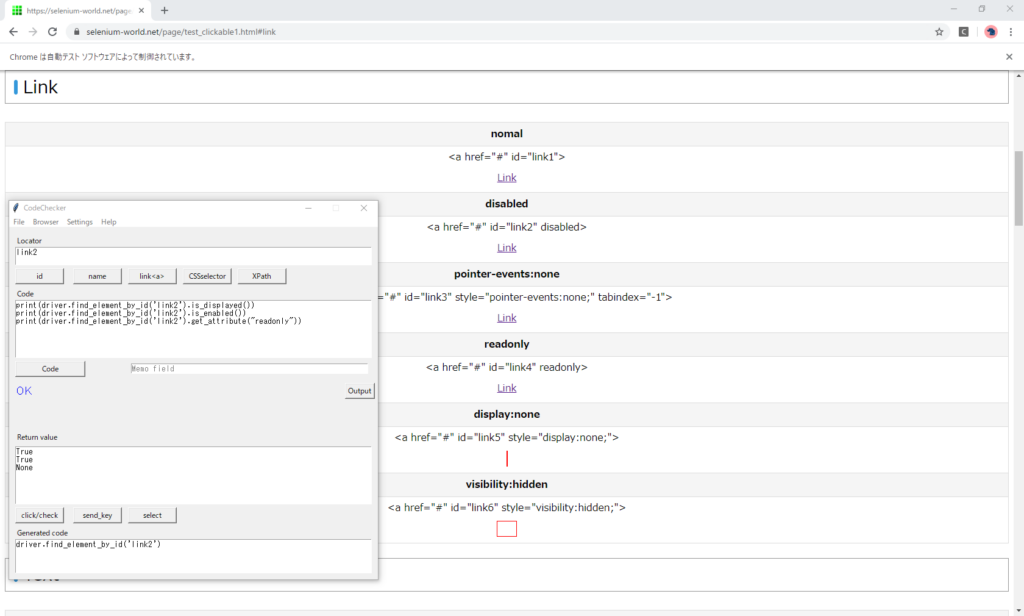
Inactive(pointer-events:none)
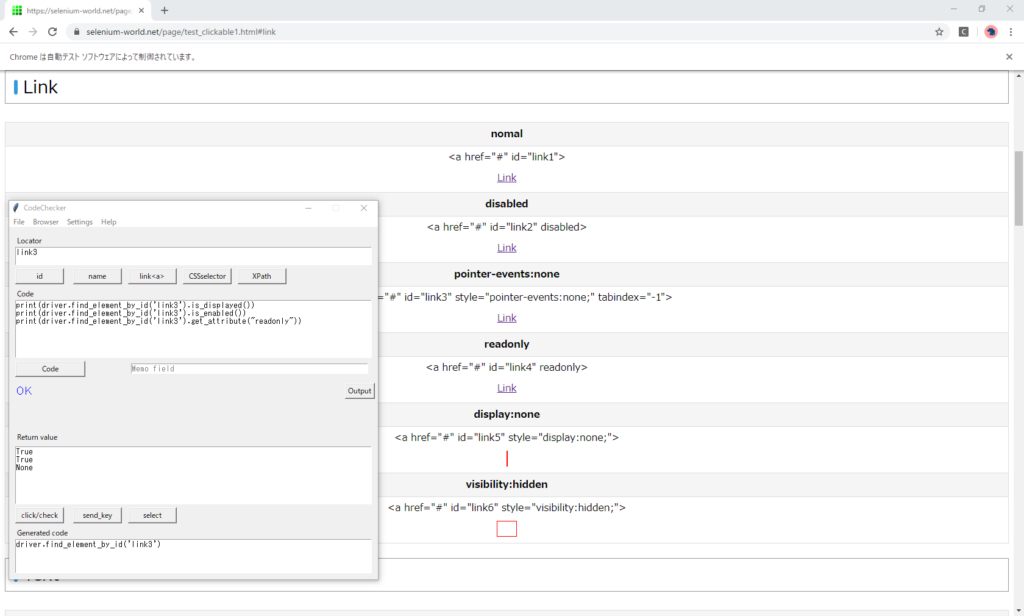
Read-only(readonly)
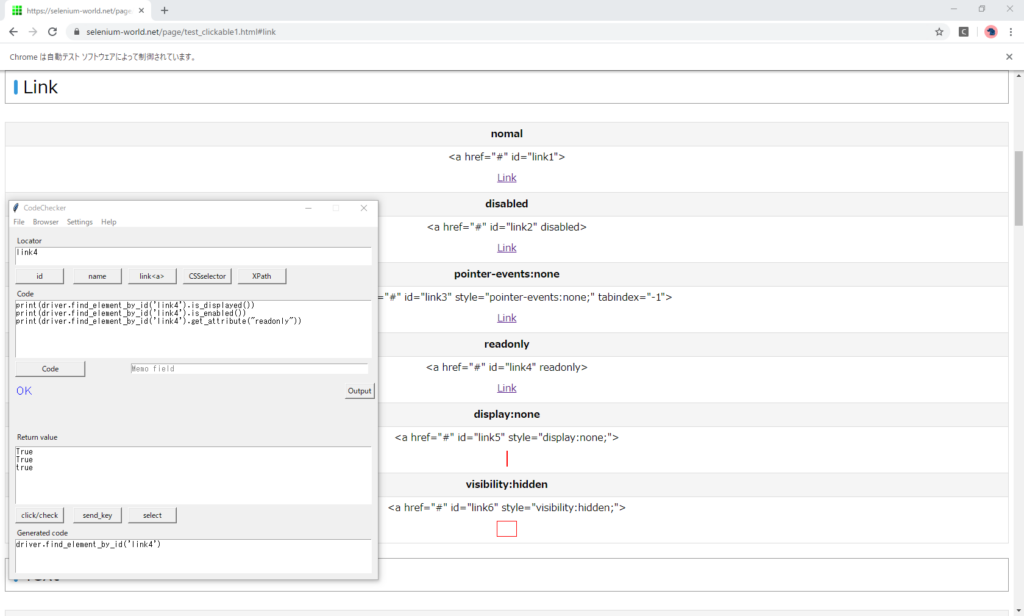
Hidden(display:none)
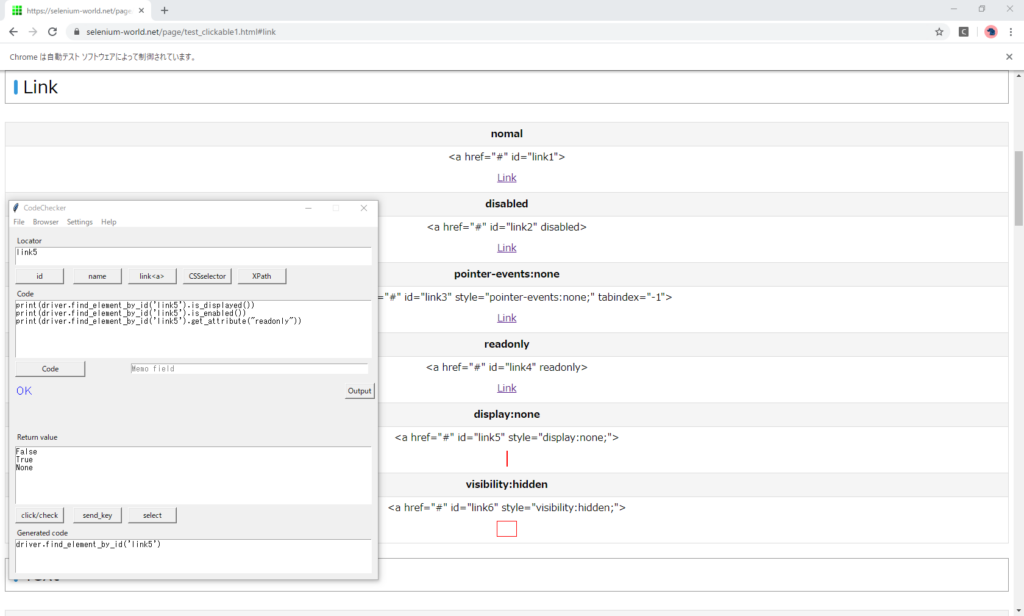
Hidden(visibility:hidden)
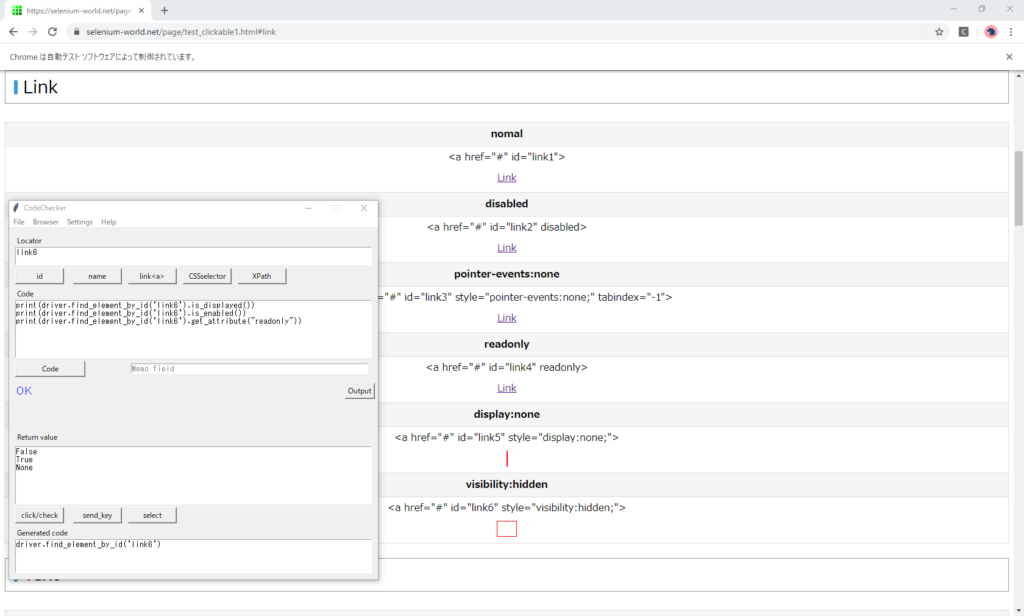
Result
Link | is_displayed() | is_enabled() | readonly |
---|---|---|---|
normal | True | True | None |
disabled | True | True | None |
pointer-events:none | True | True | None |
readonly | True | True | true |
display:none | False | True | None |
visibility:hidden | False | True | None |
Text
Send_keys
Normal
After getting the element, press the “send_key" button. “hoge" is entered.
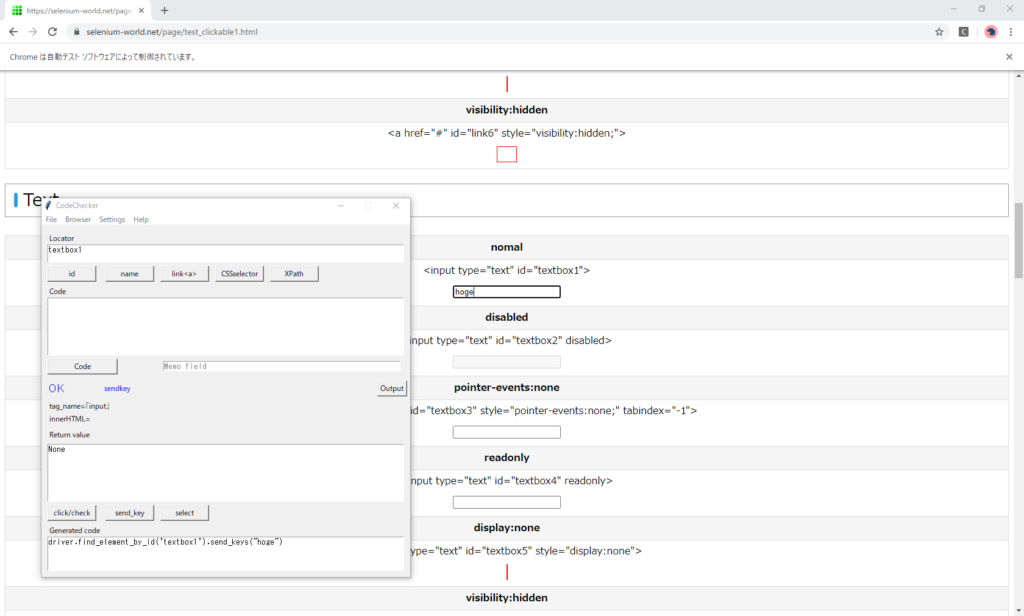
Inactive(disabled)
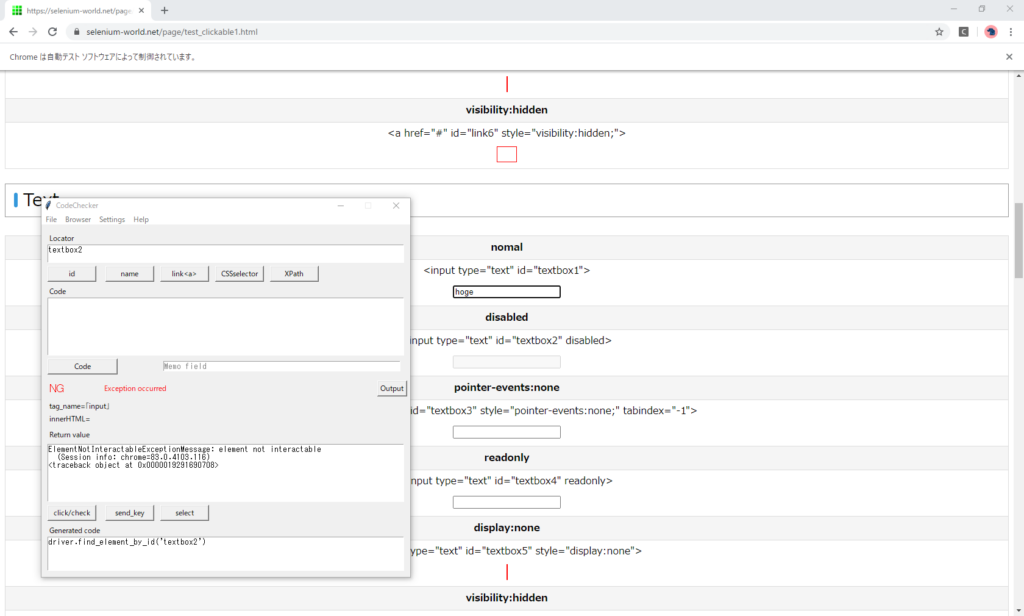
Inactive(pointer-events:none)
Enterable with selenium.
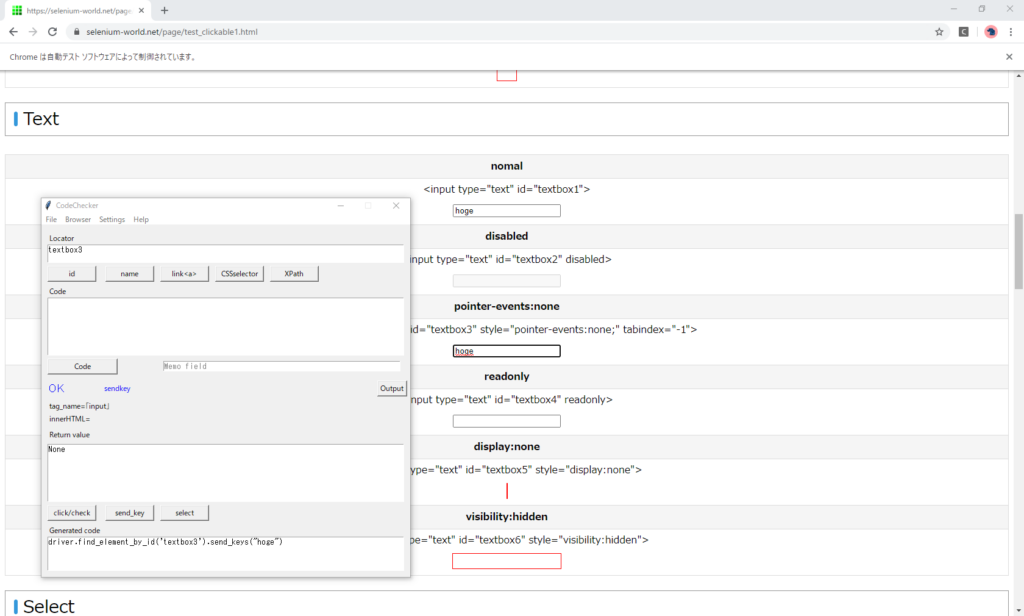
Not clickable and unable to enter due to “tabindex = -1" specification, so it is impossible to input characters with manually.
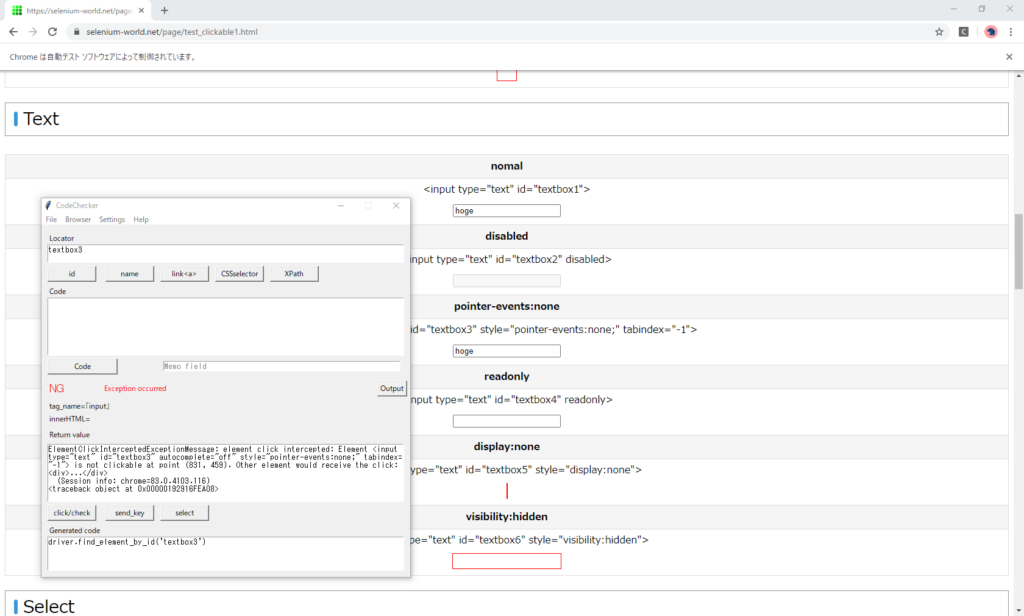
Read-only(readonly)
Nothing is entered.
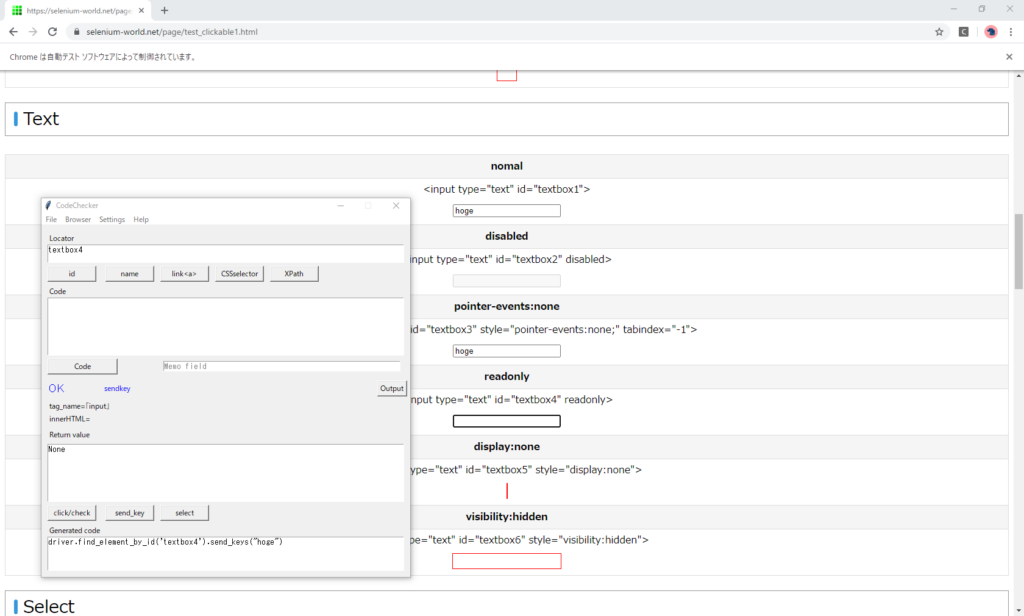
Hidden(display:none)
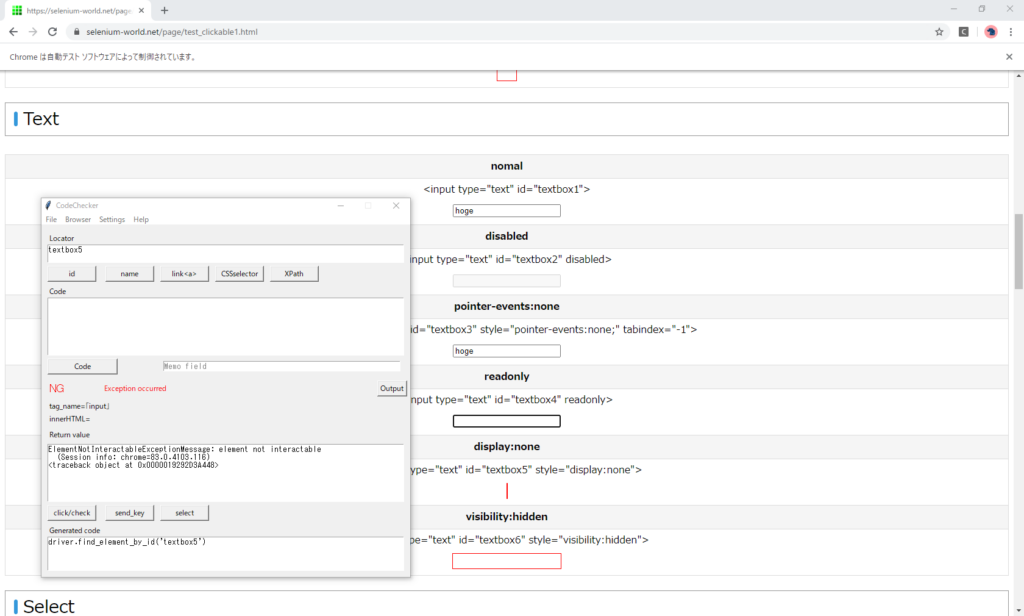
Hidden(visibility:hidden)
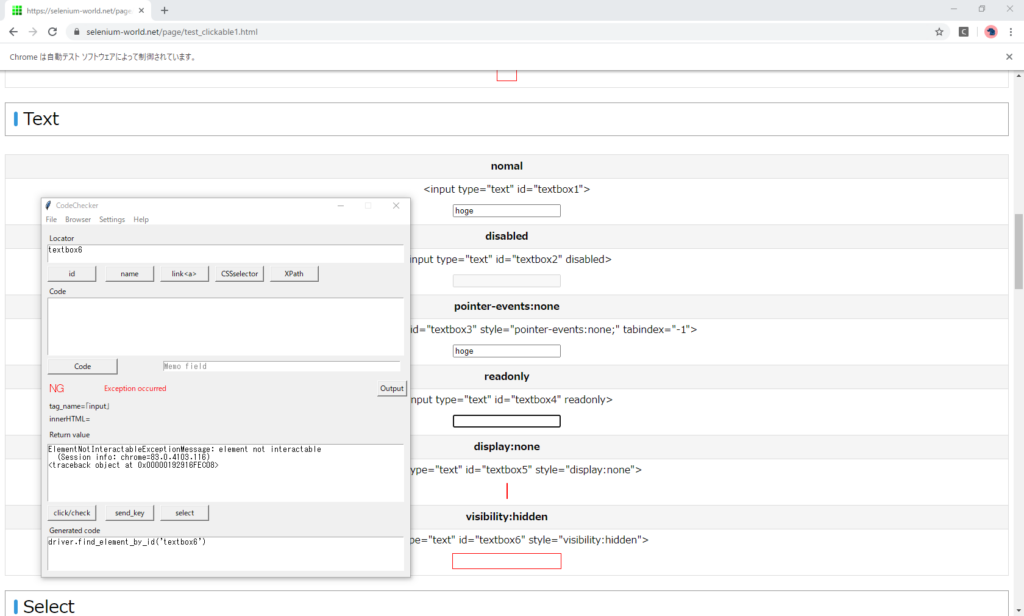
Get value
Gets the contents of the text by “get_attribute('value’)“.
Normal
“hoge" is returned.
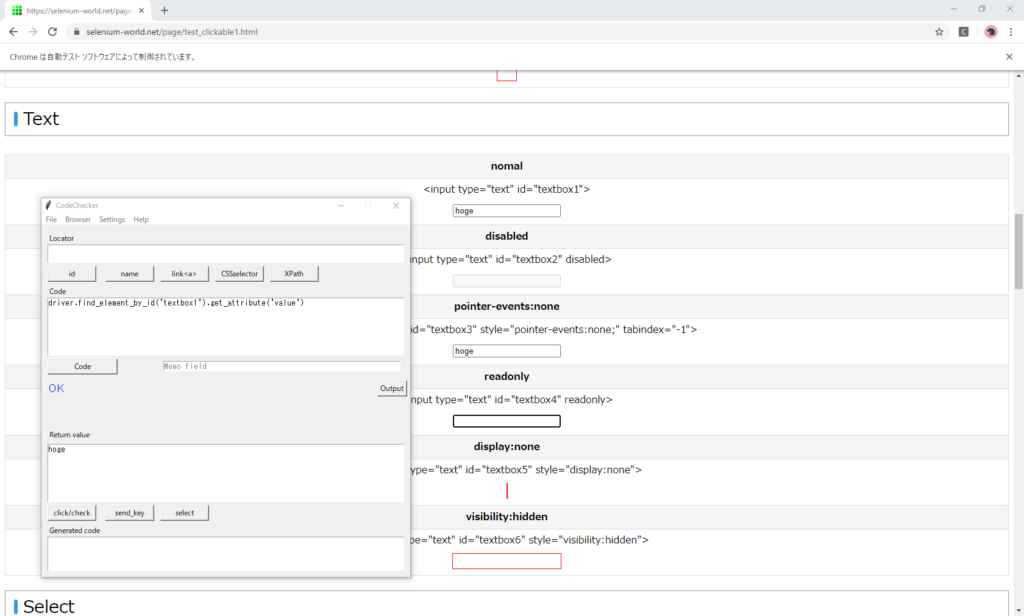
Inactive(disabled)
The input value is empty, but the value can be retrieved.
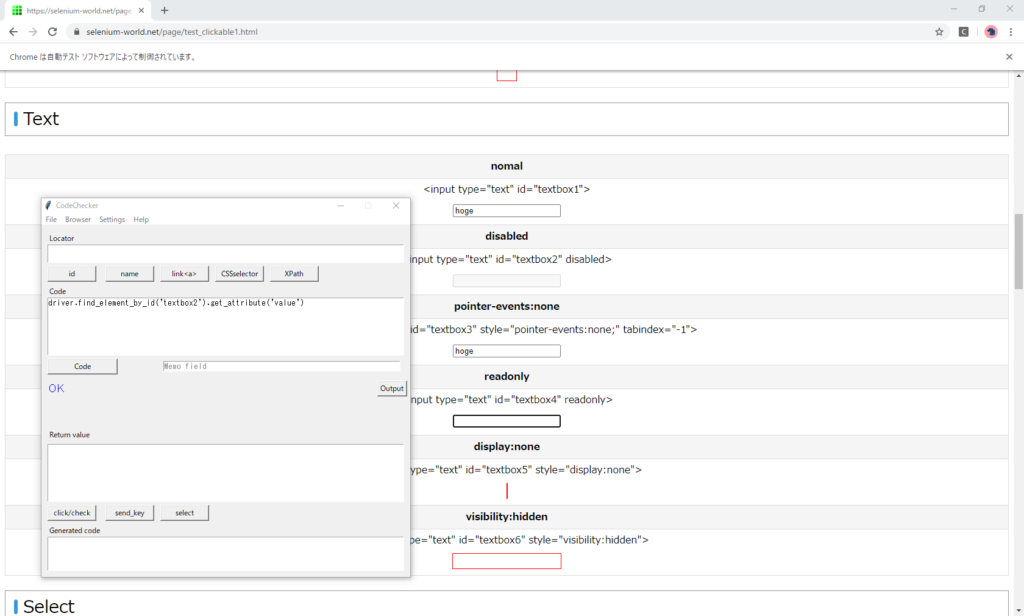
Inactive(pointer-events:none)
“hoge" is returned.
