How Selenium works(elements in each browser)
Using CodeChecker, Let’s look at Selenium’s behavior for controls in each browser.
IE version 11andEdge is 83.
Test page
Inspect for button, link, text, lists, radio, and checkbox.
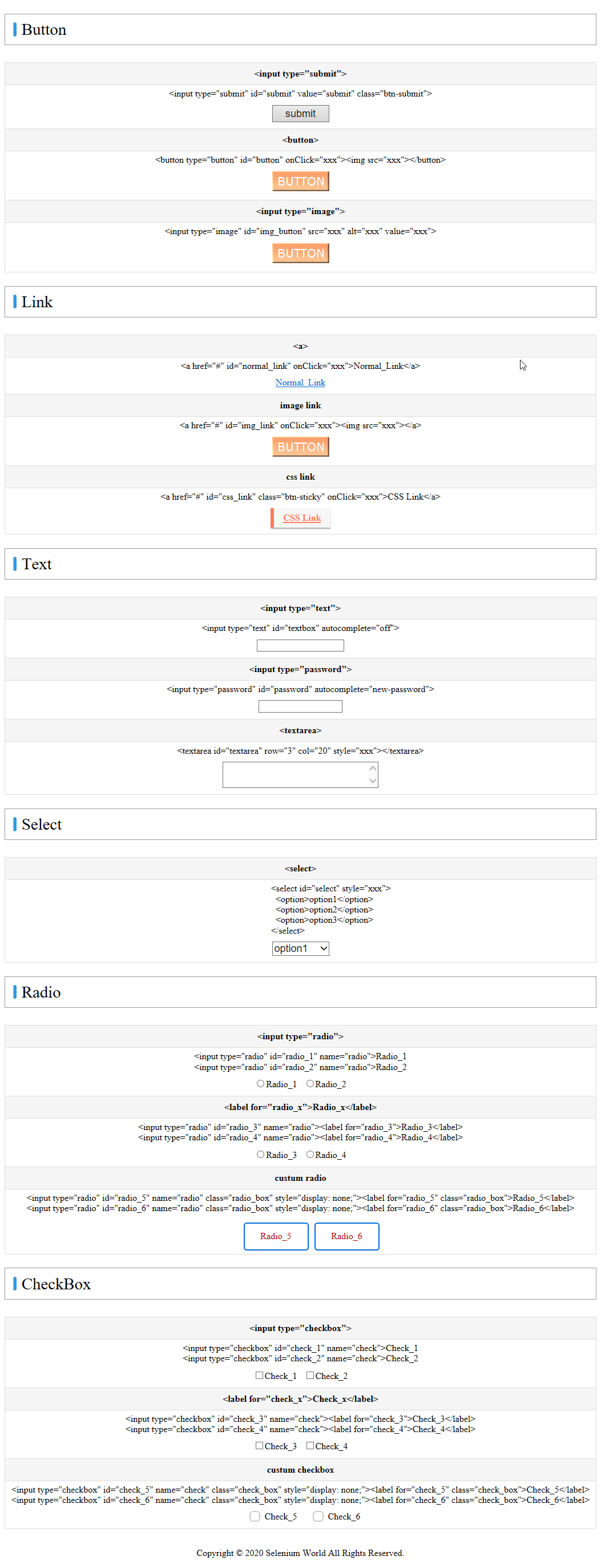
As a variation of controls,
- Button
- <input type="sbumit">
- <input type="image">
- <button>
- Link
- <a href=>
- <a href=><img src="xxx"></a>
- CSS_Custom
- Text
- <input type="text">
- <input type="password">
- <textarea>
- Radio / CheckBox
- normal
- <label for="id">
- CSS_Custom
Operating procedure
Get the element by specifying the element’s “