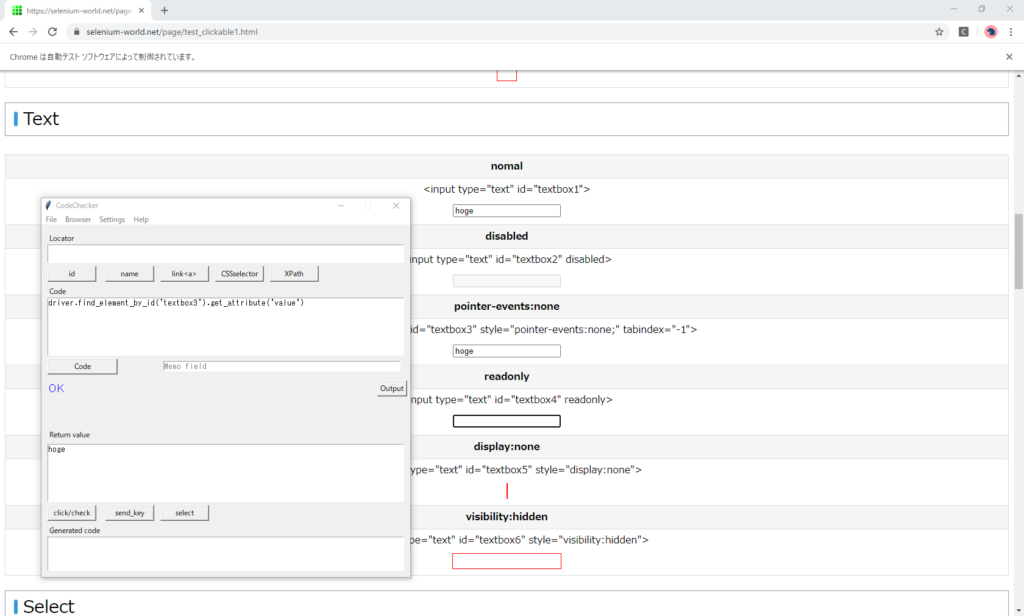
Read-only(readonly)
The input value is empty, but the value can be retrieved.
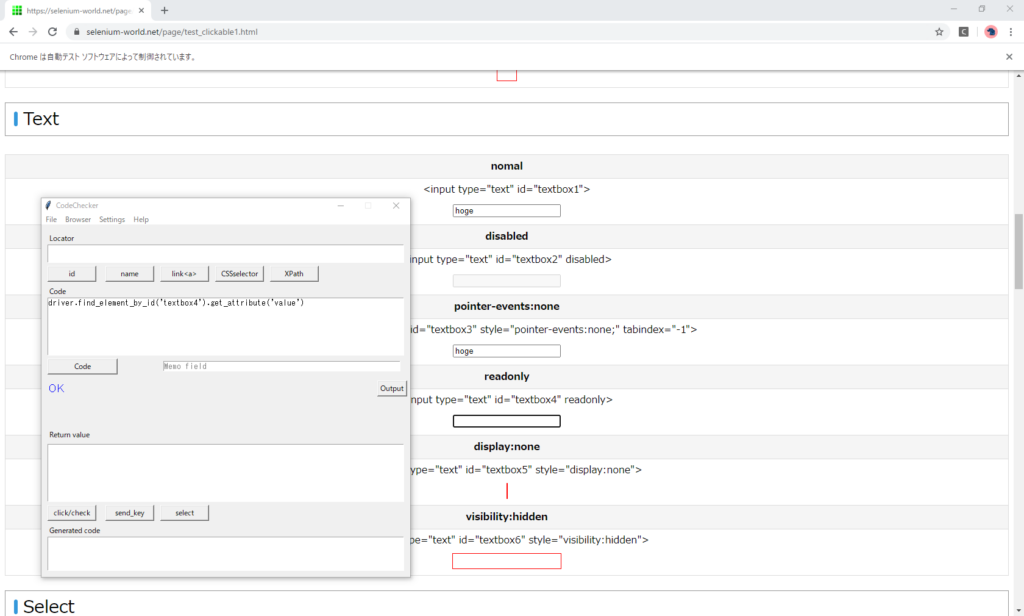
Hidden(display:none)
The input value is empty, but the value can be retrieved.
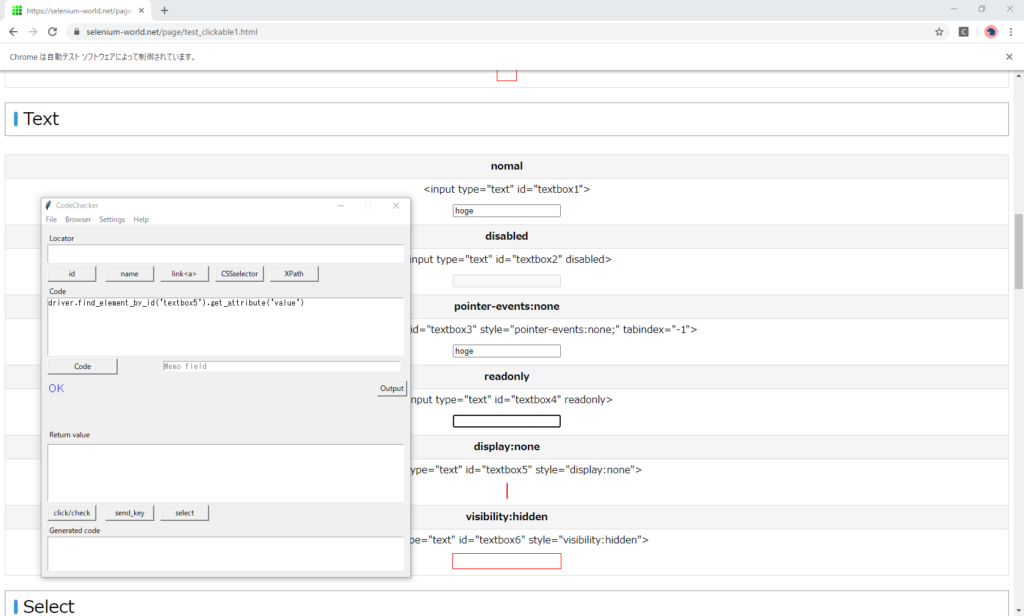
Hidden(visibility:hidden)
The input value is empty, but the value can be retrieved.
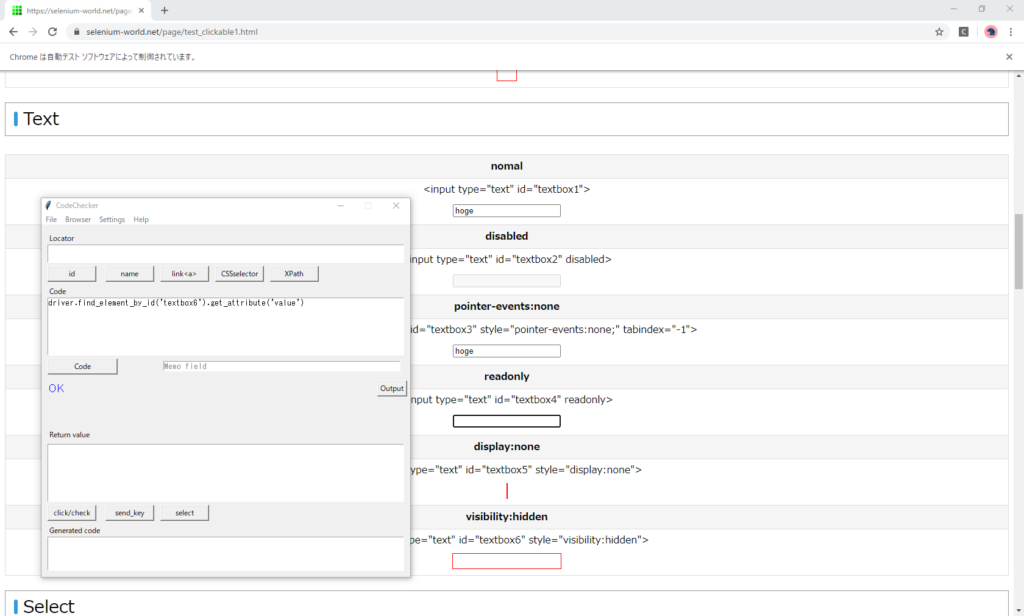
Result
Text | send_keys | get value | note |
---|---|---|---|
normal | OK | OK | |
disabled | Ex | OK | |
pointer-events:none | OK | OK | Not enterable manually |
readonly | NG | OK | |
display:none | Ex | OK | |
visibility:hidden | Ex | OK |
Get Attribute
Normal
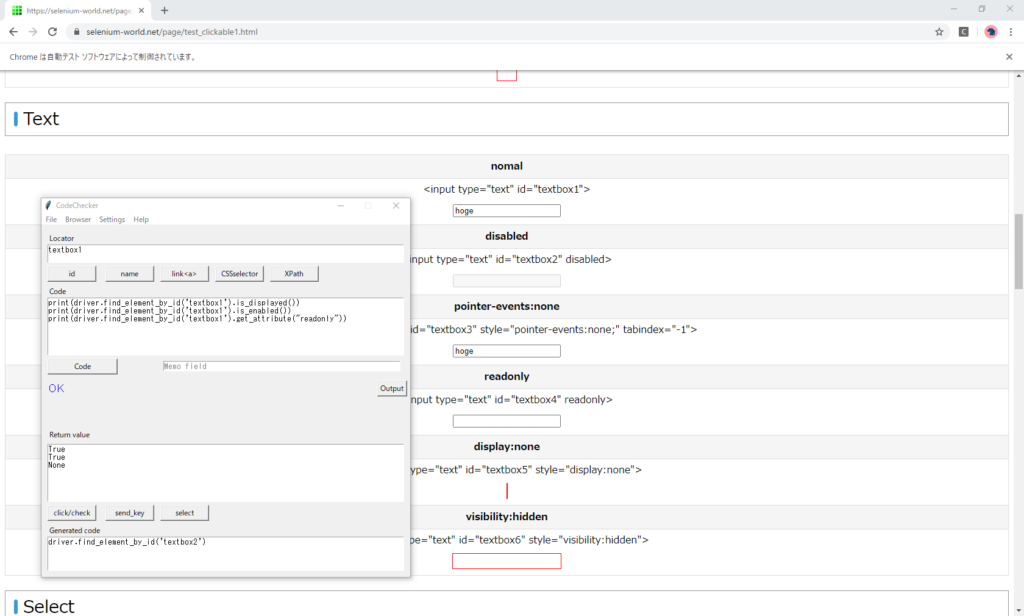
Inactive(disabled)
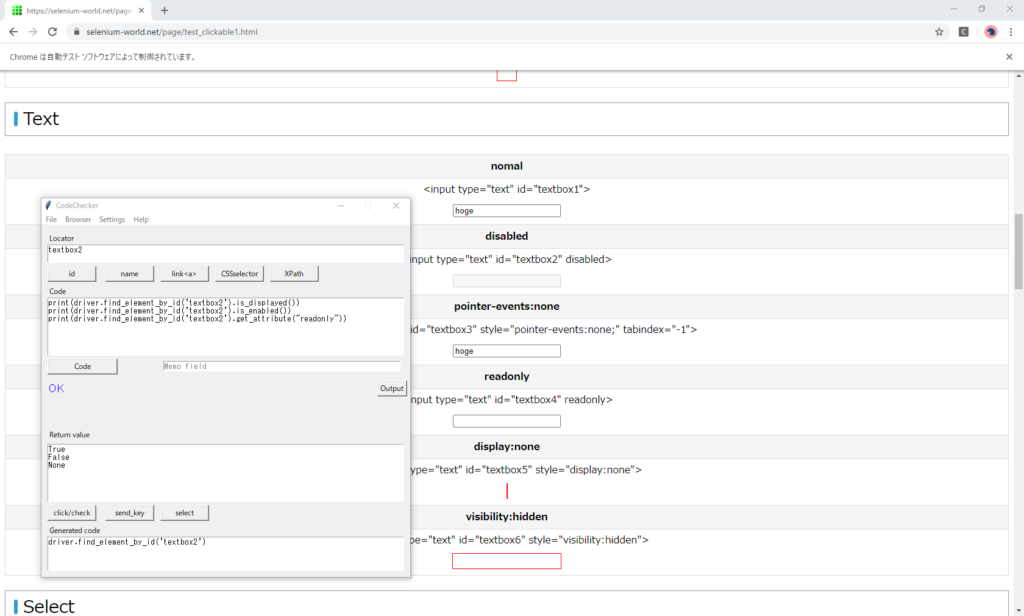
Inactive(pointer-events:none)
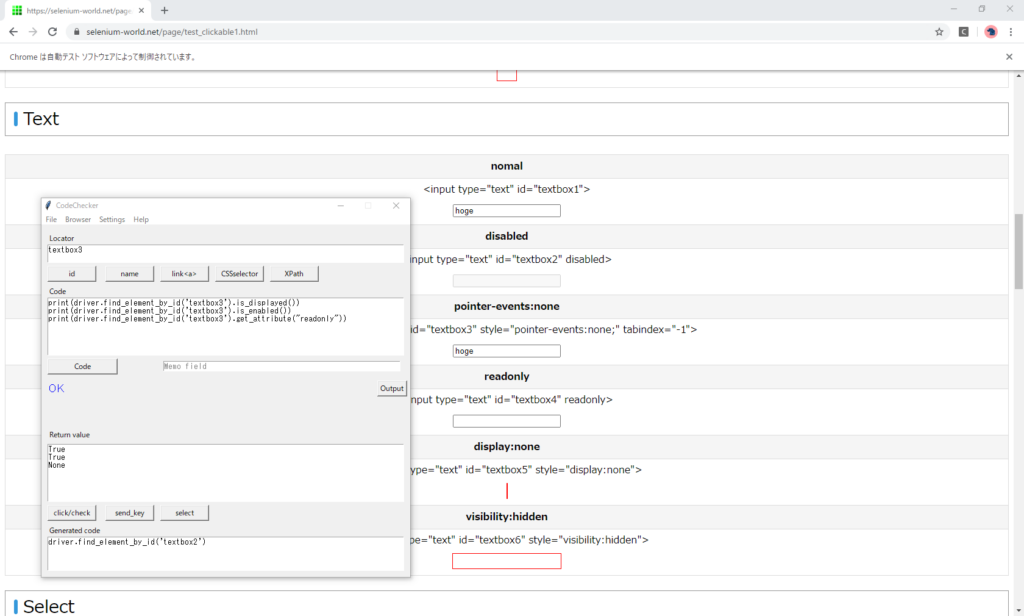
Read-only(readonly)
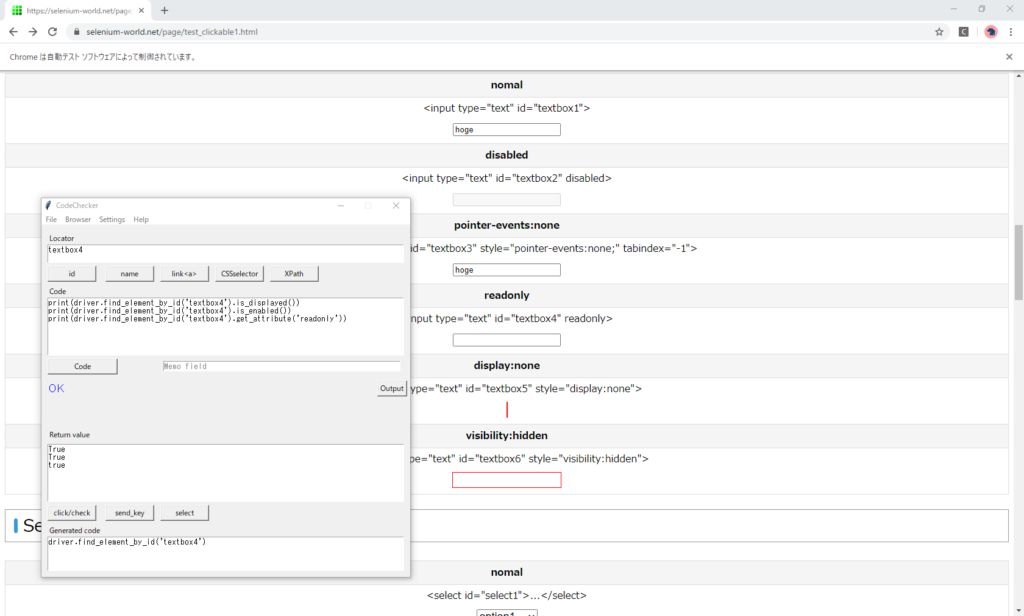
Hidden(display:none)
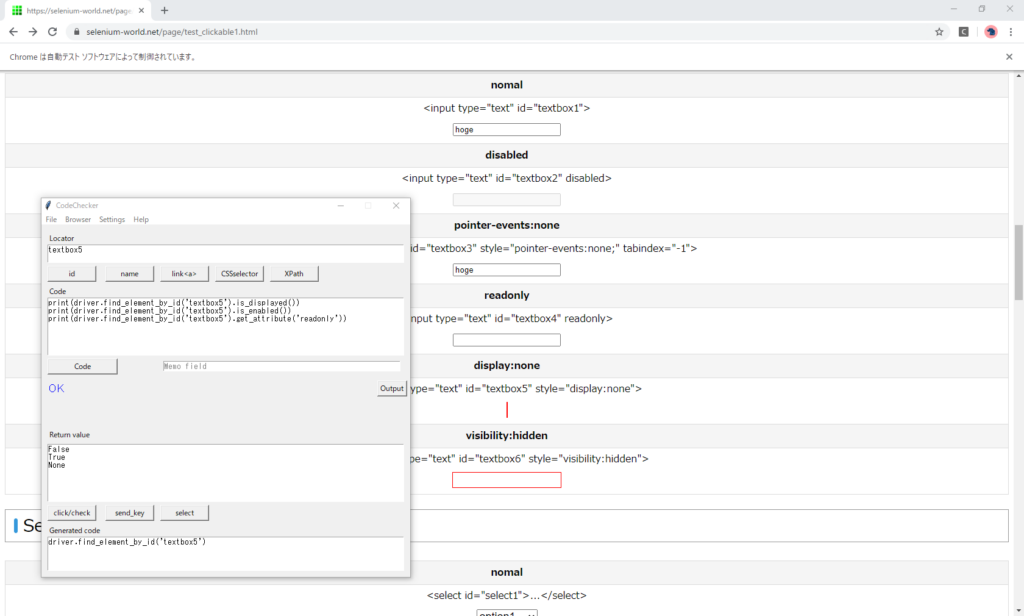
Hidden(visibility:hidden)
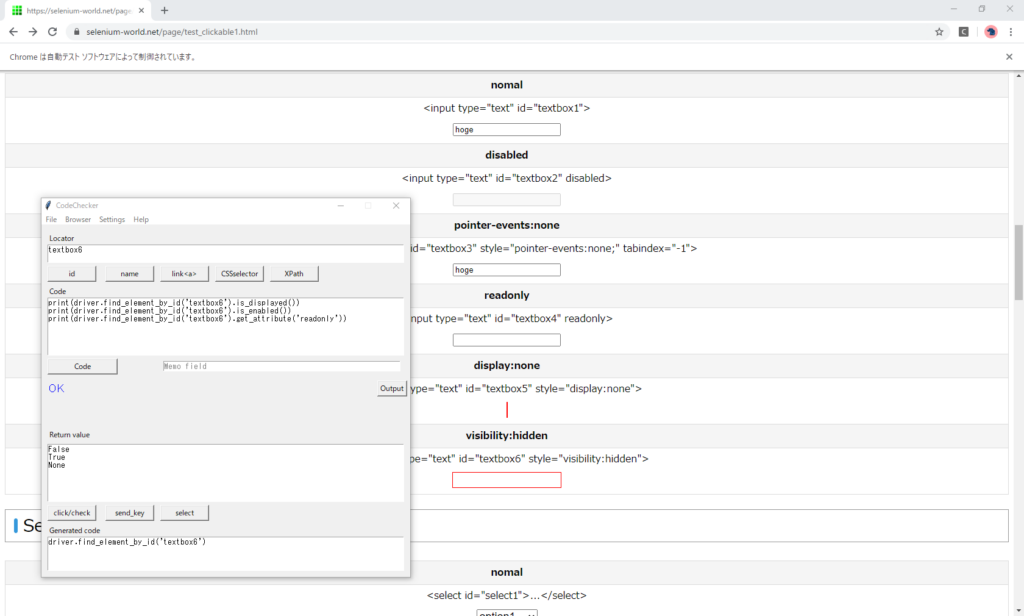
Result
Text | is_displayed() | is_enabled() | readonly |
---|---|---|---|
normal | True | True | None |
disabled | True | False | None |
pointer-events:none | True | True | None |
readonly | True | True | true |
display:none | False | True | None |
visibility:hidden | False | True | None |
Select
Select item
Normal
After getting the element, press the “select" button. The second item is selected.
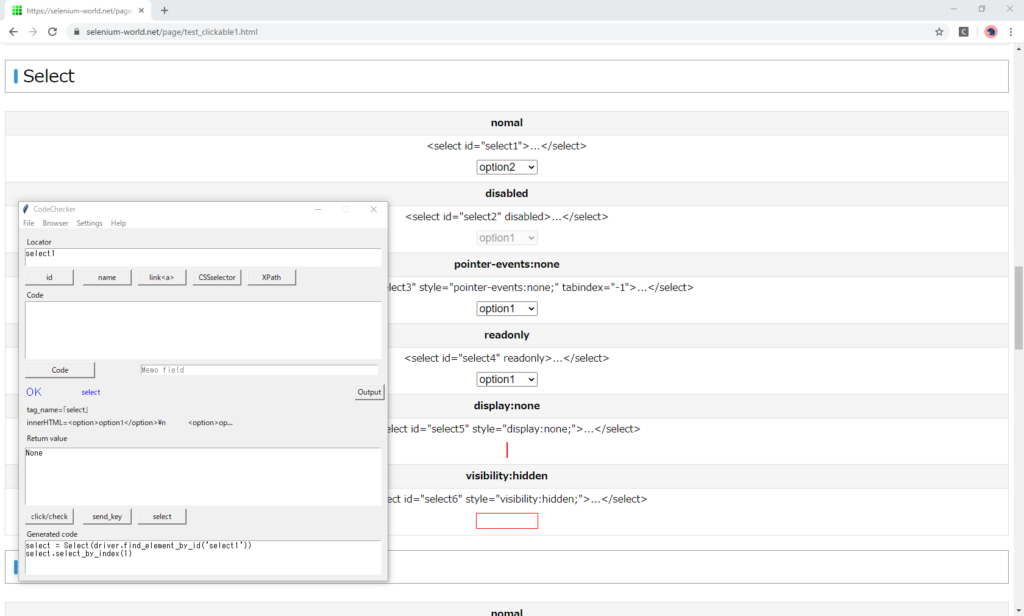
Inactive(disabled)
Not selectable.
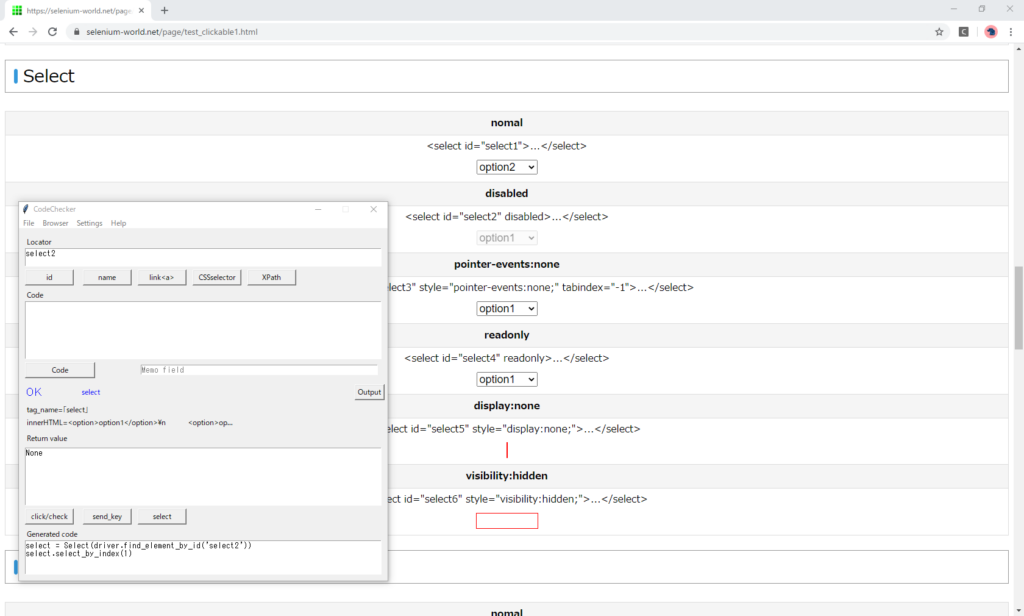
Inactive(pointer-events:none)
Not selectable.
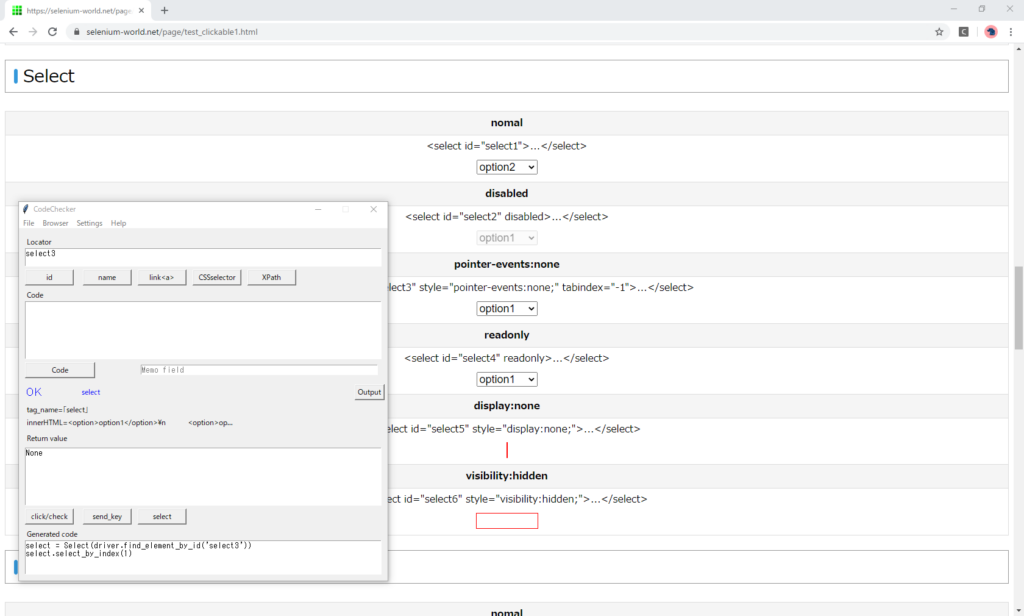
Read-only(readonly)
Selectable
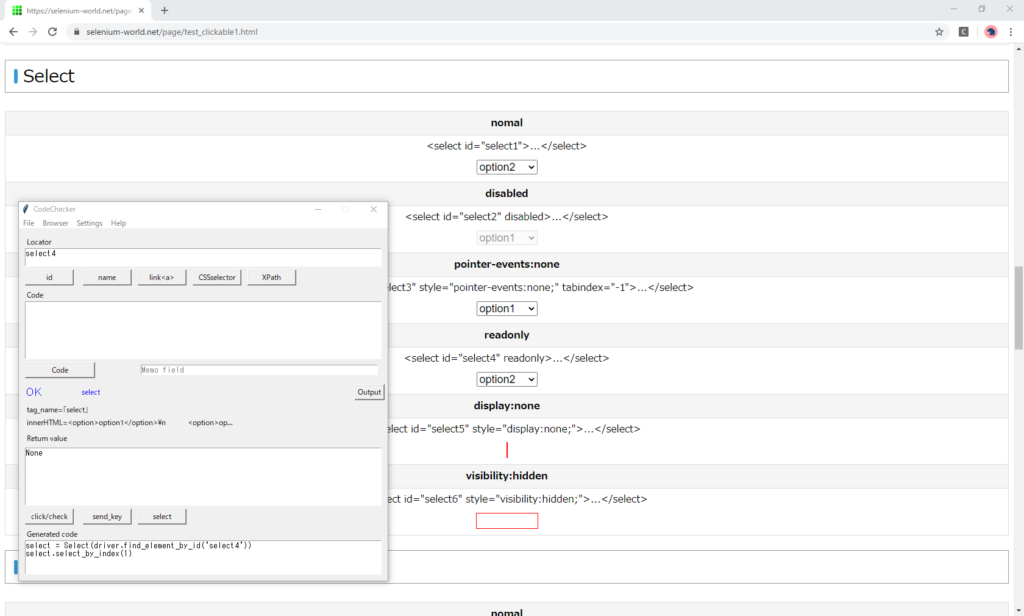
Hidden(display:none)
Exception occurred
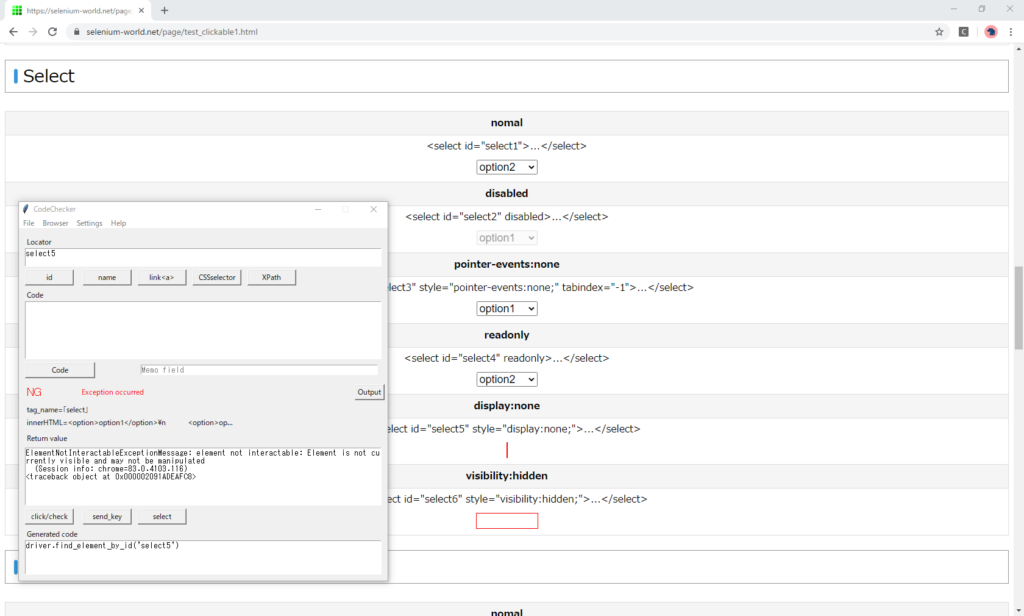
ElementNotInteractableExceptionMessage: element not interactable: Element is not currently visible and may not be manipulated
(Session info: chrome=83.0.4103.116)
<traceback object at 0x00000150CF496E08>
Hidden(visibility:hidden)
Exception occurred
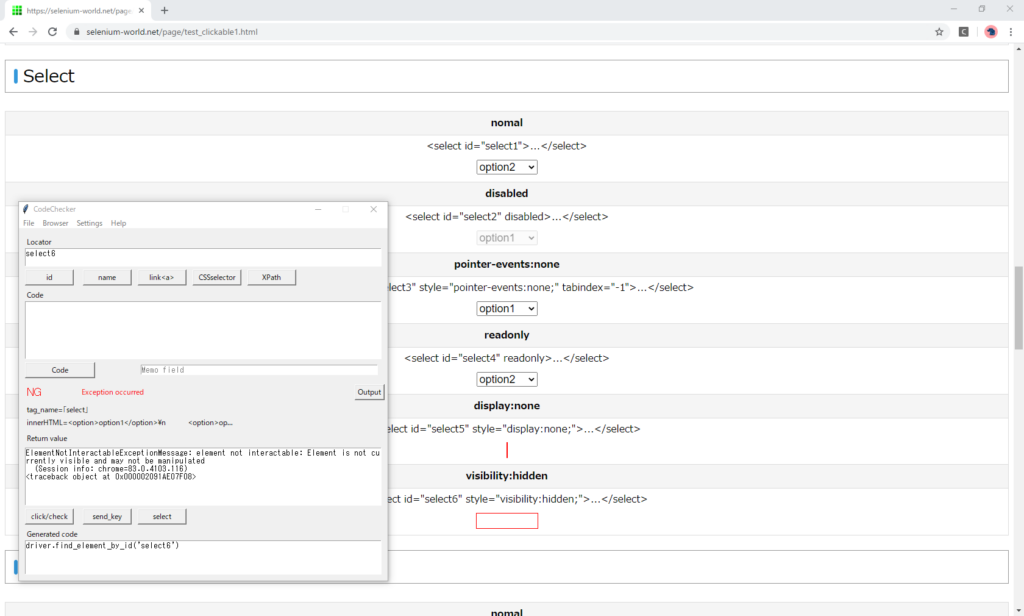
Get selected item
Normal
Input the following codes, and press the “Code" button.
select = Select(driver.find_element_by_id('select1'))
option = select.first_selected_option
print(option.text)
“option2" is displayed.
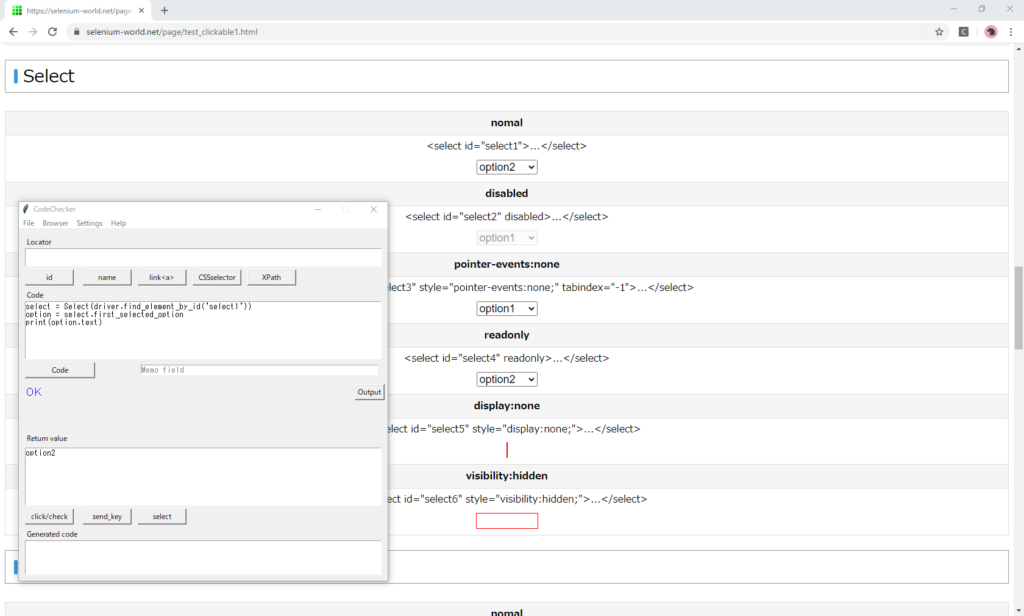
Inactive(disabled)
“option1" is displayed.
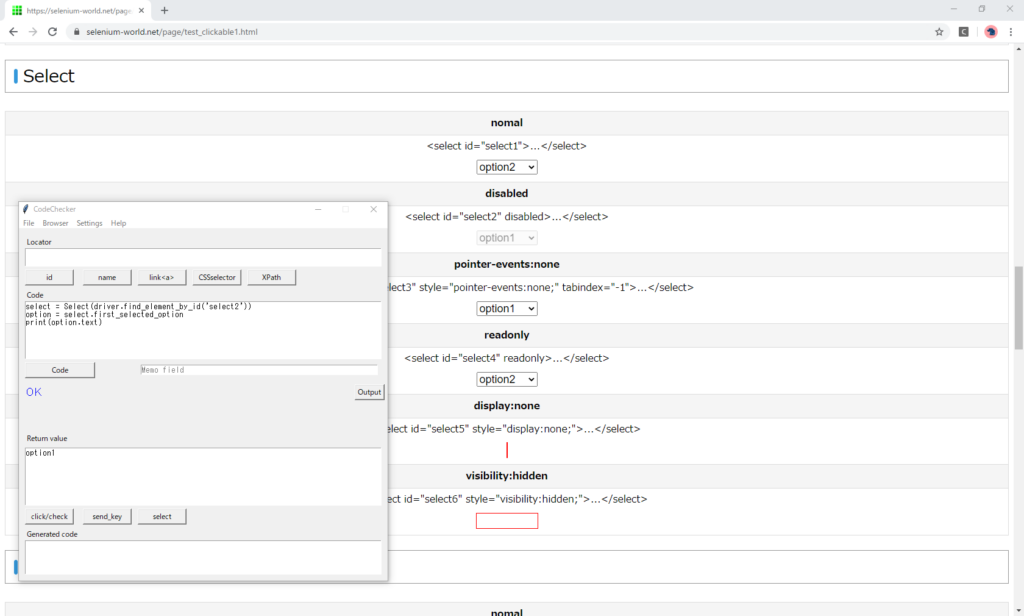
Inactive(pointer-events:none)
“option1" is displayed.
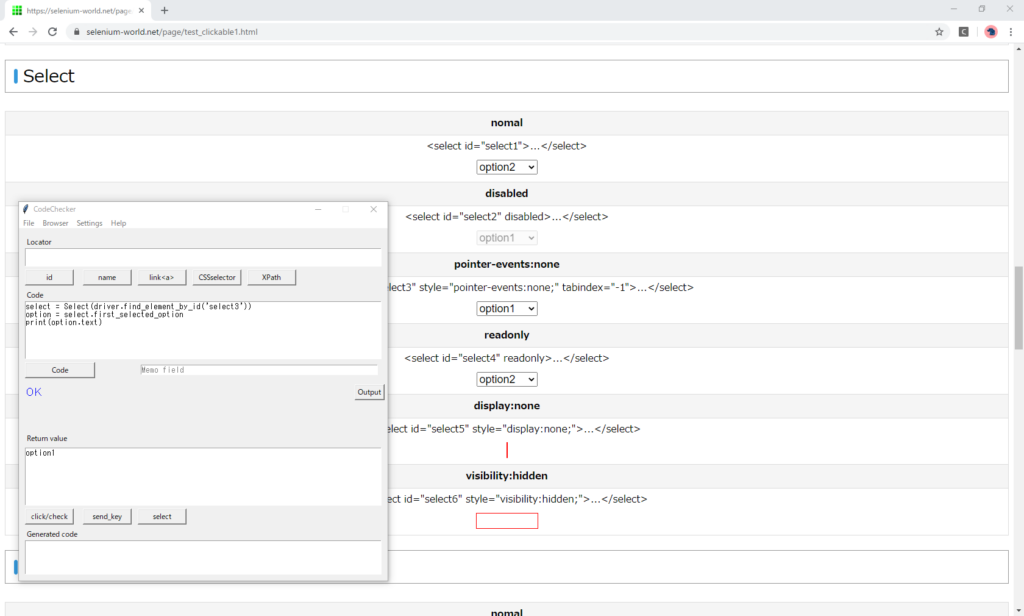
Read-only(readonly)
“option2" is displayed.
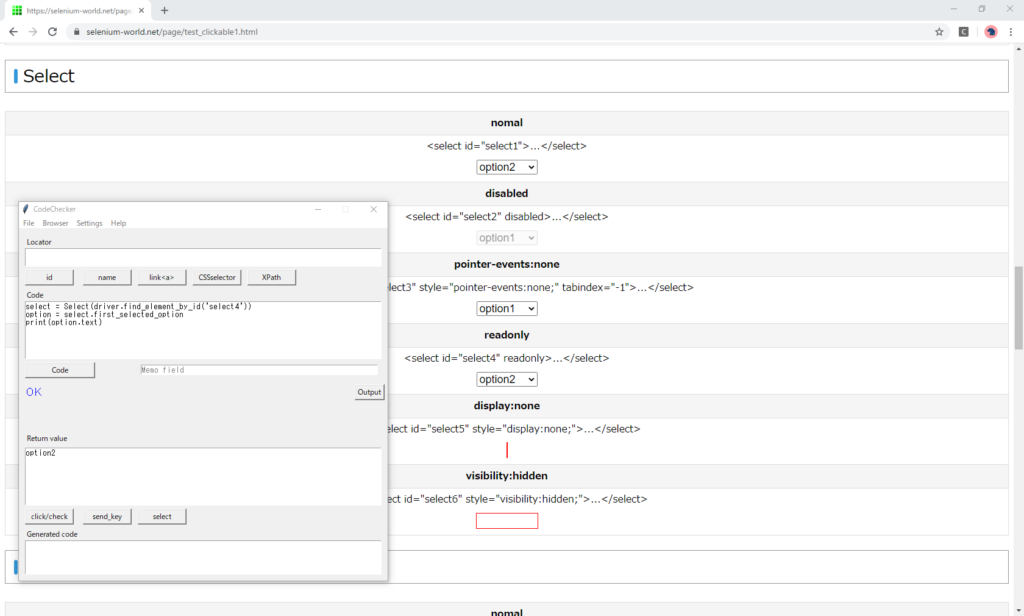
Hidden(display:none)
An empty character is returned.
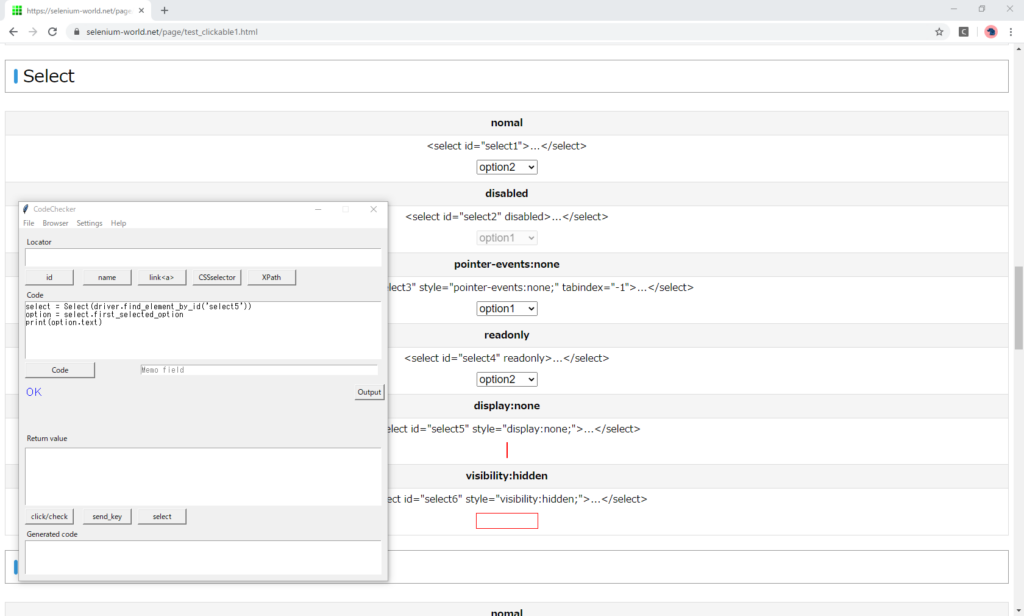
The item specified with <option> becomes an empty character.
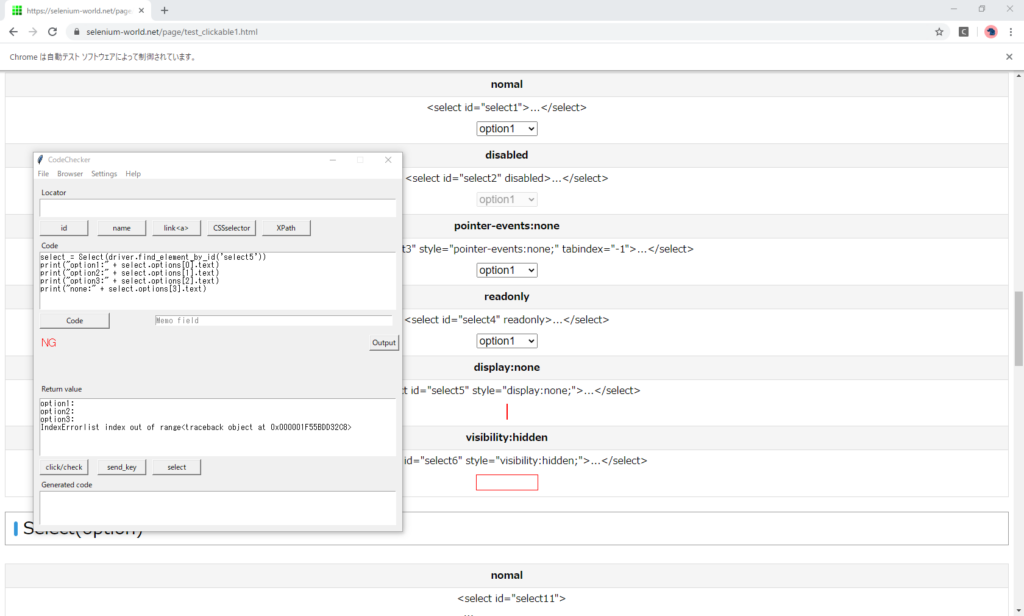
Hidden(visibility:hidden)
An empty character is returned.
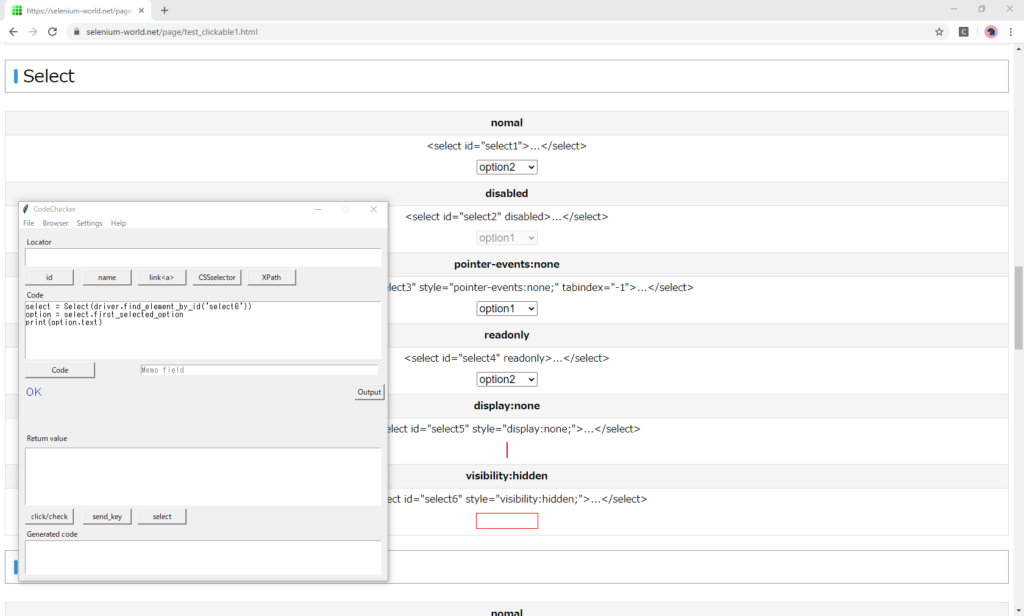
Result
Select | Select item | get Selected item | note |
---|---|---|---|
normal | OK | OK | |
disabled | NG | OK | |
pointer-events:none | NG | OK | |
readonly | OK | OK | |
display:none | Ex | NG* | *Empty is returned |
visibility:hidden | Ex | NG* | *Empty is returned |
Get Attribute
Normal
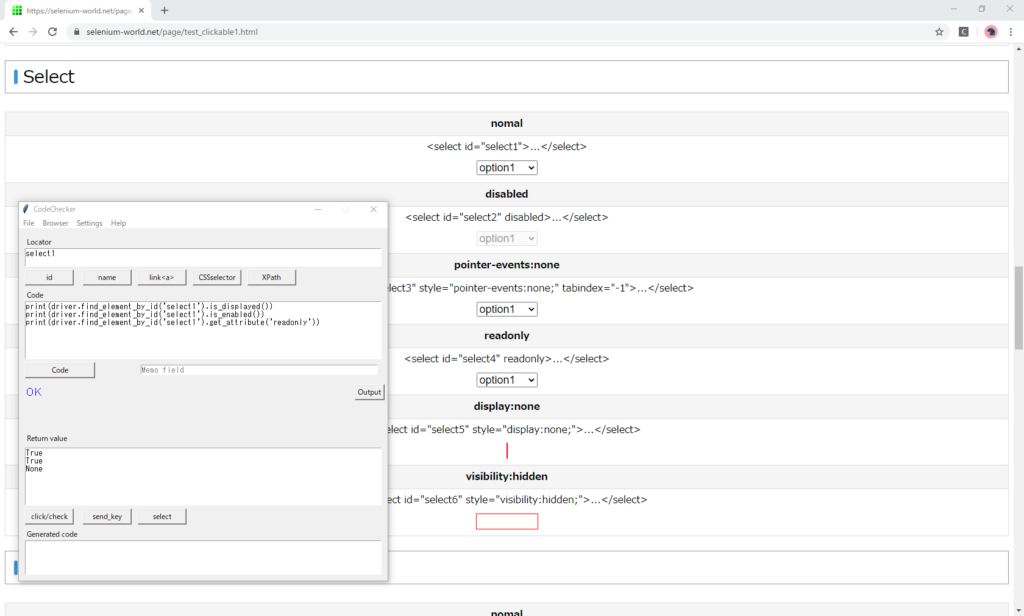
Inactive(disabled)
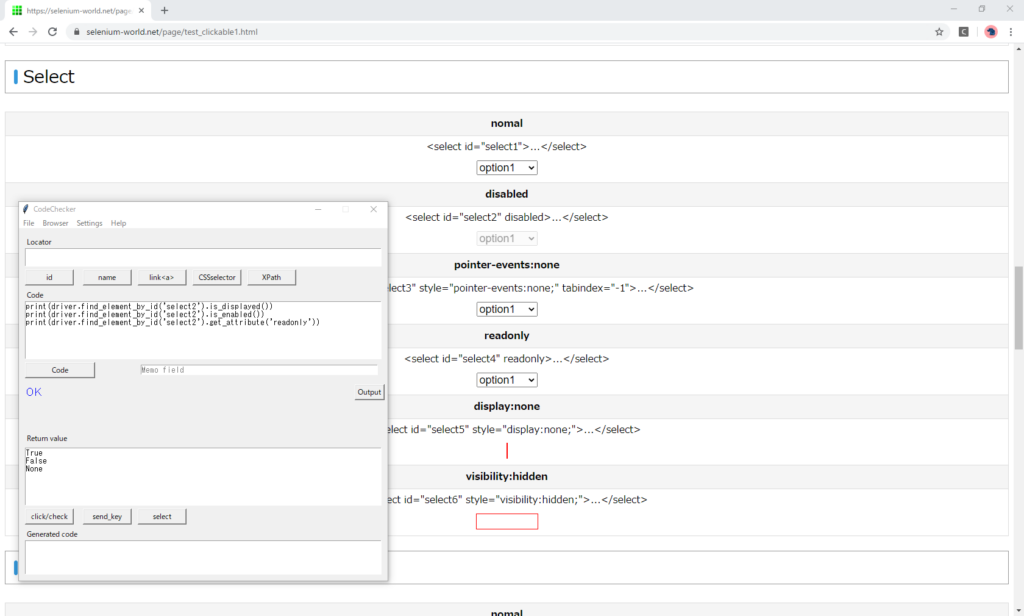
Inactive(pointer-events:none)
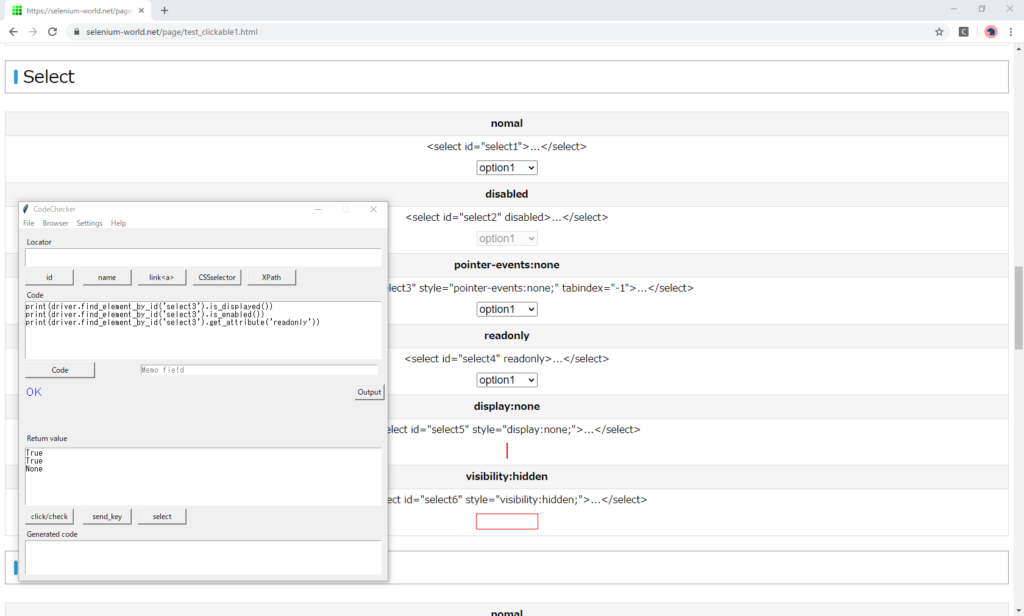
Read-only(readonly)
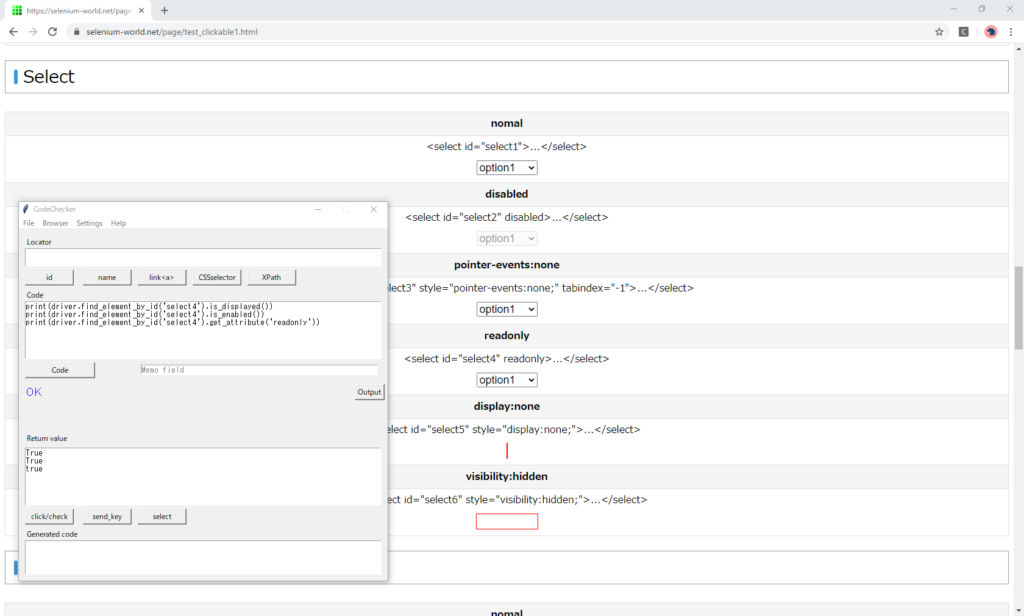
Hidden(display:none)
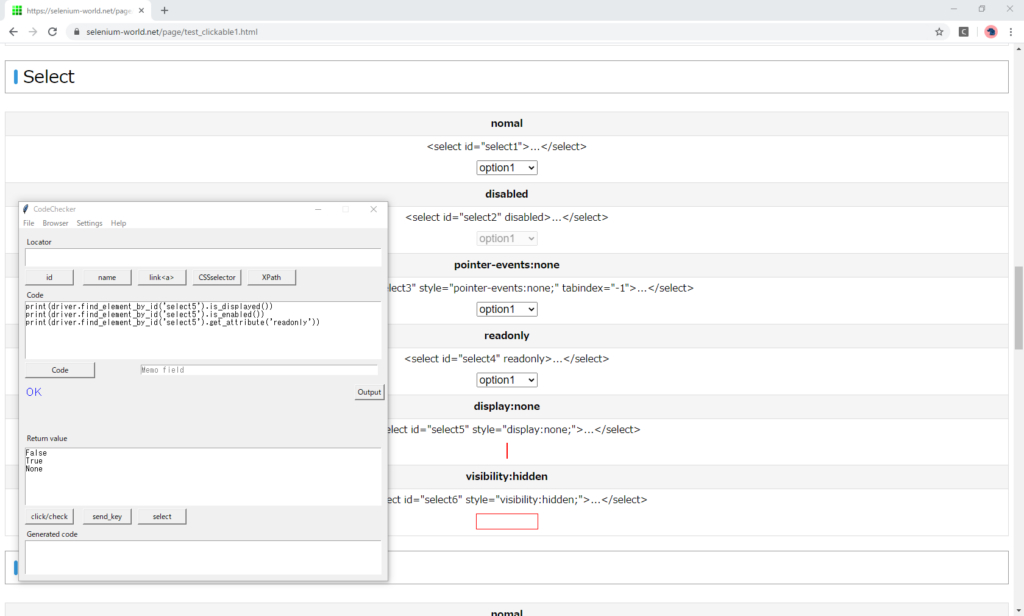
Hidden(visibility:hidden)
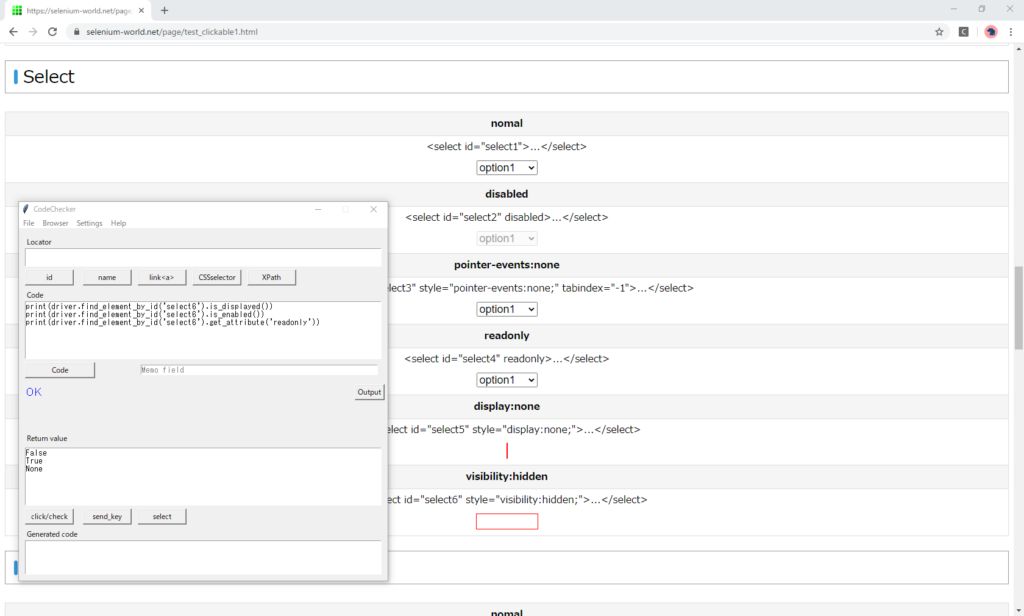
Result
Select | is_displayed() | is_enabled() | readonly |
---|---|---|---|
normal | True | True | None |
disabled | True | False | None |
pointer-events:none | True | True | None |
readonly | True | True | true |
display:none | False | True | None |
visibility:hidden | False | True | None |
Select(option)
Inspect for option2
Select item
Normal
“option2" is selected.
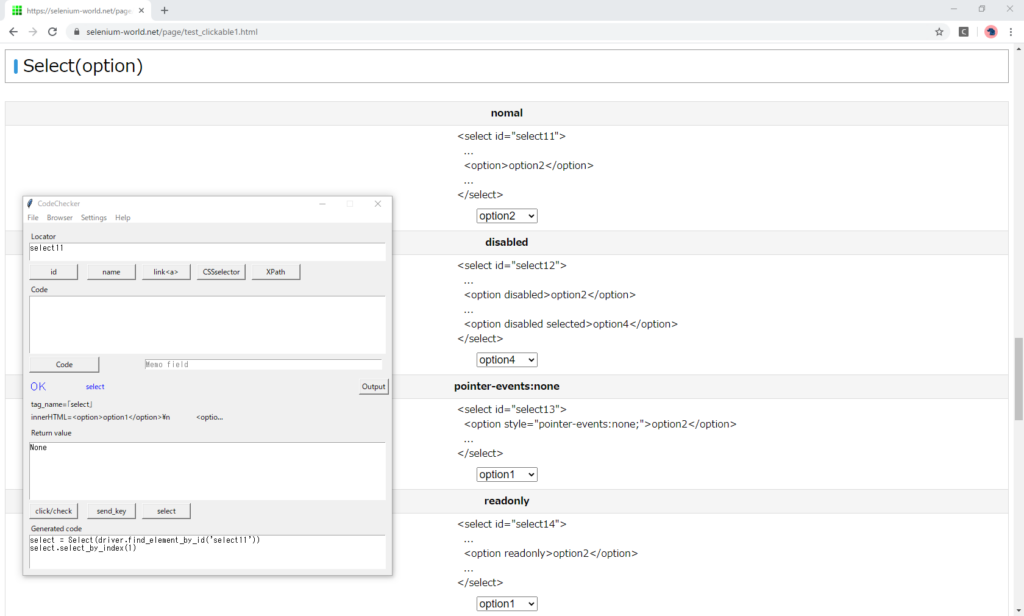
Inactive(disabled)
Not selectable.
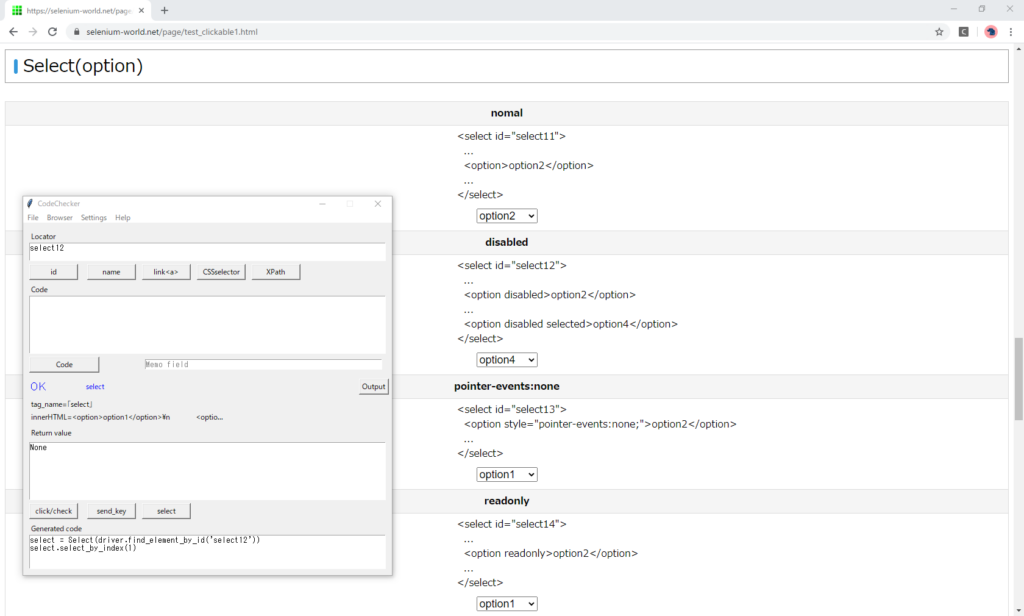
Not selectable manually.
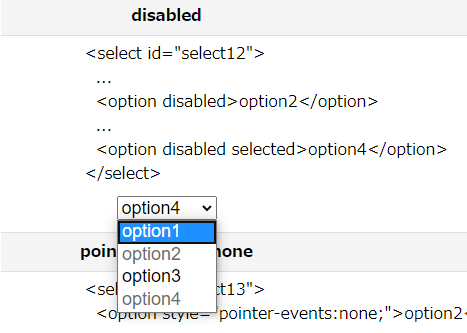
Inactive(pointer-events:none)
Not selectable.
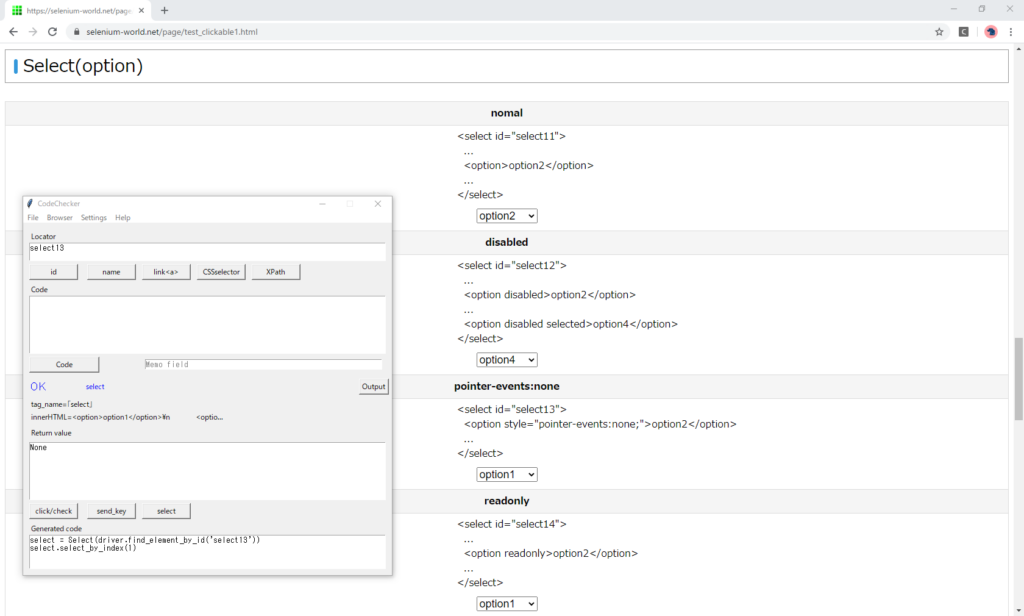
You can select “option2" manually.
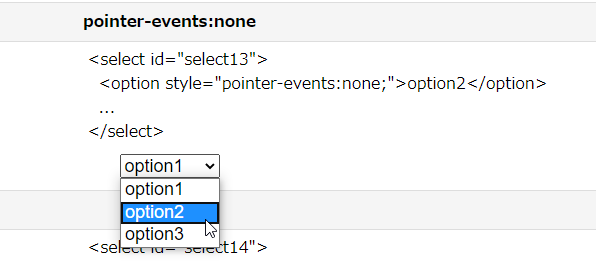
Read-only(readonly)
“option2" is selected.
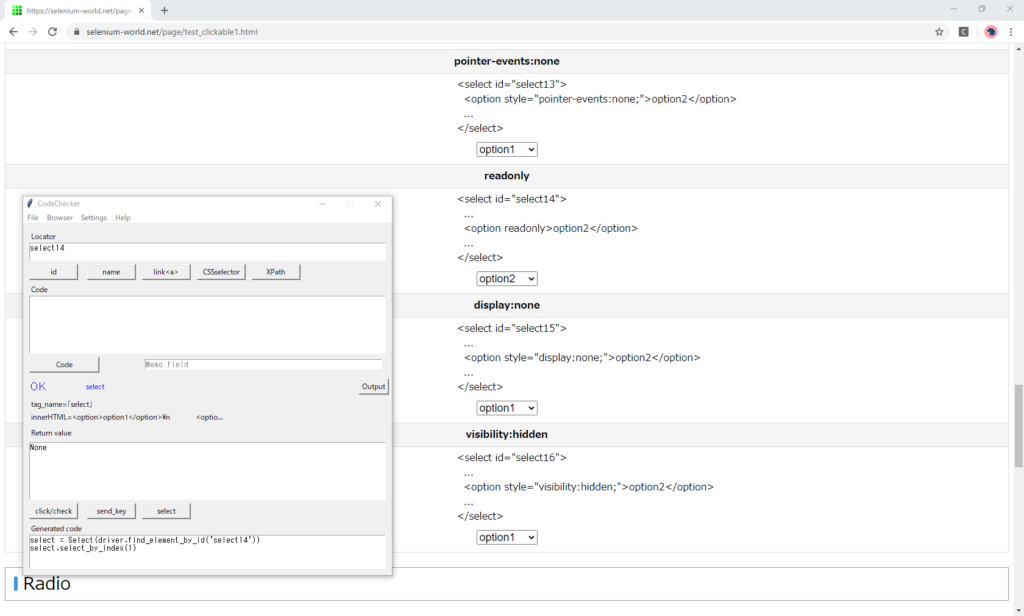
Hidden(display:none)
“option2" is selected.
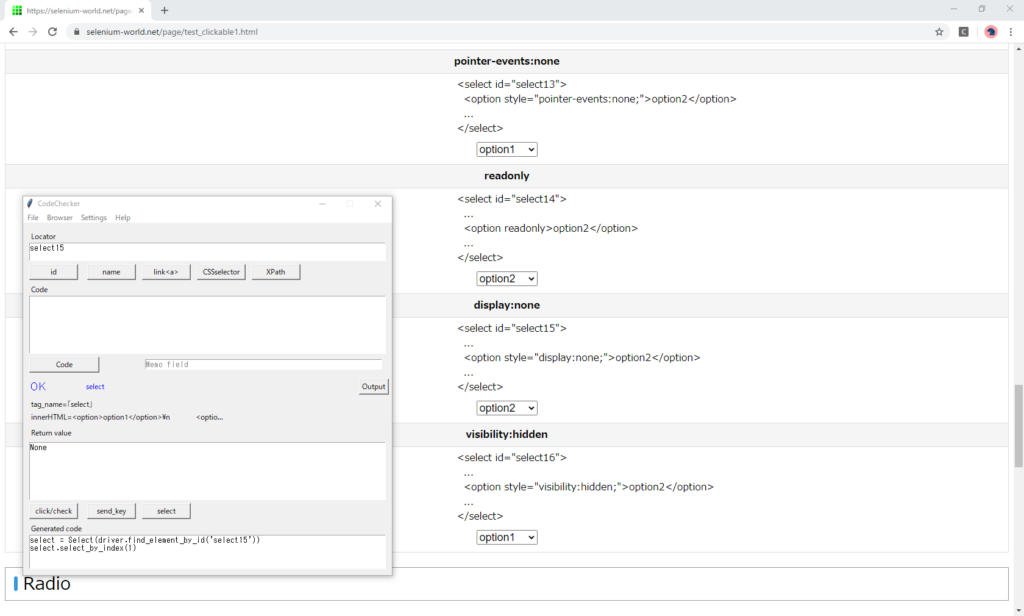
You cannot select “option2" manually.
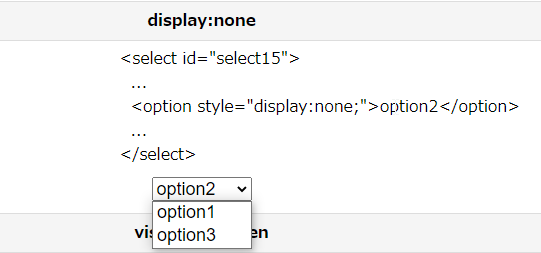
Hidden(visibility:hidden)
“option2" is selected.
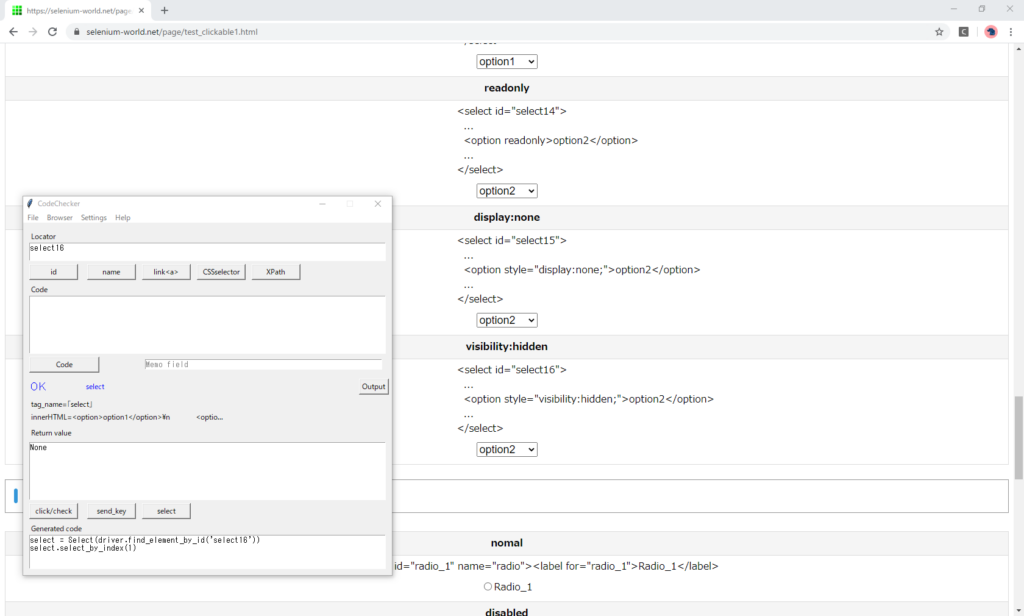
You cannot select “option2" manually.
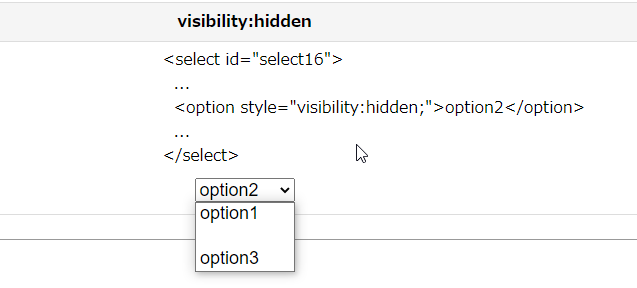
Get selected item
Normal
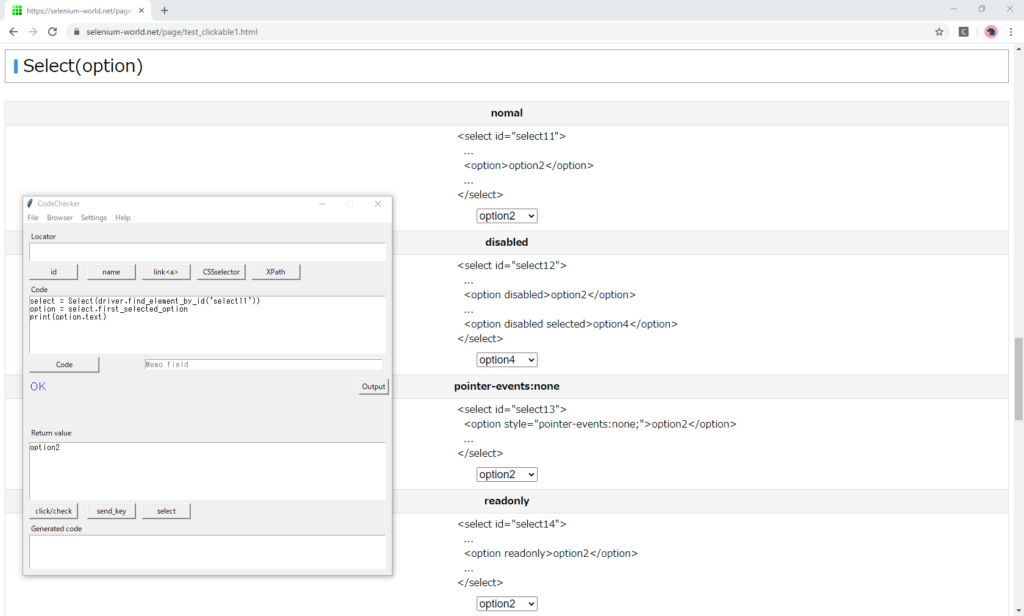
Inactive(disabled)
“option4" is disabled with “disabled", and selected with “selected".
“option4" is displayed.
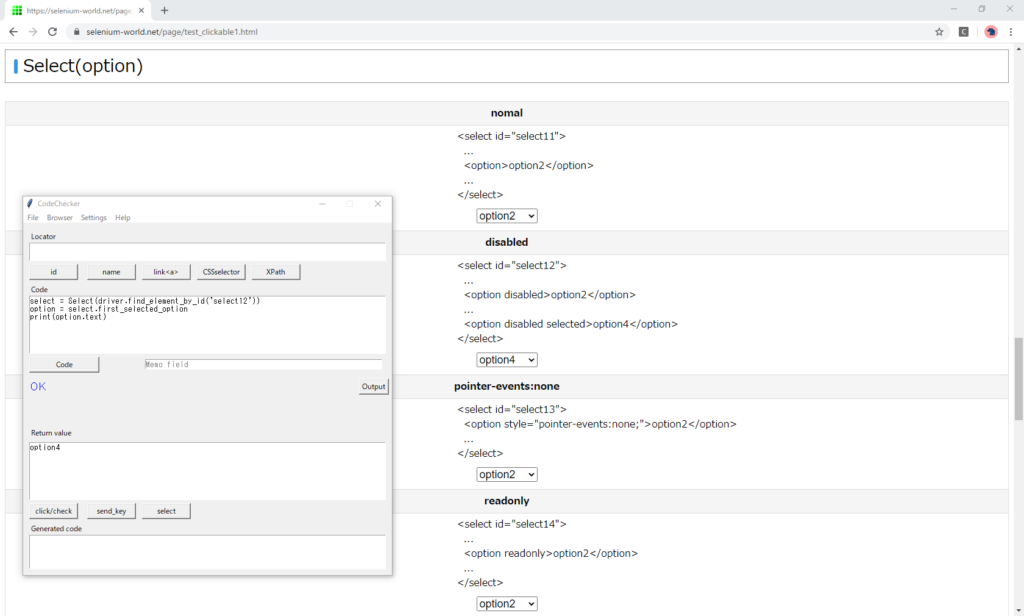
Inactive(pointer-events:none)
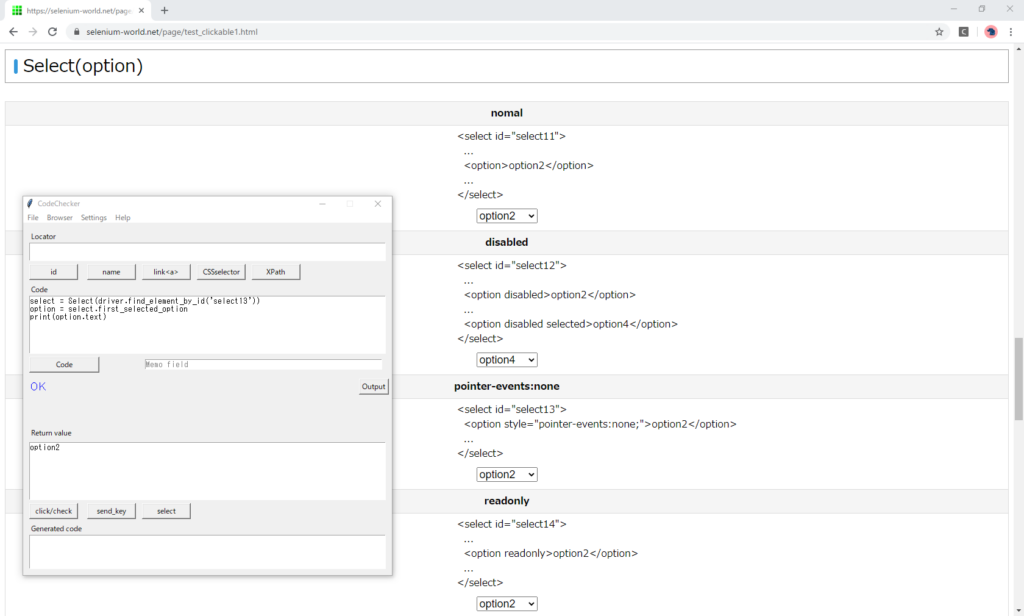
Read-only(readonly)
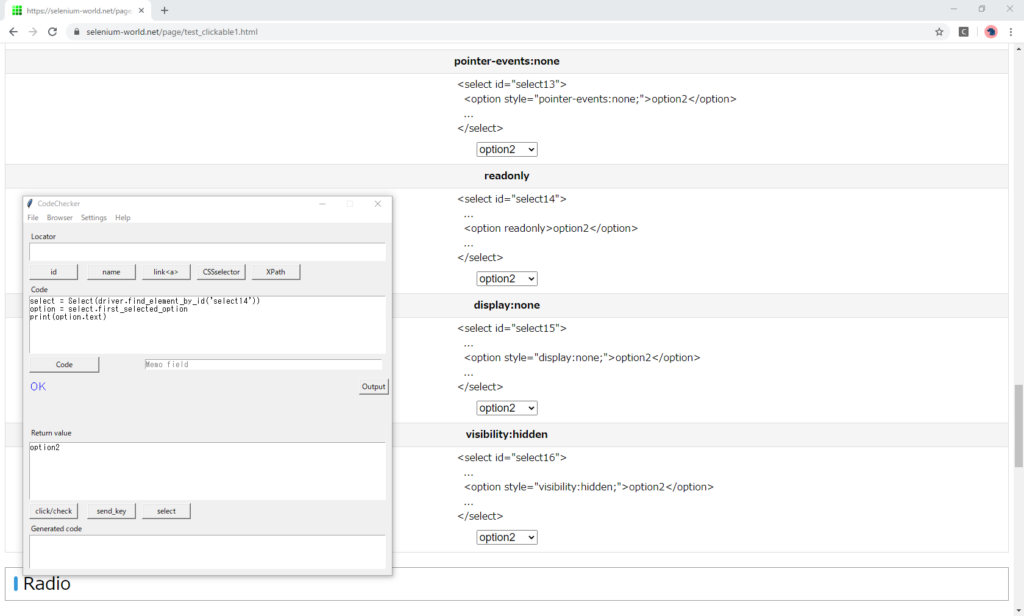
Hidden(display:none)
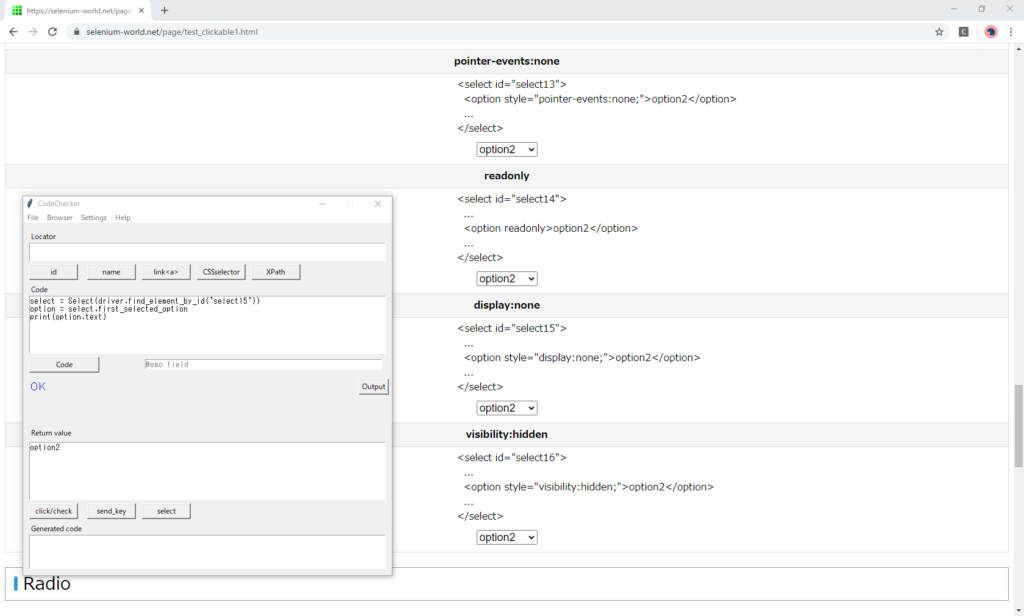
Hidden(visibility:hidden)
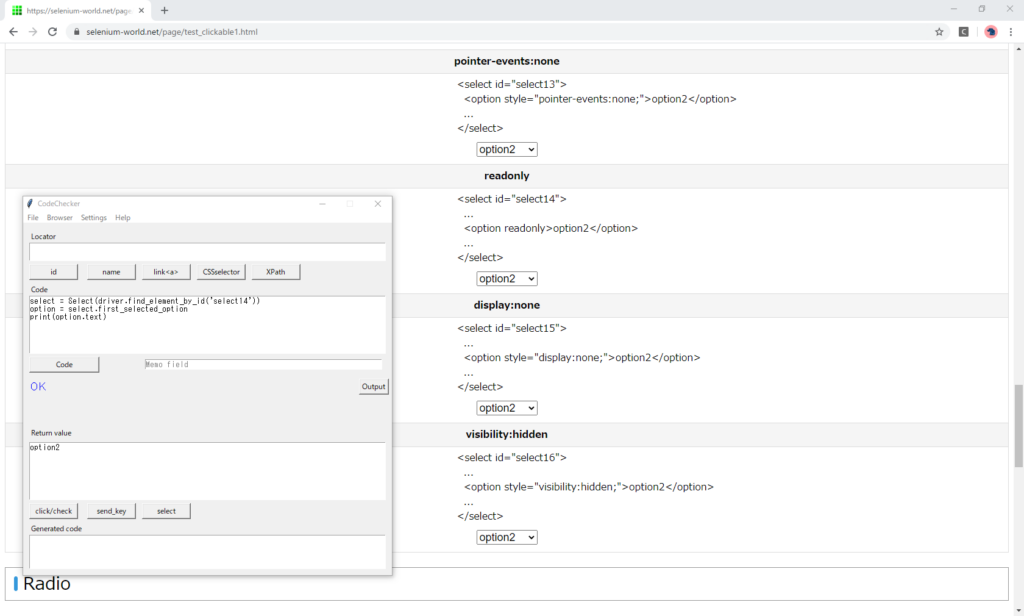
Result
Select(option) | Select item | get selected item | note |
---|---|---|---|
normal | OK | OK | |
disabled | NG | OK | |
pointer-events:none | *NG | OK | *Selectable manually |
readonly | OK | OK | |
display:none | *OK | OK | *Not selectable manually |
visibility:hidden | *OK | OK | *Not selectable manually |
Get Attribute
Normal
Input the following codes, and press the “Code" button.
select = Select(driver.find_element_by_id('select11'))
option = select.options[1]
print(option.text)
print(option.is_displayed())
print(option.is_enabled())
print(option.get_attribute('readonly'))
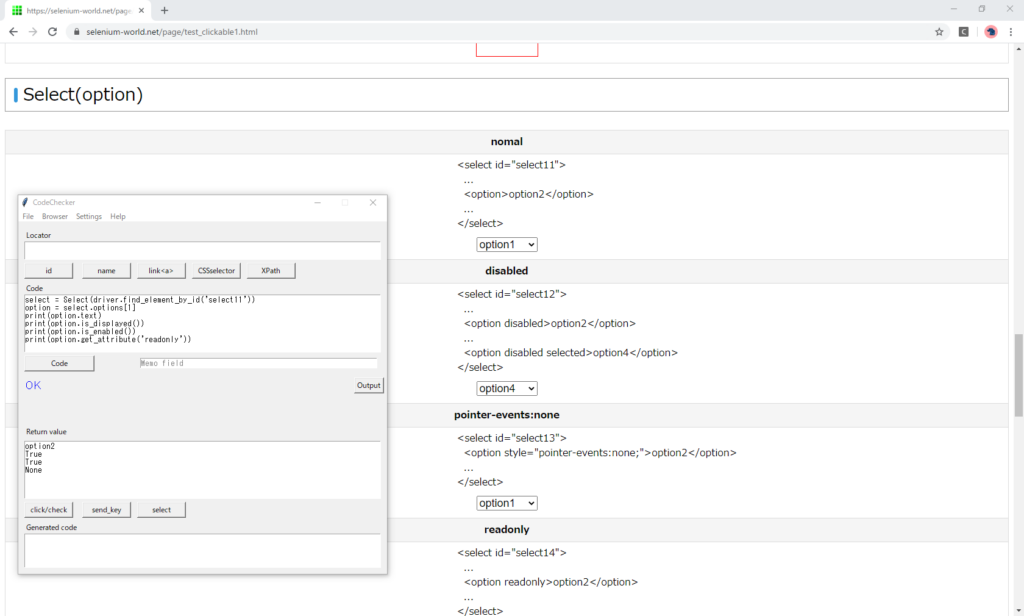
Inactive(disabled)
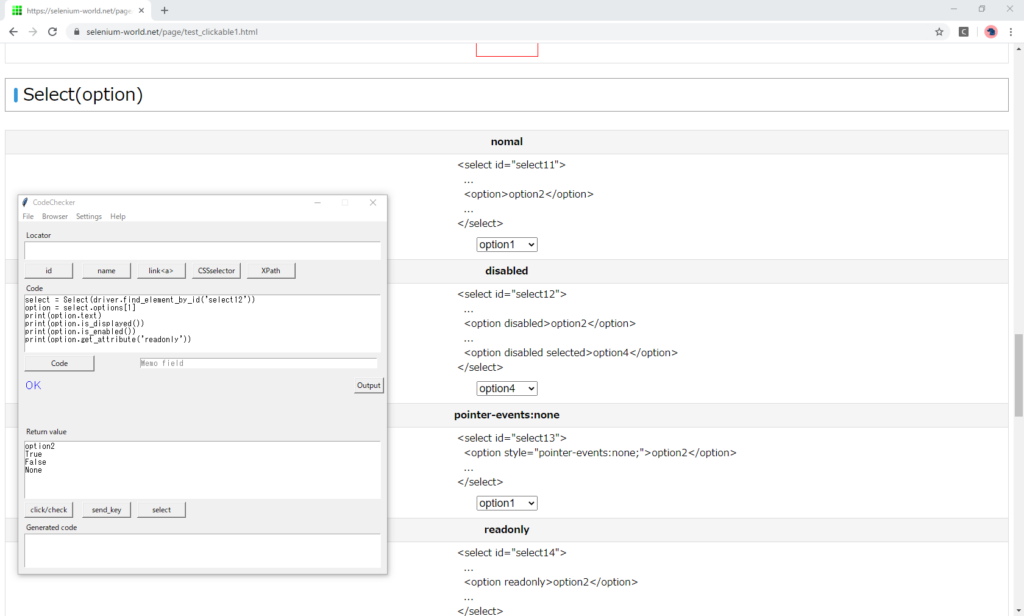
Inactive(pointer-events:none)
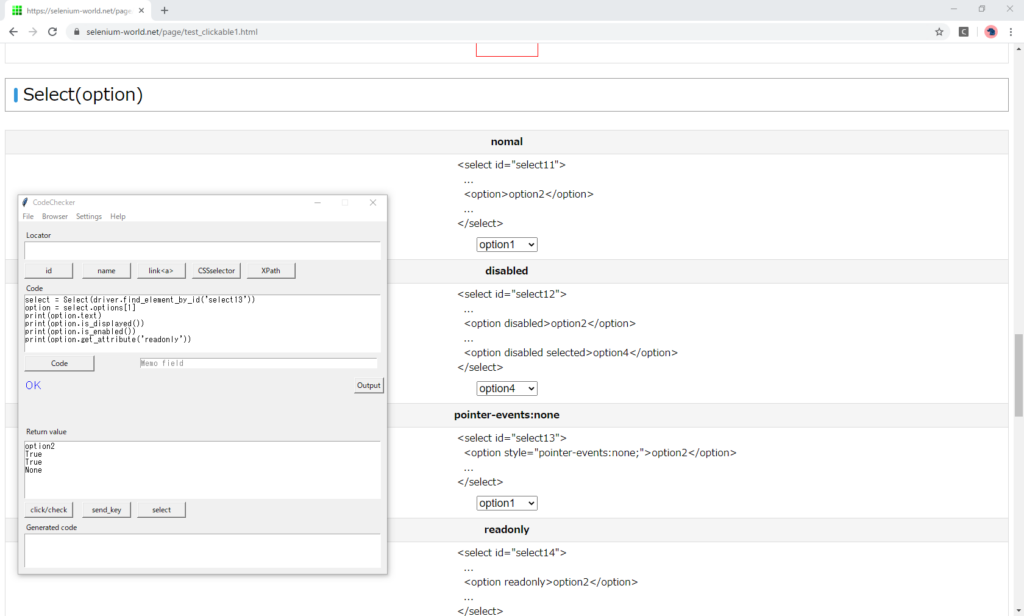
Read-only(readonly)
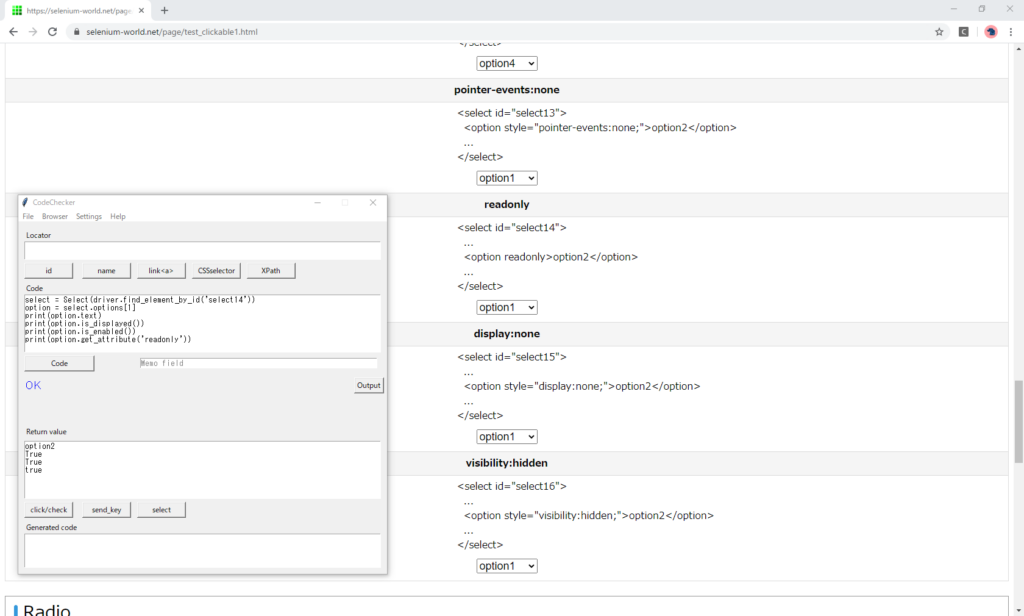
Hidden(display:none)
Although “option2" is hidden, “is_displayed" is “True".
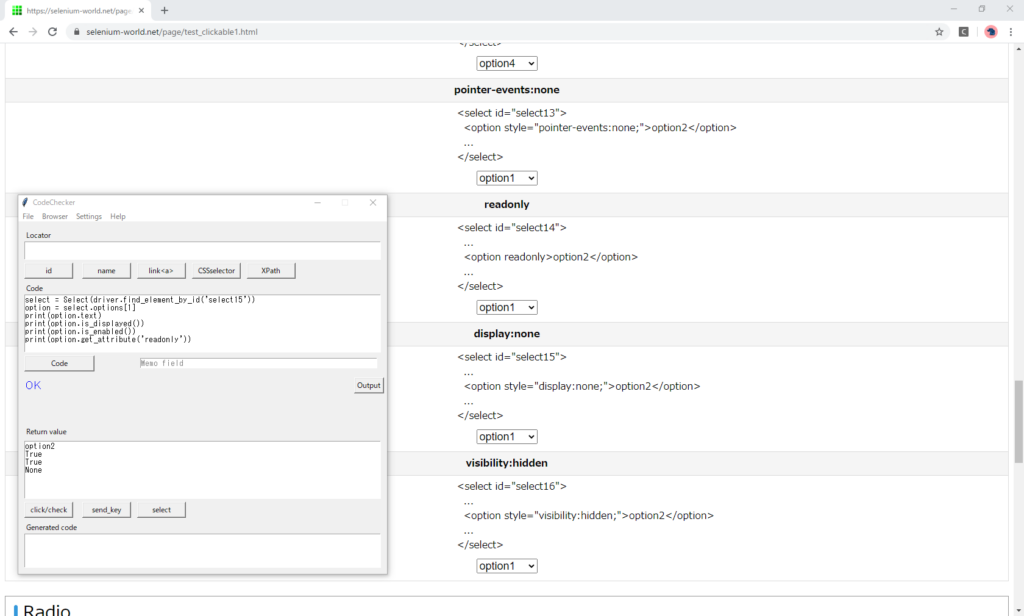
Hidden(visibility:hidden)
Although “option2" is hidden, “is_displayed" is “True".
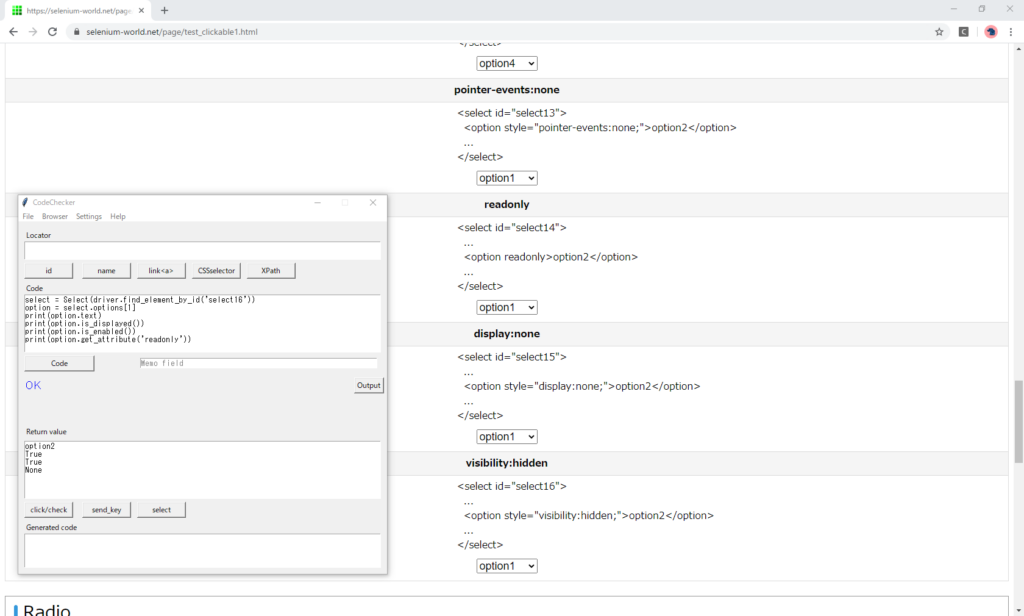
Result
Select(option) | is_displayed() | is_enabled() | readonly |
---|---|---|---|
normal | True | True | None |
disabled | True | False | None |
pointer-events:none | True | True | None |
readonly | True | True | true |
display:none | True | True | None |
visibility:hidden | True | True | None |
Radio
Click
Normal
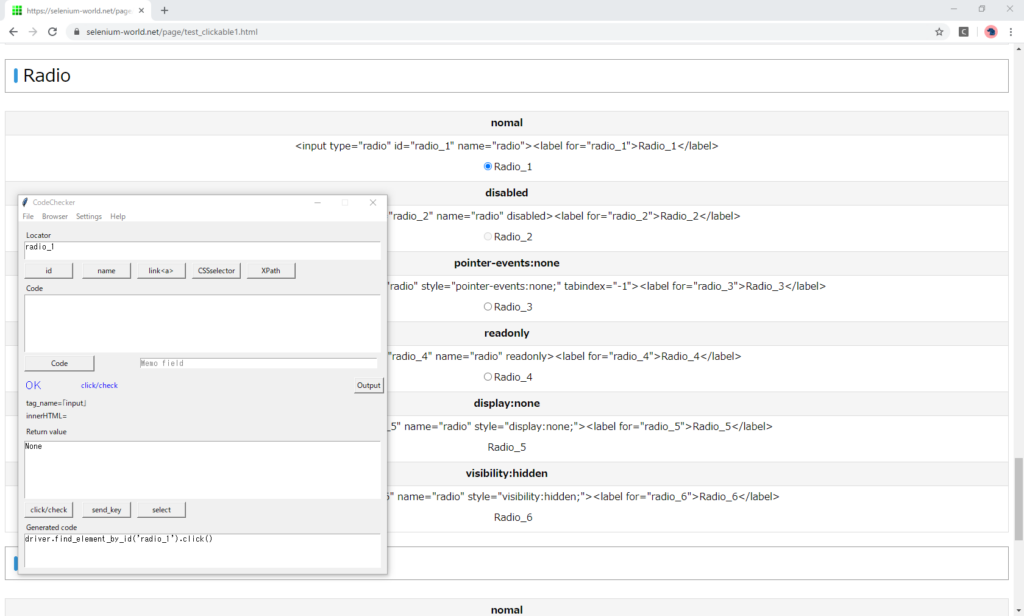
Inactive(disabled)
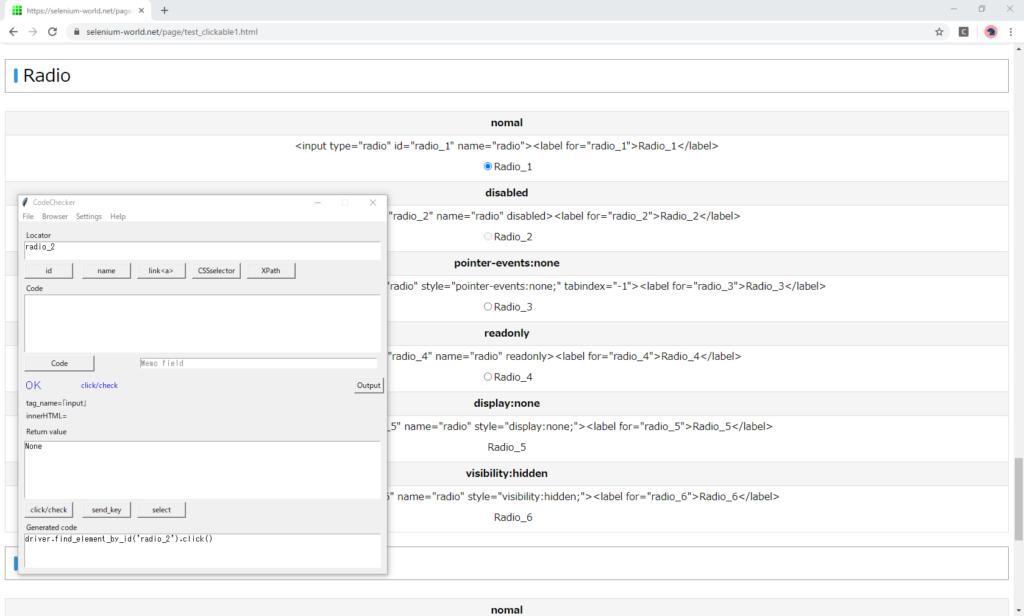
Inactive(pointer-events:none)
Exception occurred.
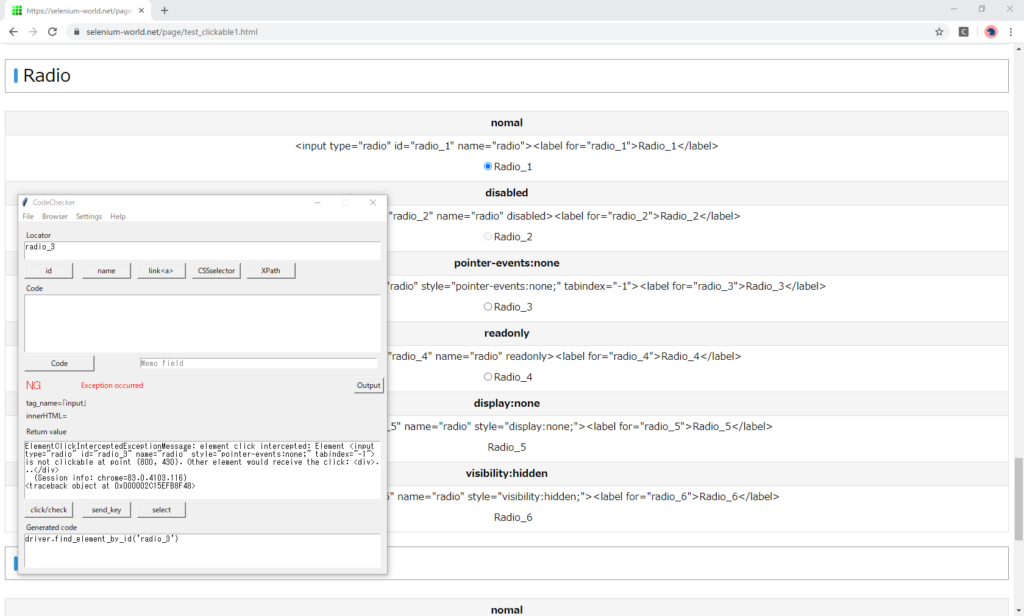
ElementClickInterceptedExceptionMessage: element click intercepted: Element <input type="radio" id="radio_3" name="radio" style="pointer-events:none;" tabindex="-1"> is not clickable at point (800, 430). Other element would receive the click: <div>...</div>
(Session info: chrome=83.0.4103.116)
<traceback object at 0x000002C15EFB8F48>
Read-only(readonly)
It is clickable.
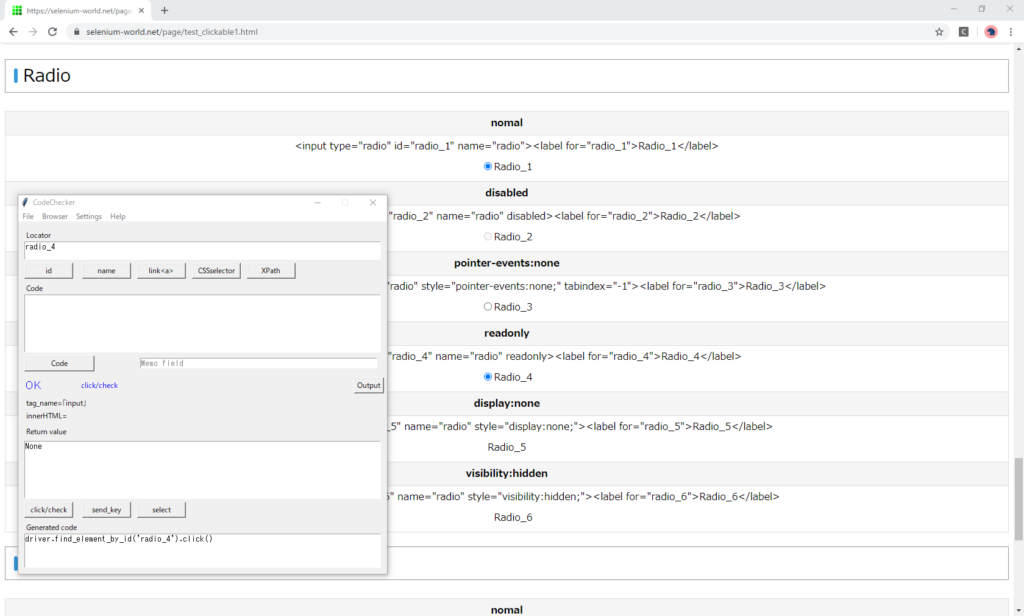
Hidden(display:none)
Exception occurred.
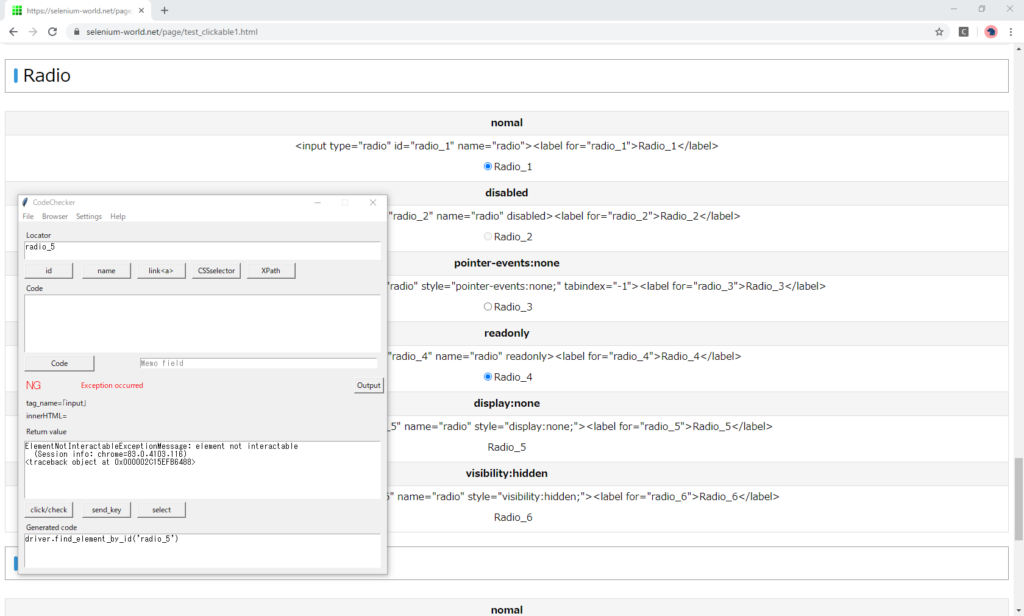
Message
ElementNotInteractableExceptionMessage: element not interactable
(Session info: chrome=83.0.4103.116)
<traceback object at 0x000002C15EFB6488>
Hidden(visibility:hidden)
Exception occurred.
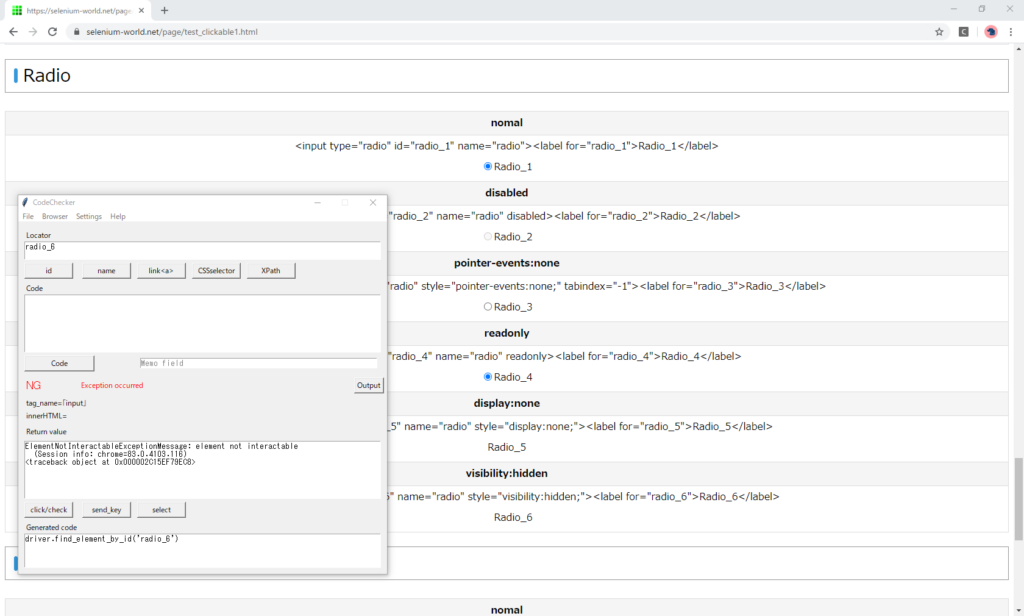
Get value
Normal
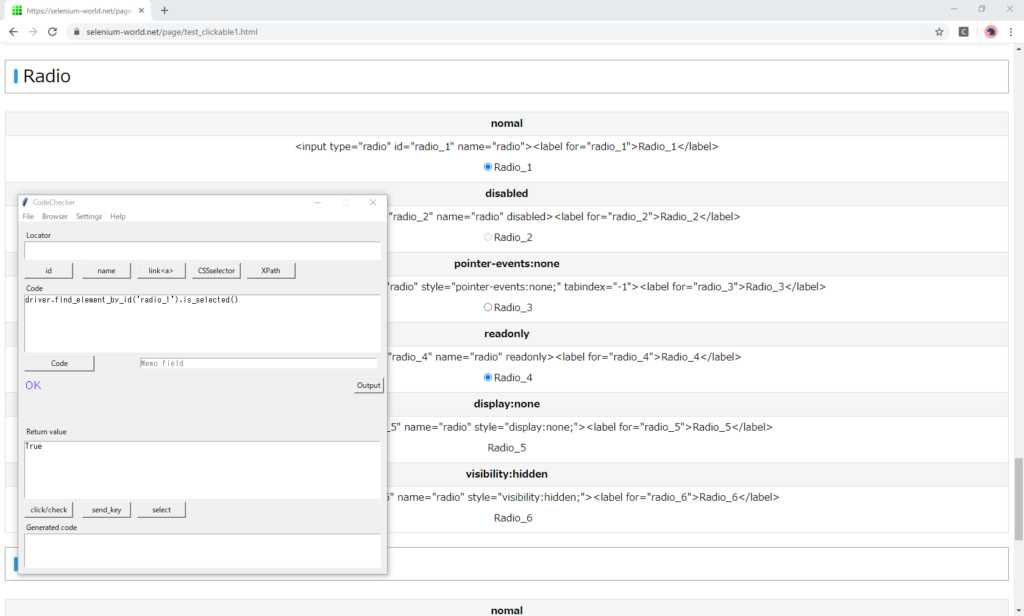
Inactive(disabled)
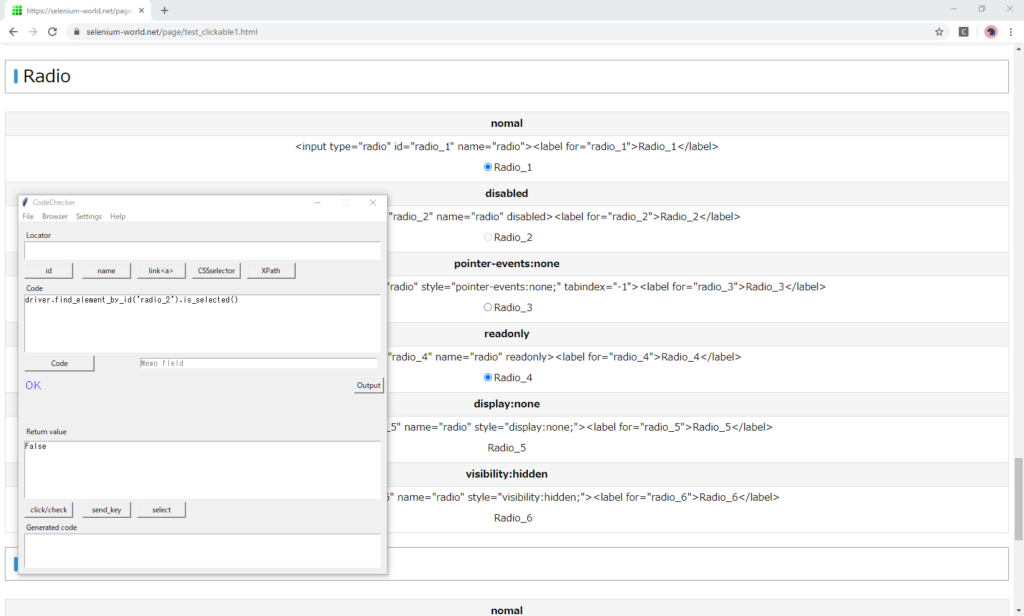
Inactive(pointer-events:none)
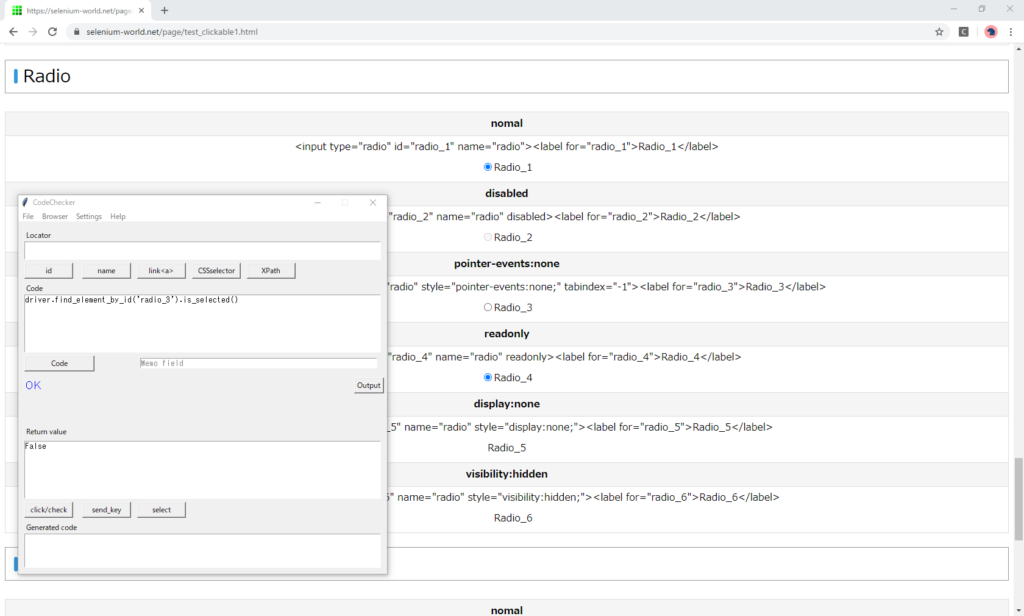
Read-only(readonly)
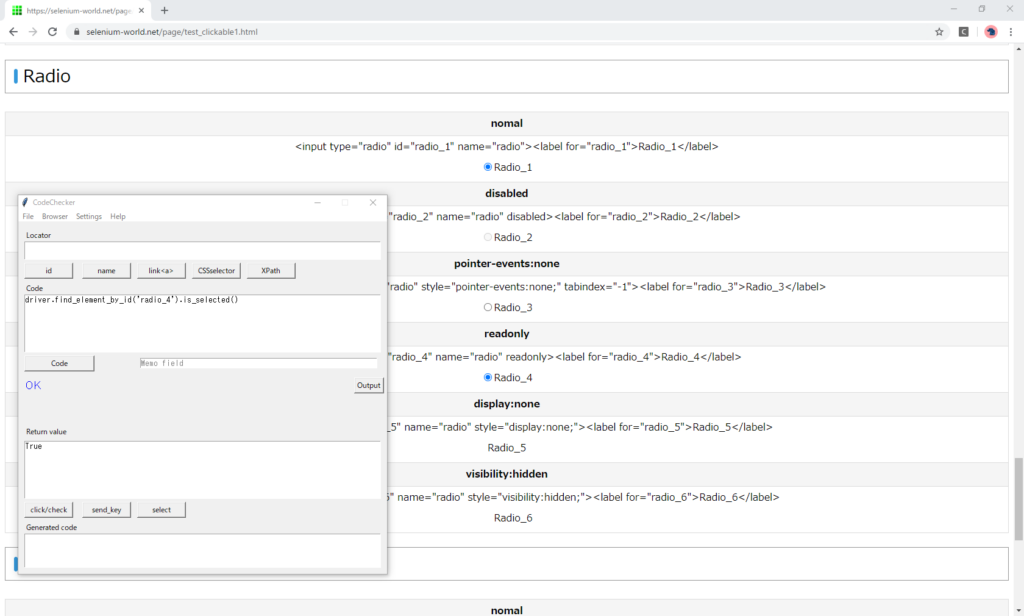
Hidden(display:none)
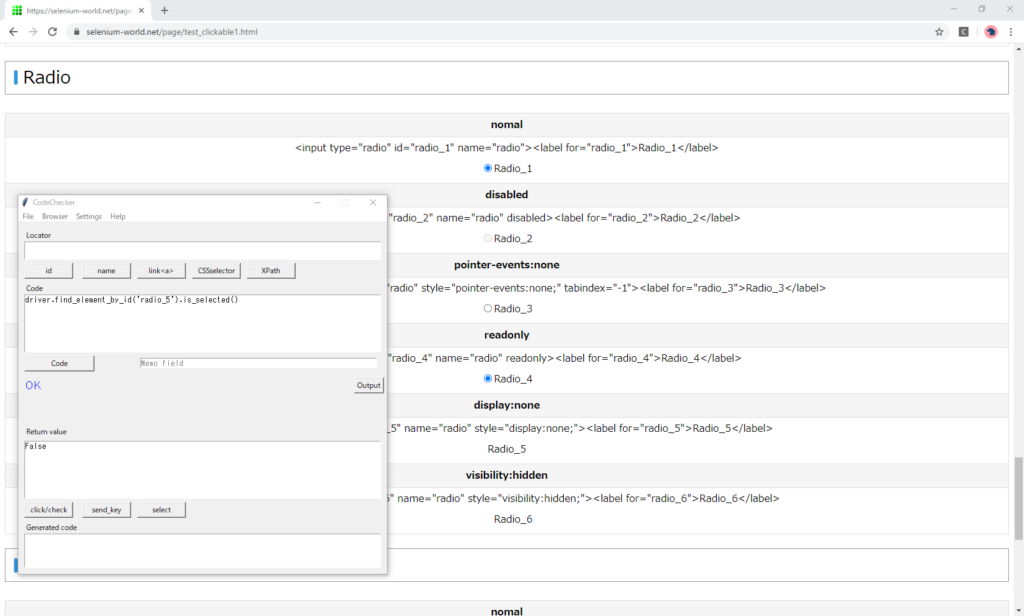
Hidden(visibility:hidden)
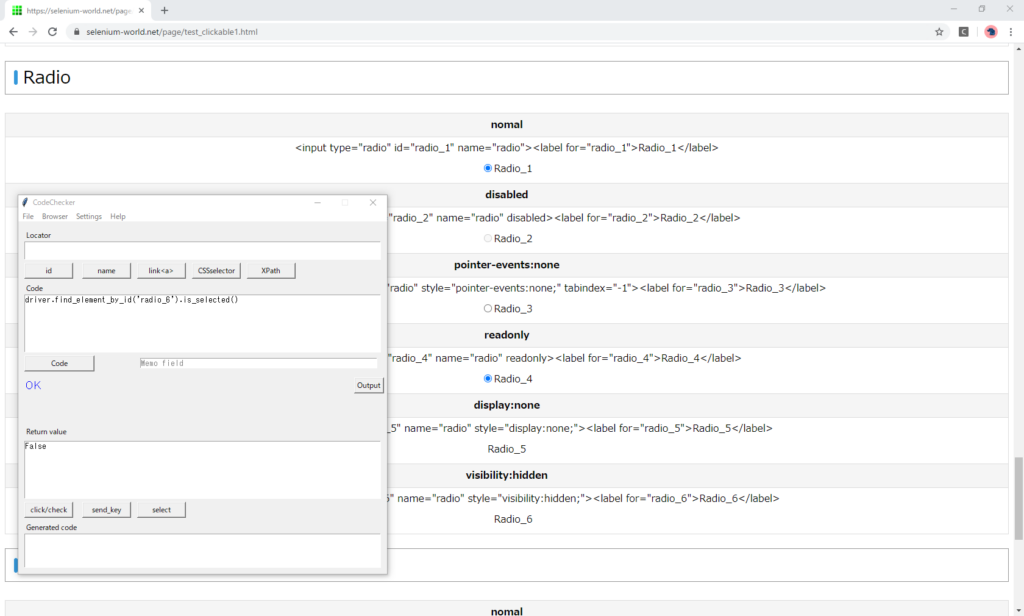
Behavior when label is clicked
The Radio button is specified “label for", so you can check it by clicking label.
Normal
Enter the XPath obtained by XPathGetter in the locator field and press the “XPath" button.
The escaped code to get the label element is outputted.
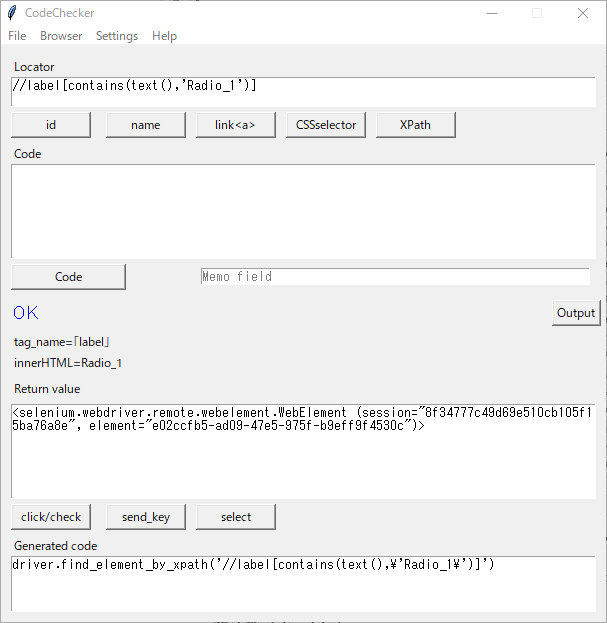
Input the following codes, and press the “Code" button.
driver.find_element_by_xpath('//label[contains(text(),\'Radio_1\')]').click()
print(driver.find_element_by_id('radio_1').is_selected())
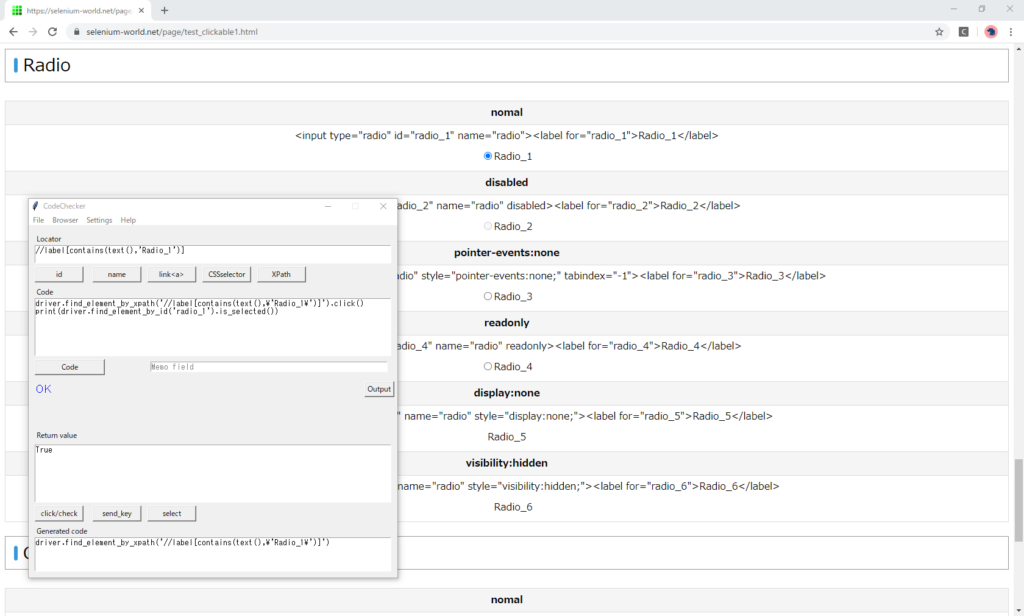
Inactive(disabled)
Not checkable by clicking label.
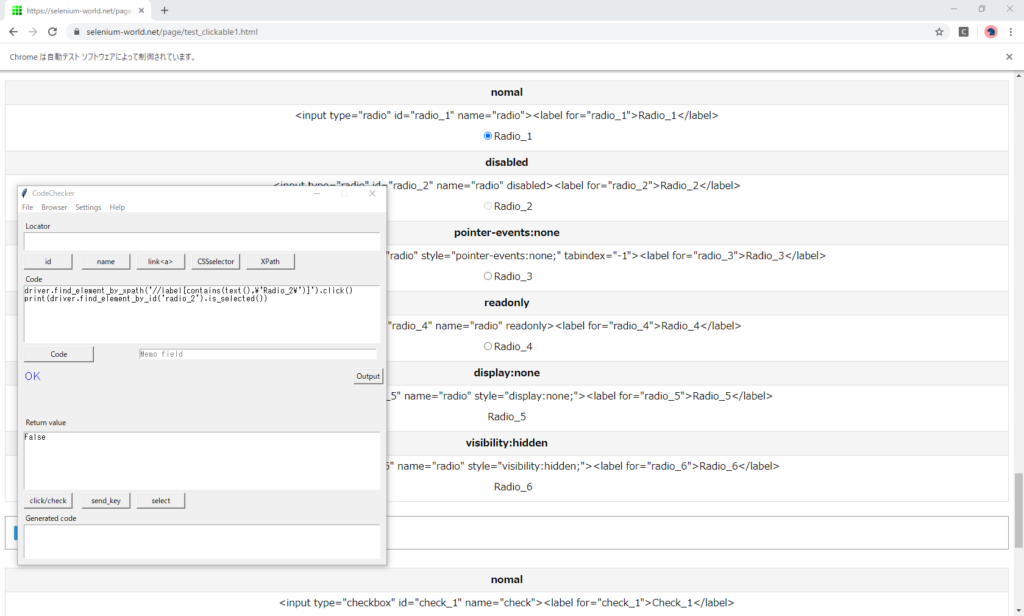
Inactive(pointer-events:none)
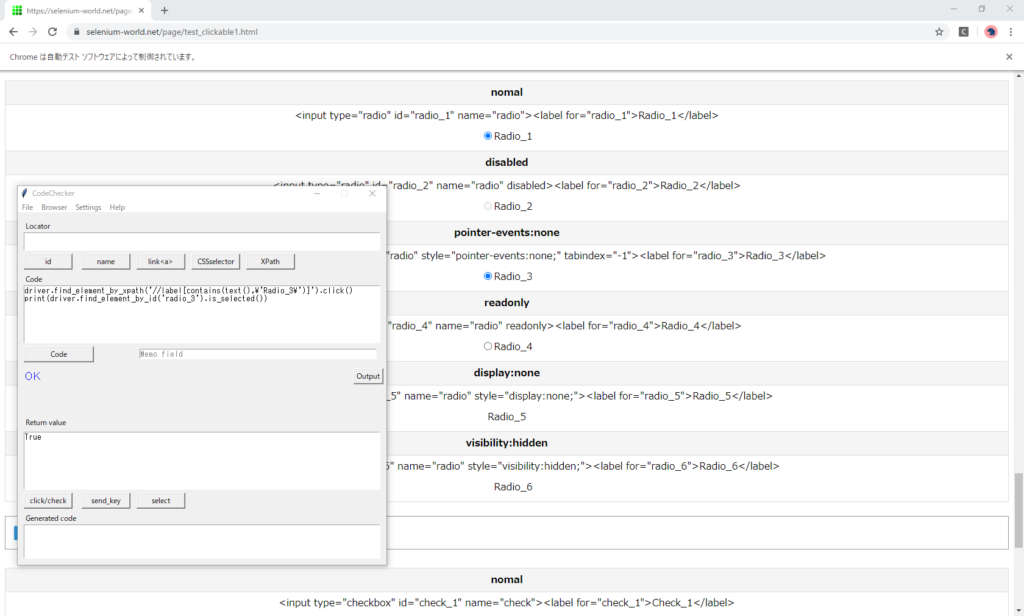
Read-only(readonly)
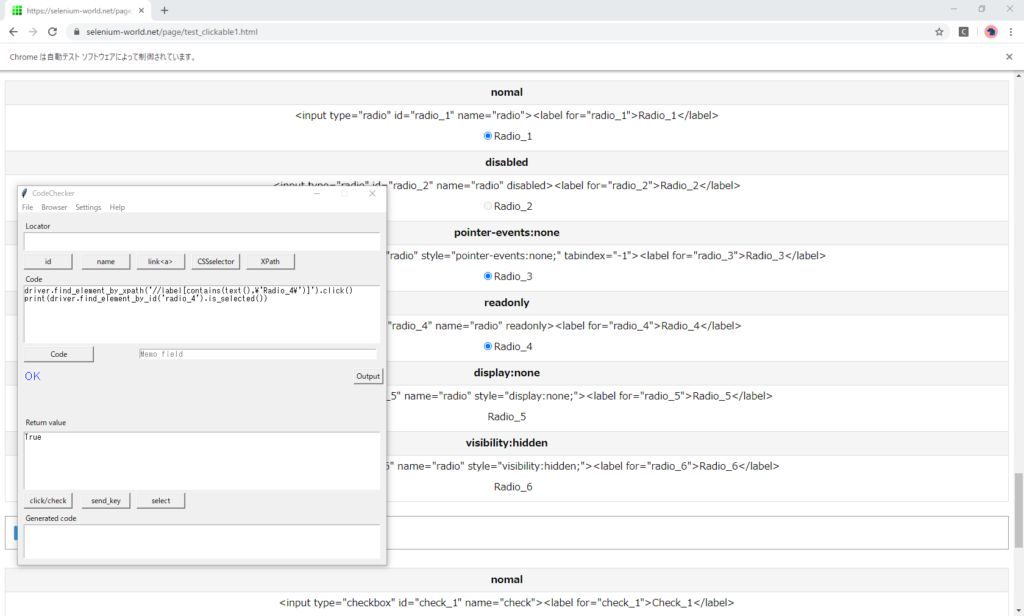
Hidden(display:none)
It is not displayed, but the button is checked by clicking the label.
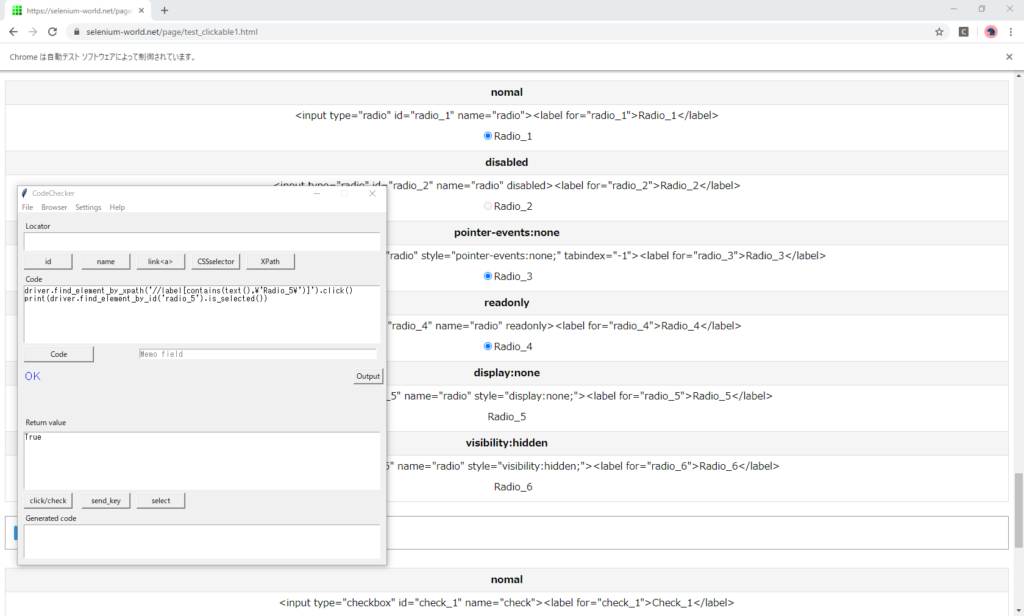
Hidden(visibility:hidden)
It is not displayed, but the button is checked by clicking the label.
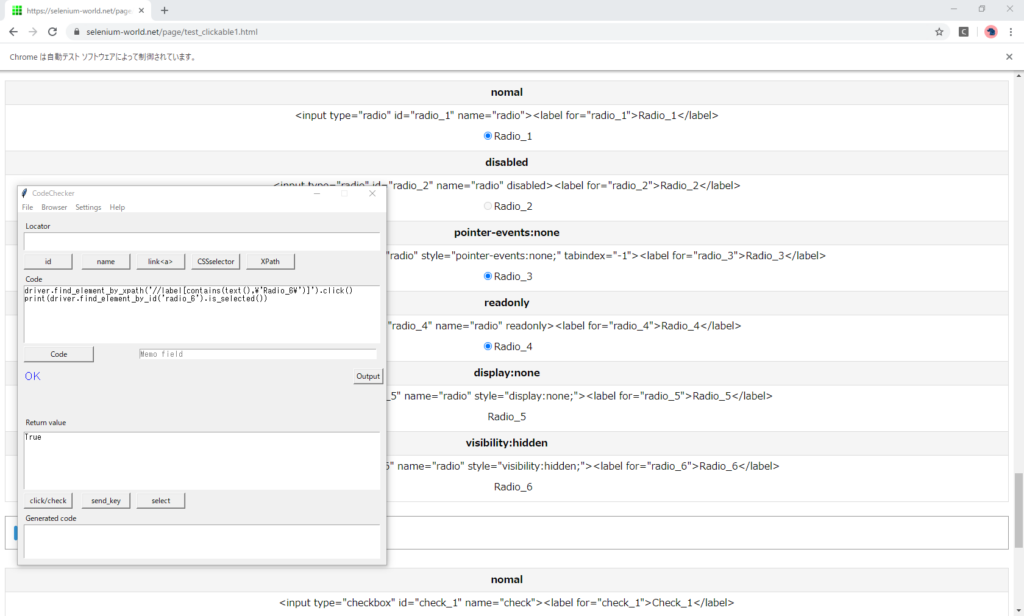
Result
Radio | click radio | click label | get value |
---|---|---|---|
normal | OK | OK | OK |
disabled | NG | NG | OK |
pointer-events:none | Ex | OK | OK |
readonly | OK | OK | OK |
display:none | Ex | OK | OK |
visibility:hidden | Ex | OK | OK |
Get Attribute
Normal
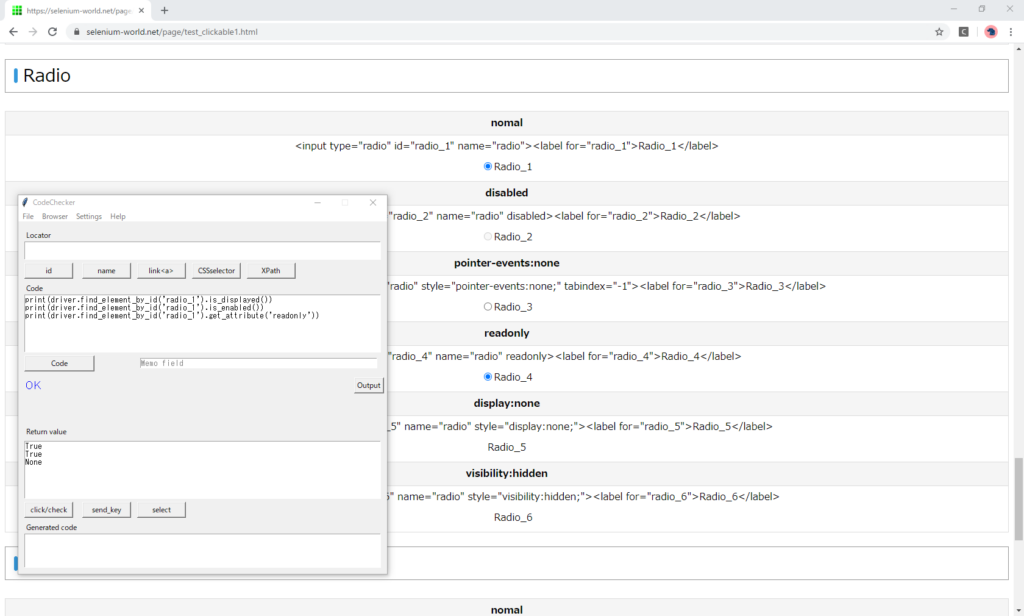
Inactive(disabled)
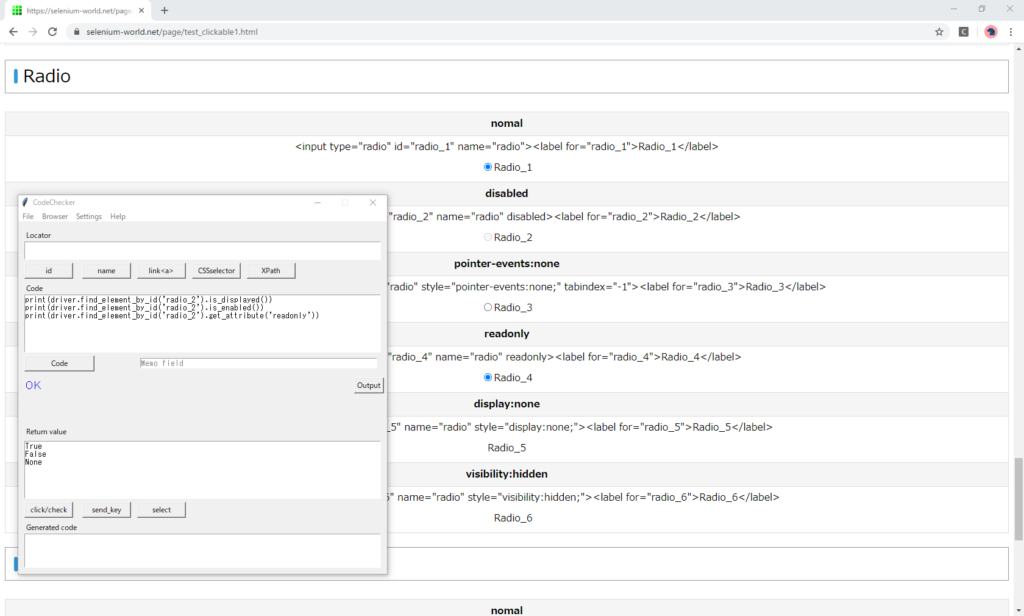
Inactive(pointer-events:none)
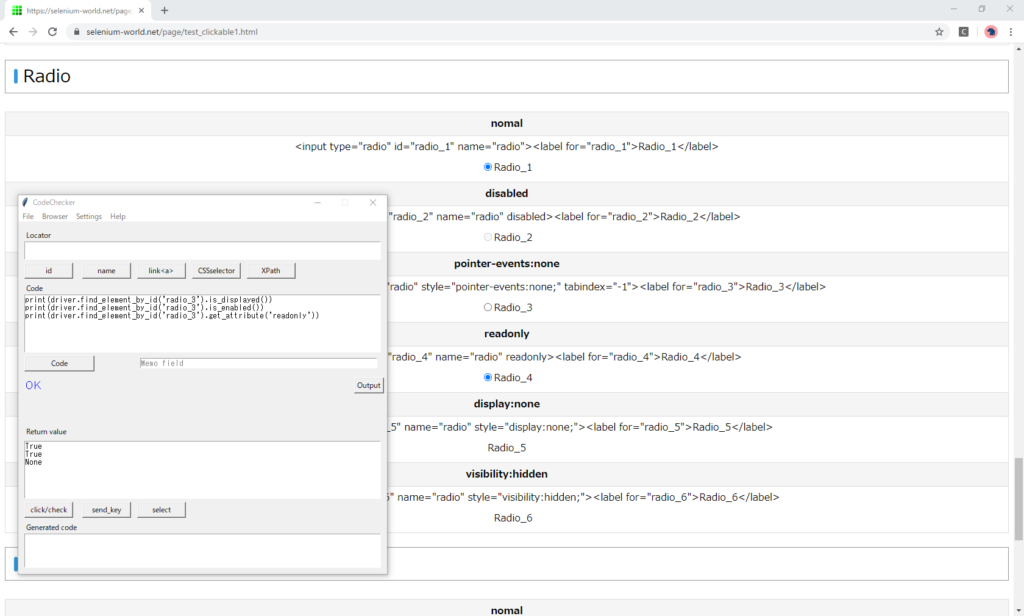
Read-only(readonly)
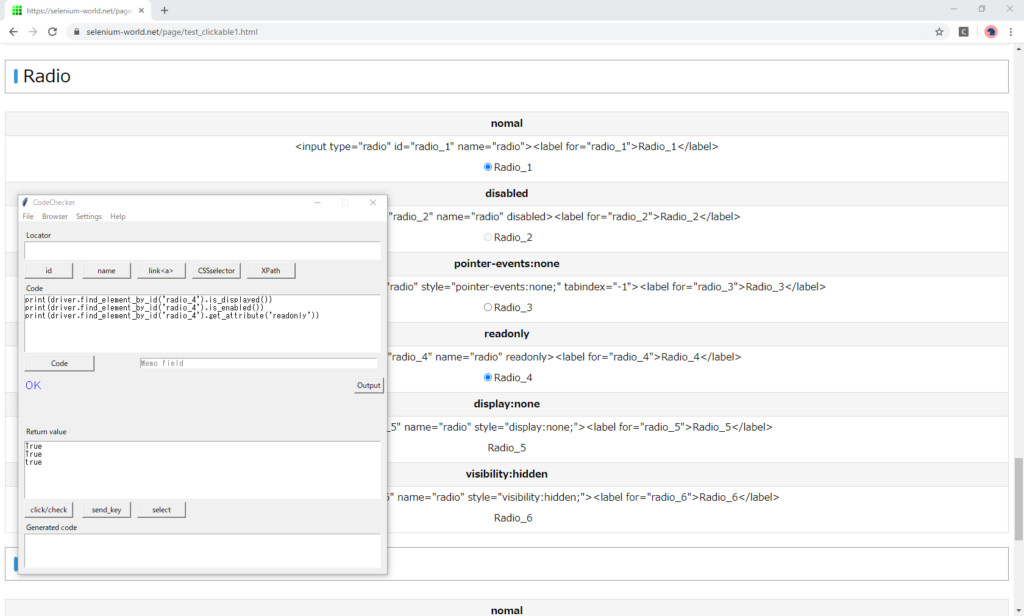
Hidden(display:none)
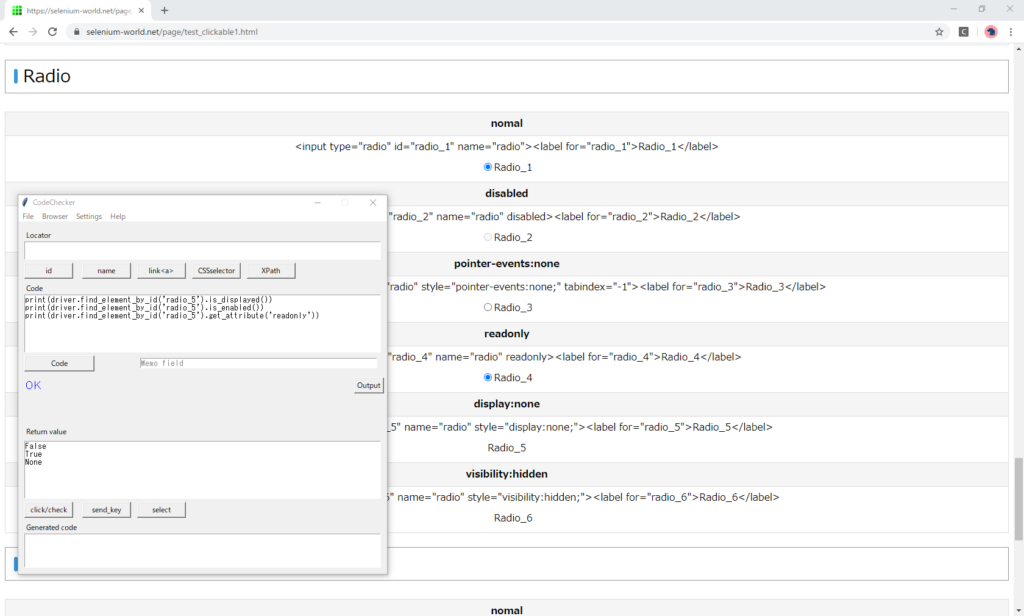
Hidden(visibility:hidden)
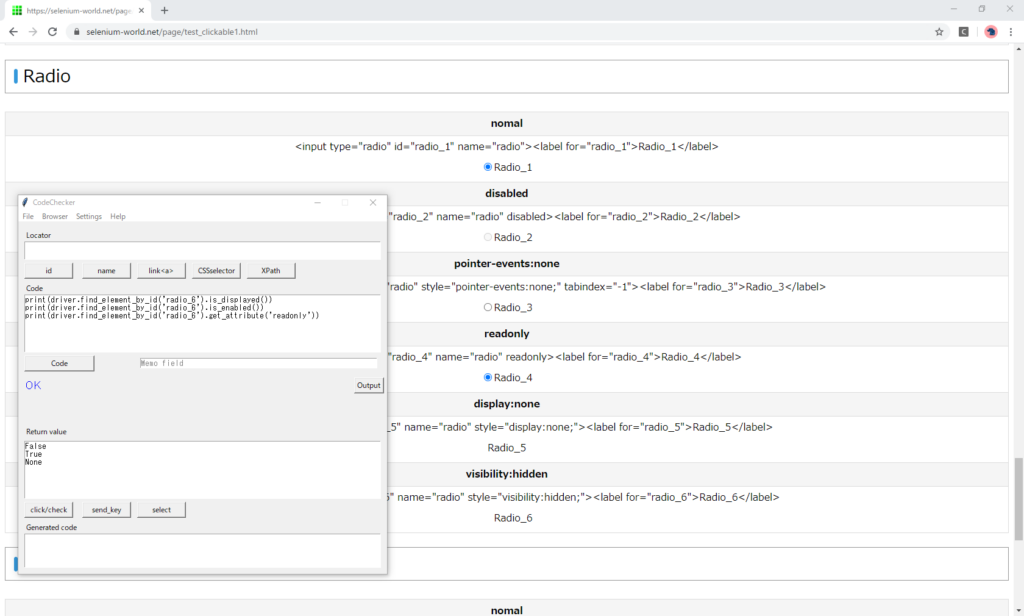
Result
Radio | is_displayed() | is_enabled() | readonly |
---|---|---|---|
normal | True | True | None |
disabled | True | False | None |
pointer-events:none | True | True | None |
readonly | True | True | true |
display:none | False | True | None |
visibility:hidden | False | True | None |
CheckBox
Click
Normal
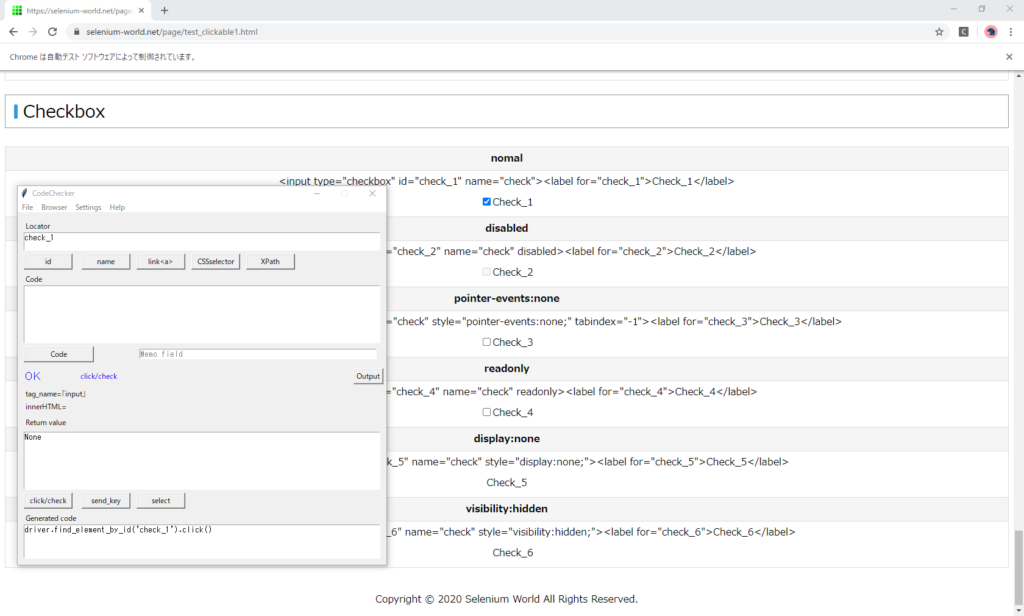
Inactive(disabled)
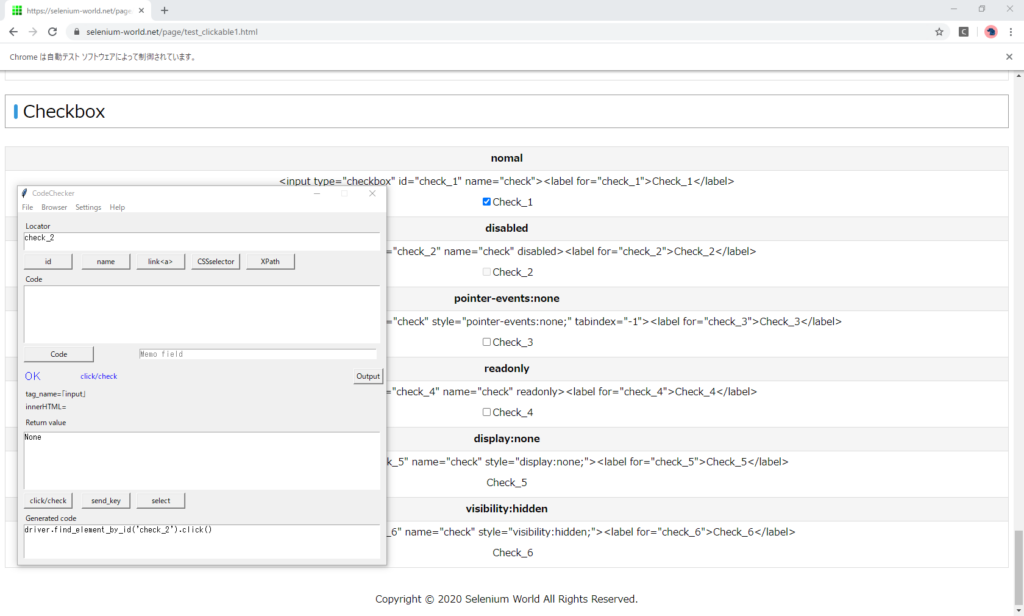
Inactive(pointer-events:none)
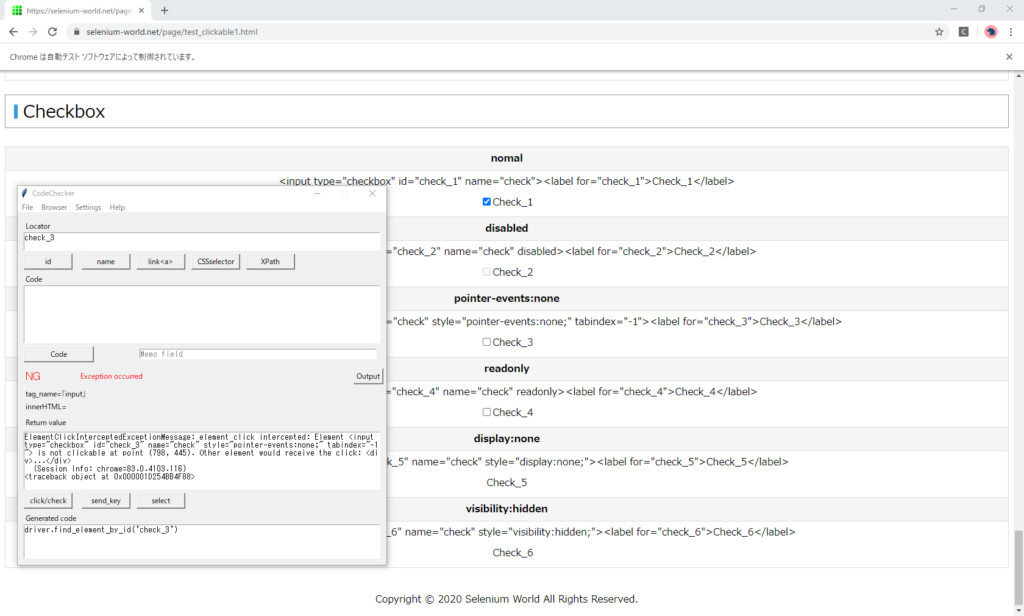
Read-only(readonly)
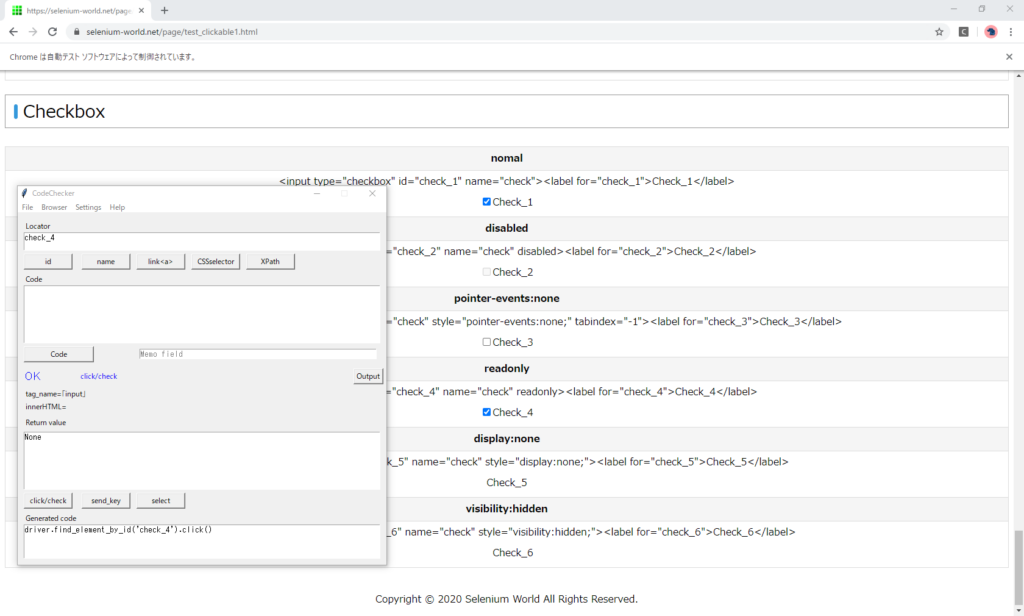
Hidden(display:none)
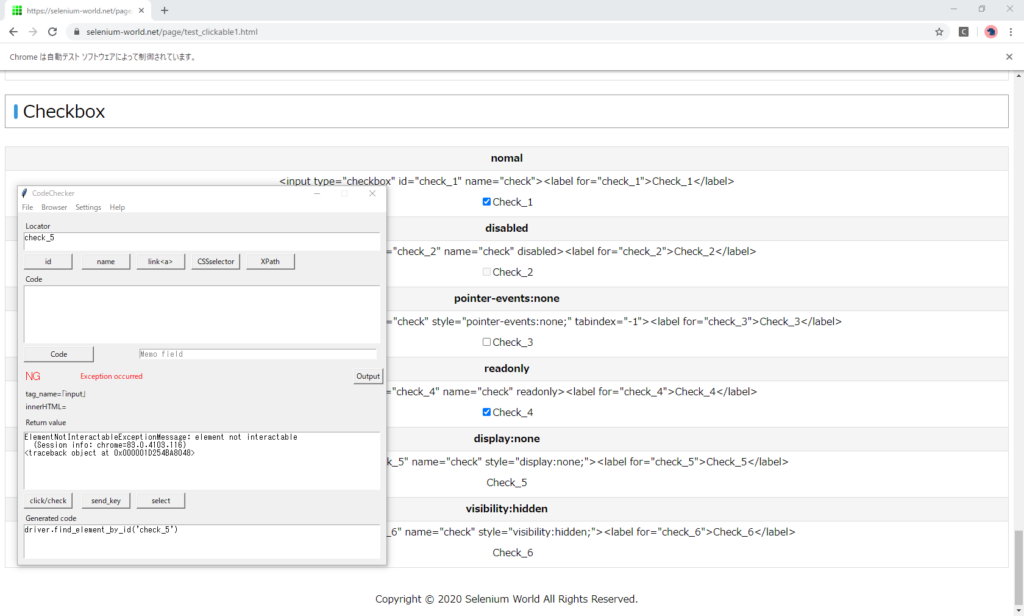
Hidden(visibility:hidden)
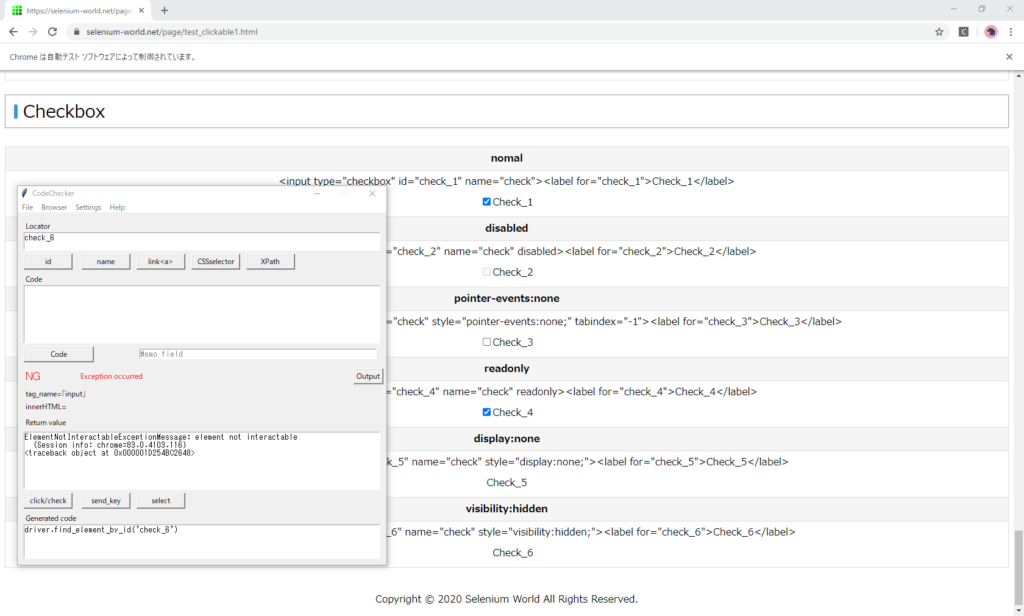
Get value
Normal
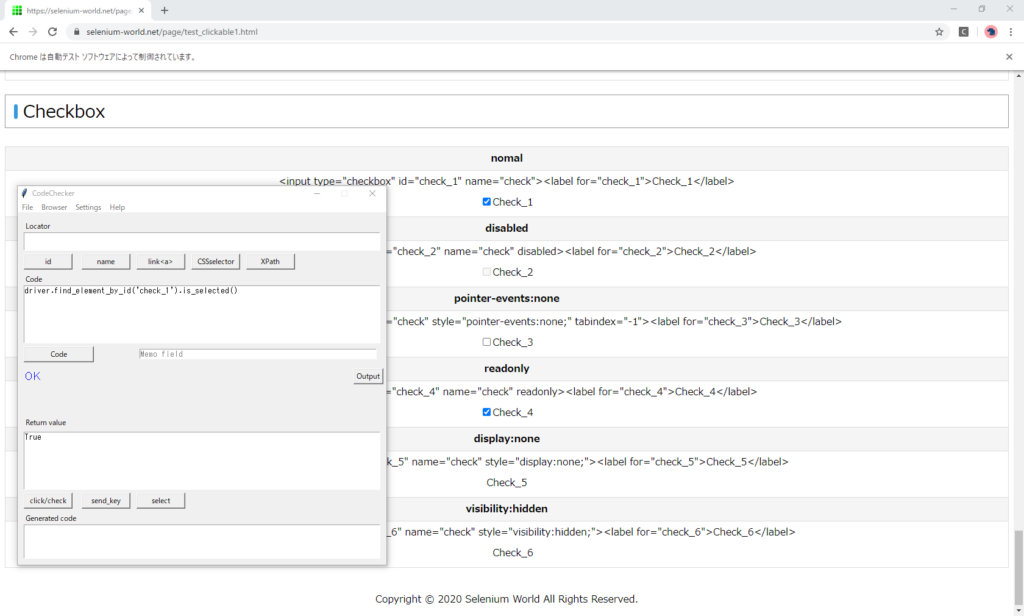
Inactive(disabled)
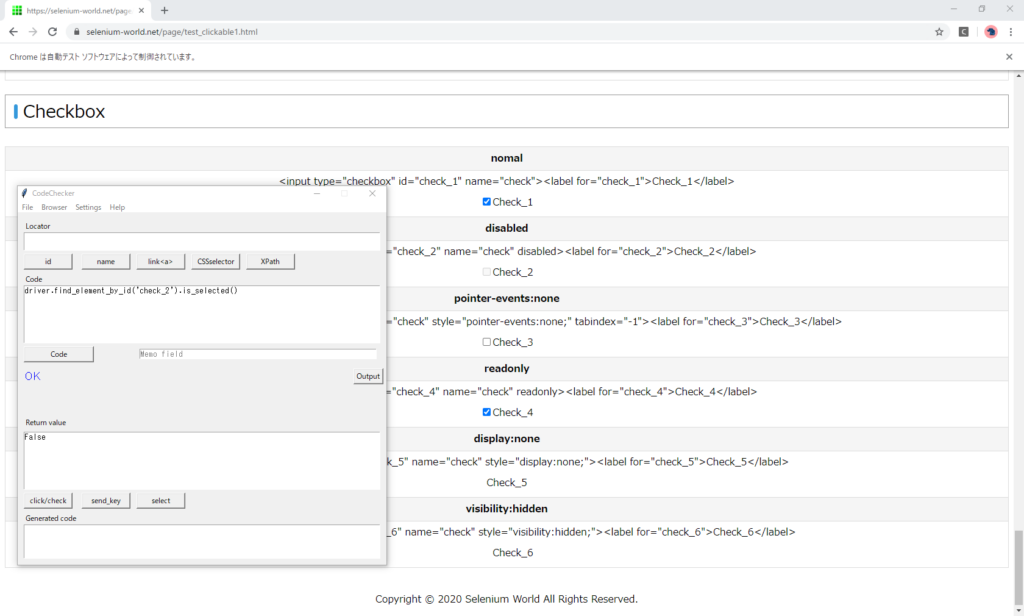
Inactive(pointer-events:none)
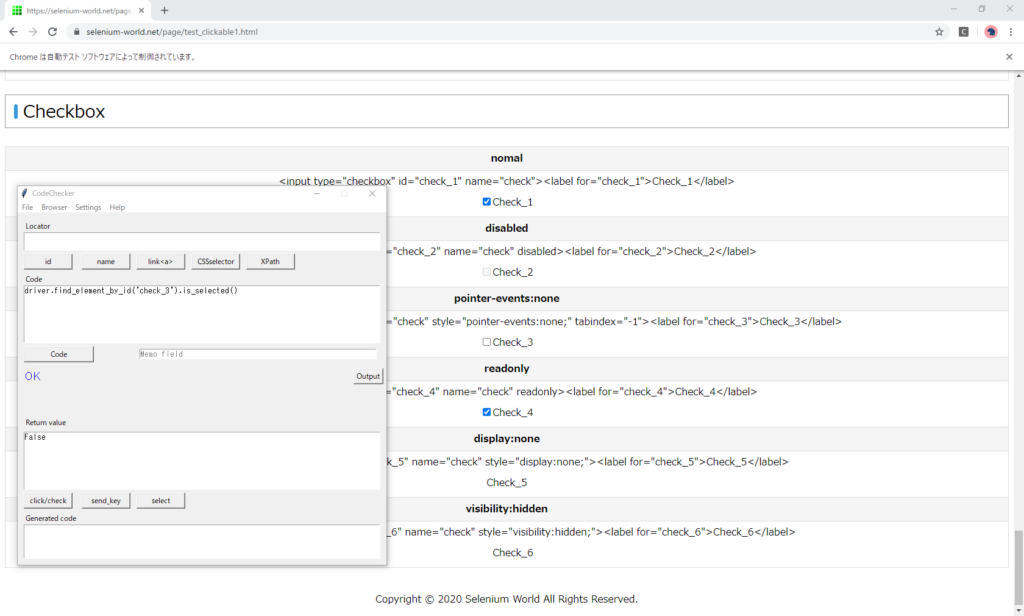
Read-only(readonly)
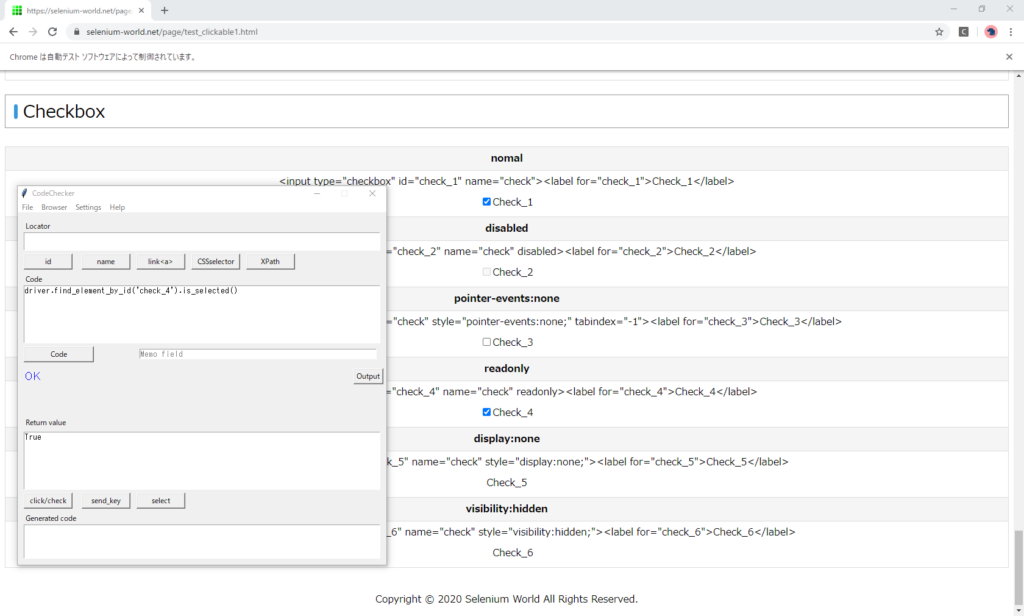
Hidden(display:none)
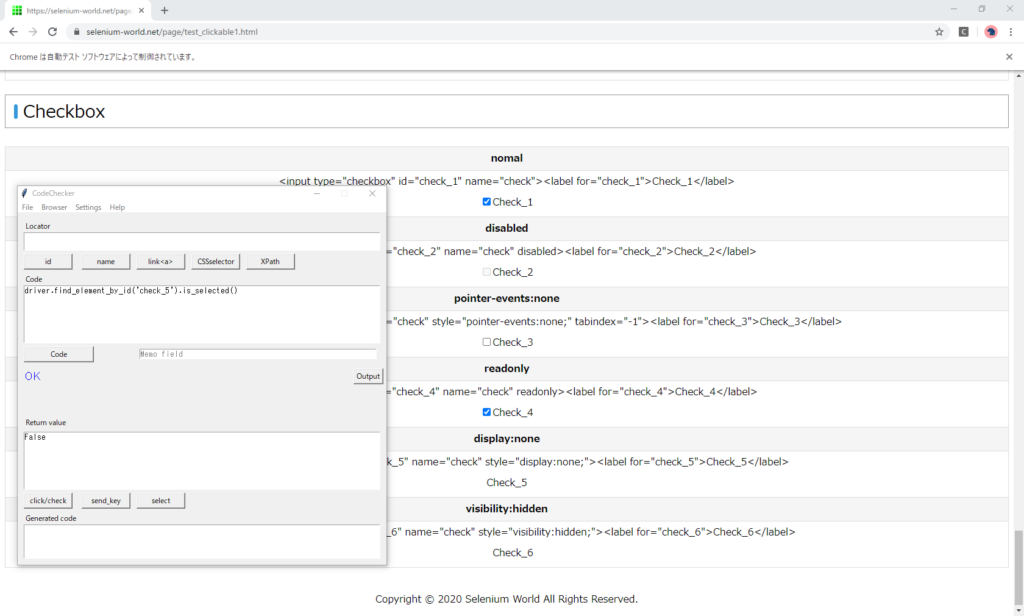
Hidden(visibility:hidden)
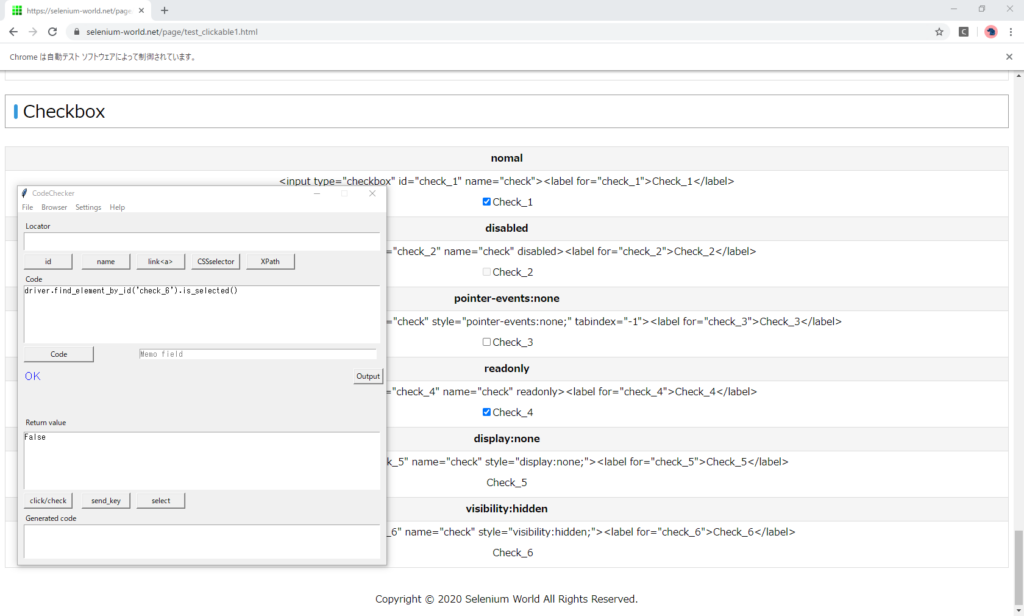
Behavior when label is clicked
The checkbox is specified “label for", so you can check it by clicking label.
Normal
Input the following codes, and press the “Code" button.
driver.find_element_by_xpath('//label[contains(text(),\'Check_1\')]').click()
print(driver.find_element_by_id('check_1').is_selected())
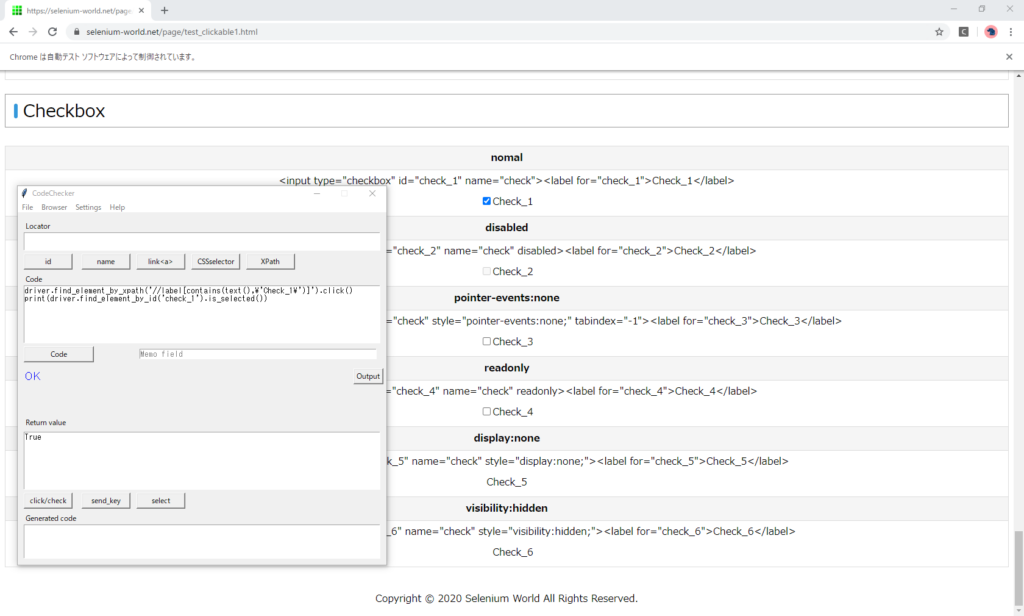
Inactive(disabled)
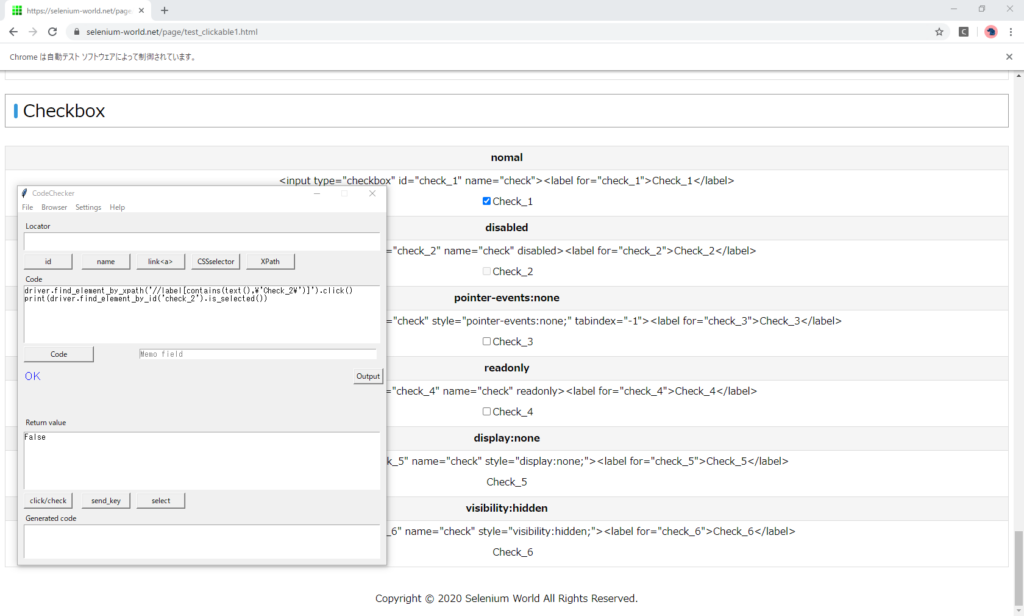
Inactive(pointer-events:none)
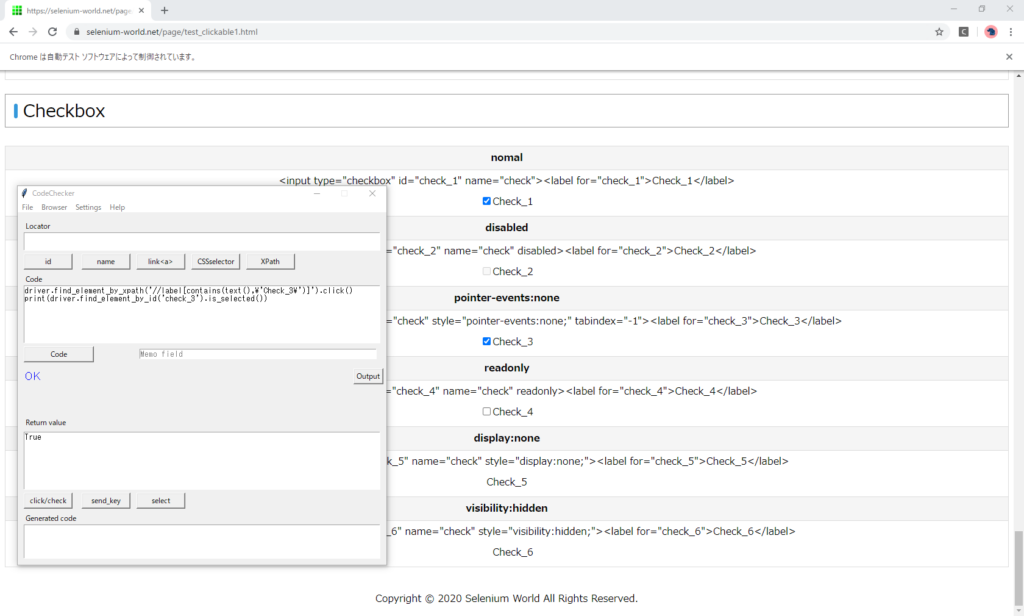
Read-only(readonly)
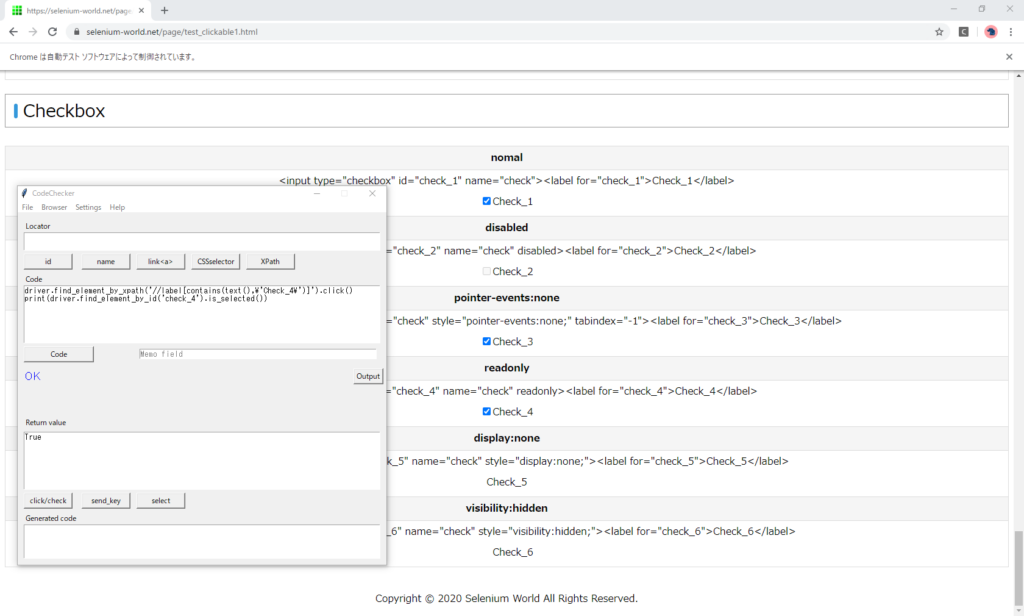
Hidden(display:none)
It is not displayed, but the button is checked by clicking the label.
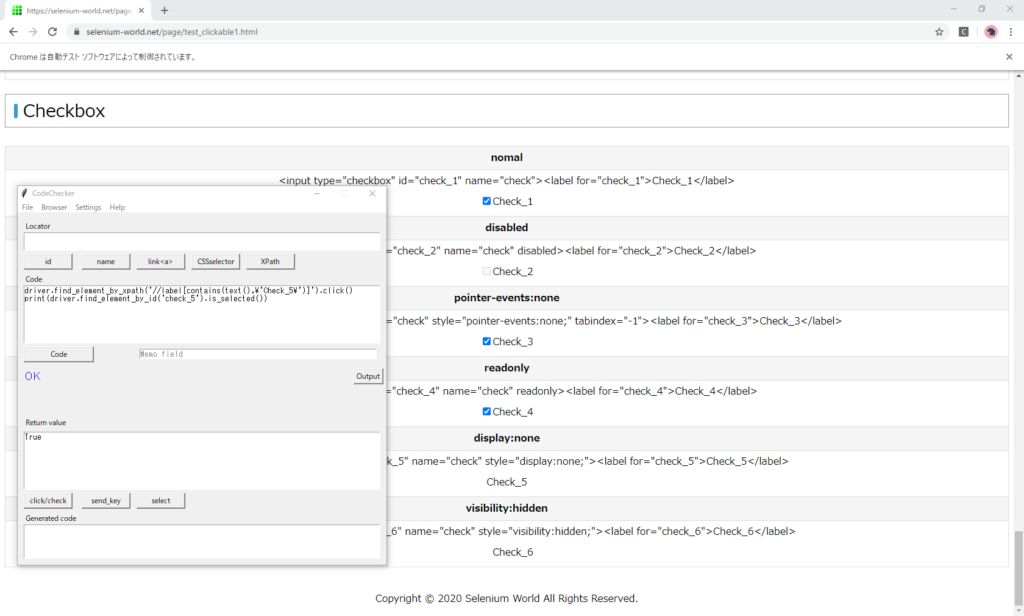
Hidden(visibility:hidden)
It is not displayed, but the button is checked by clicking the label.
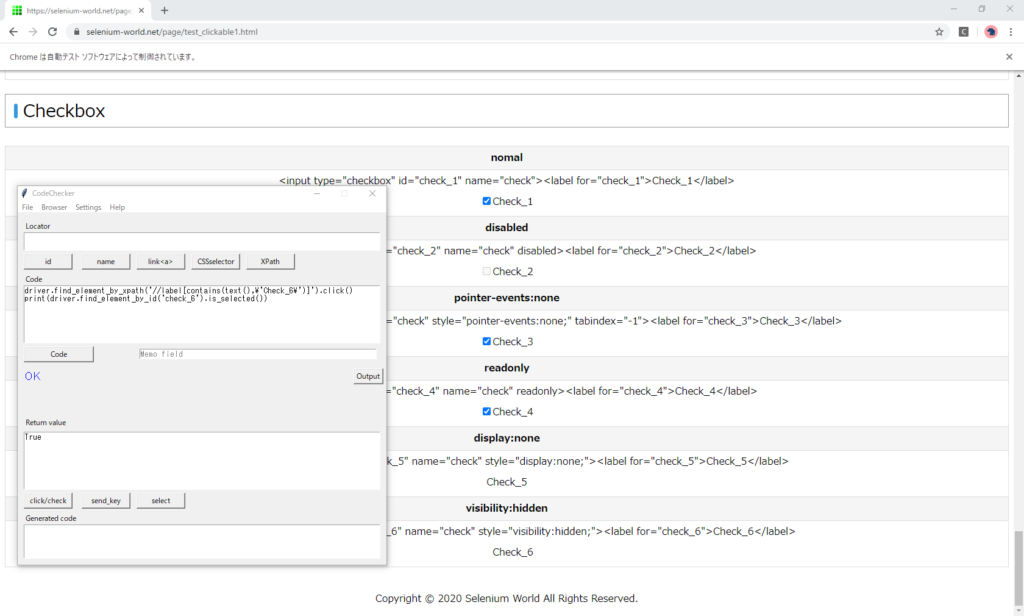
Result
CheckBox | click checkbox | click label | get value |
---|---|---|---|
normal | OK | OK | OK |
disabled | NG | NG | OK |
pointer-events:none | Ex | OK | OK |
readonly | OK | OK | OK |
display:none | Ex | OK | OK |
visibility:hidden | Ex | OK | OK |
Get Attribute
Normal
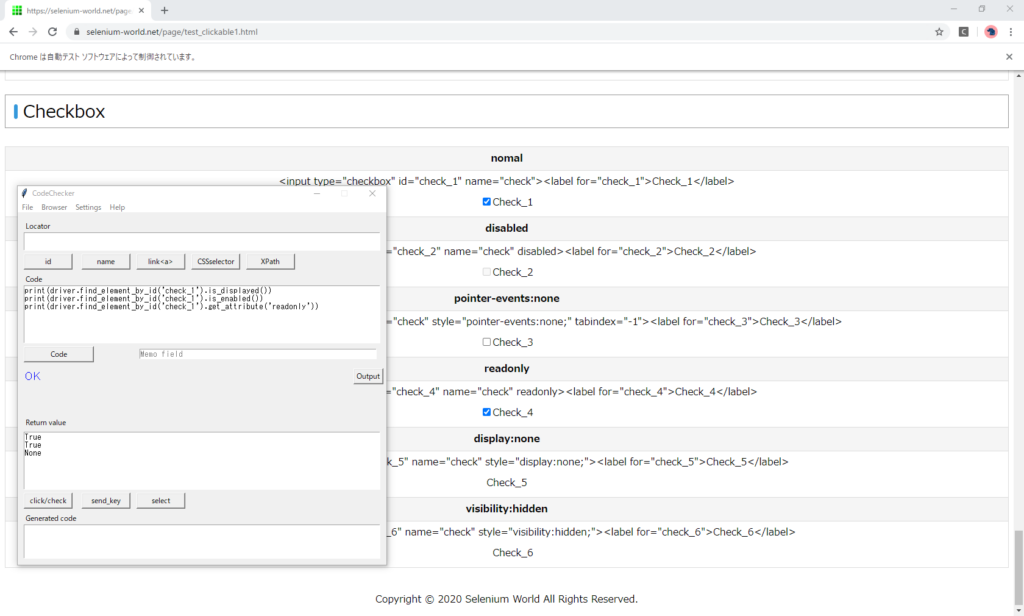
Inactive(disabled)
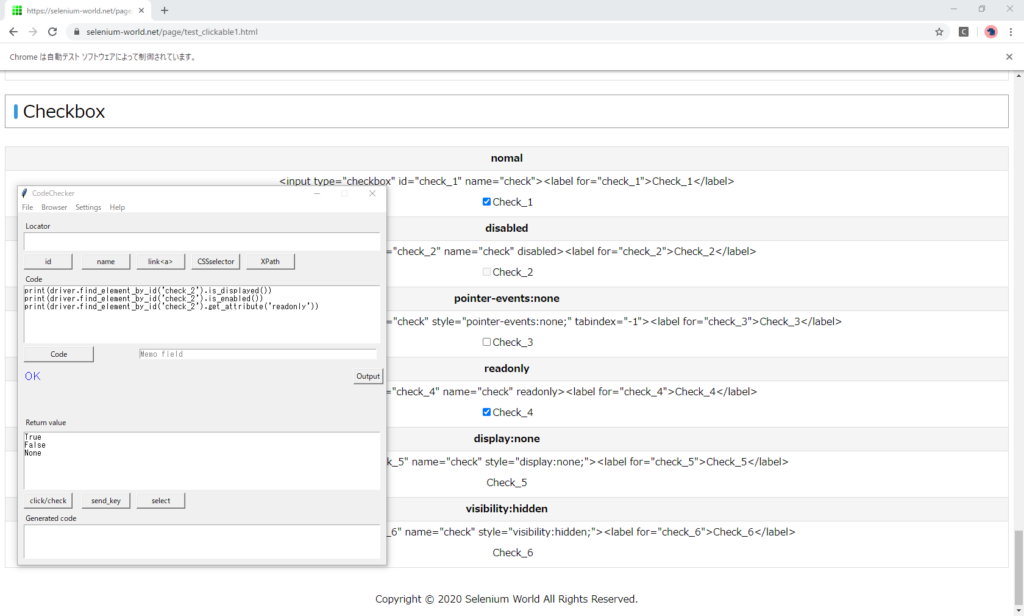
Inactive(pointer-events:none)
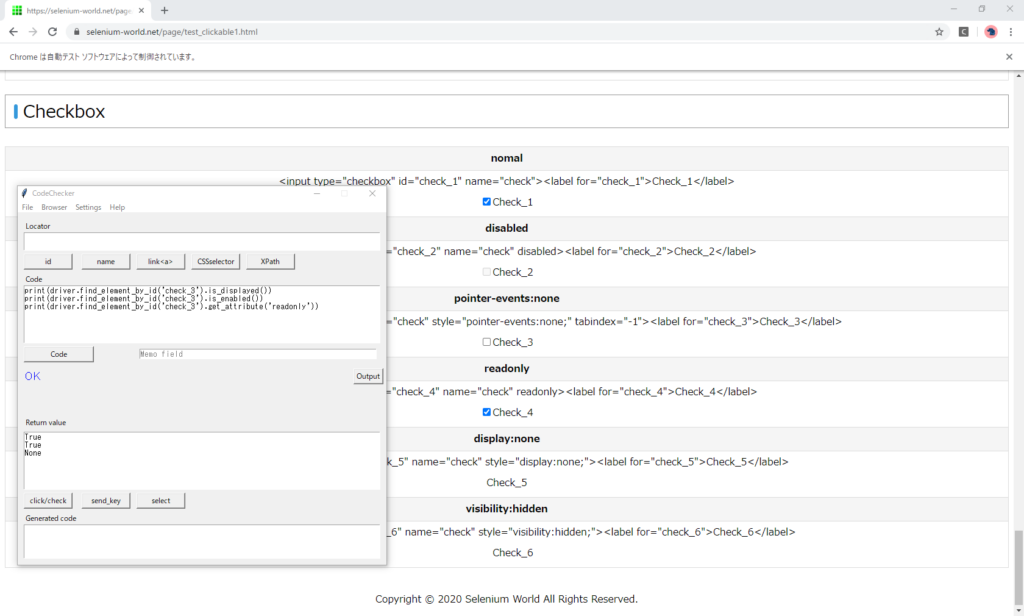
Read-only(readonly)
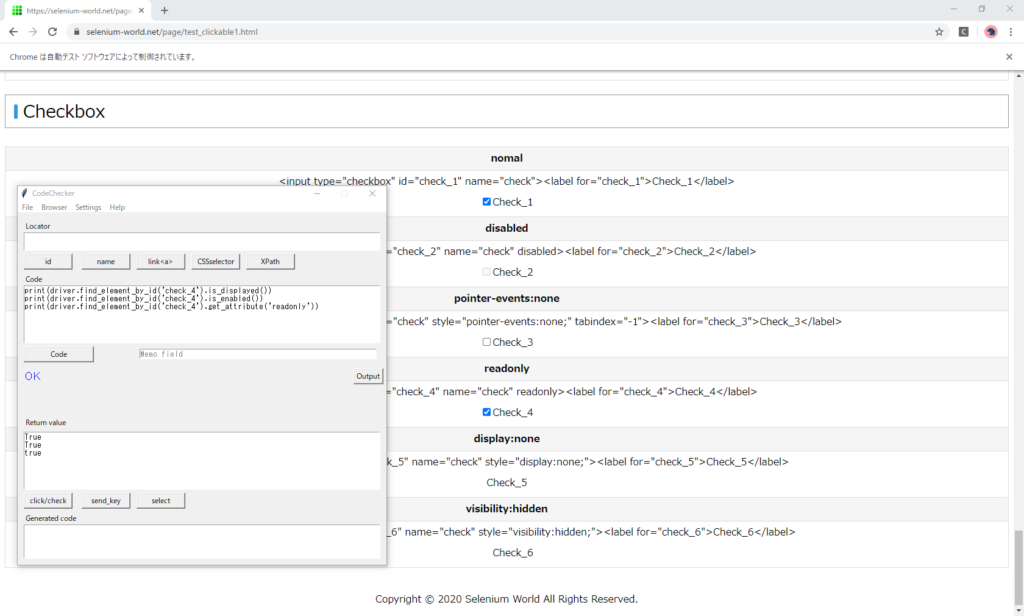
Hidden(display:none)
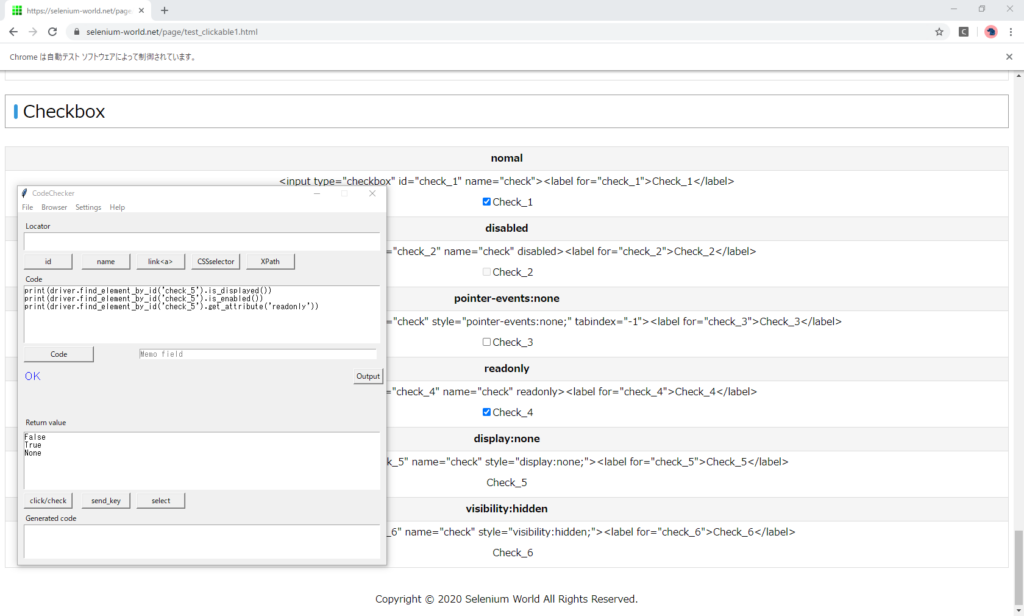
Hidden(visibility:hidden)
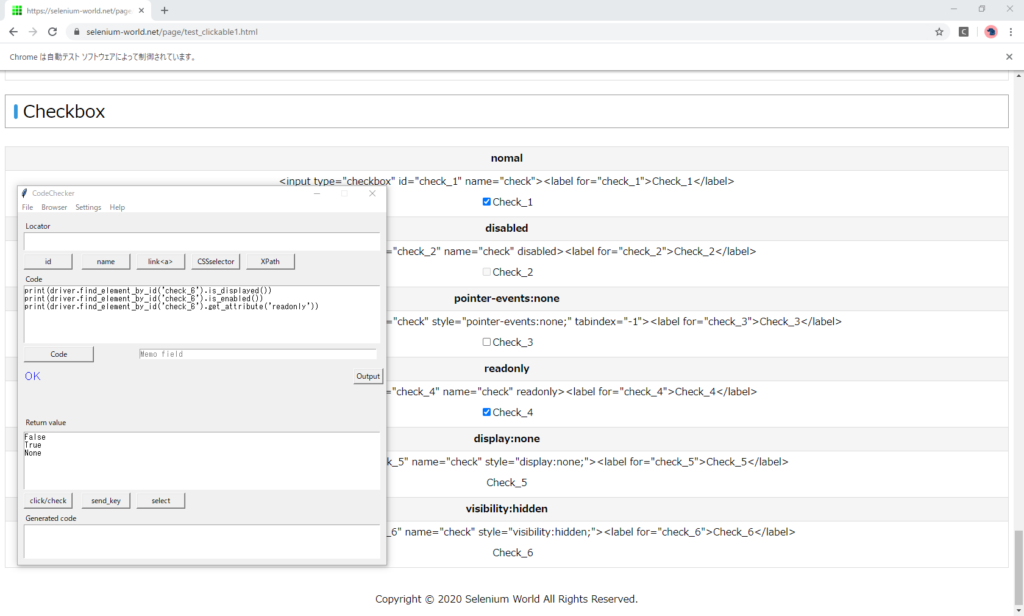
Result
CheckBox | is_displayed() | is_enabled() | readonly |
---|---|---|---|
normal | True | True | None |
disabled | True | False | None |
pointer-events:none | True | True | None |
readonly | True | True | true |
display:none | False | True | None |
visibility:hidden | False | True | None |
Discussion
New Comments
No comments yet. Be the first one!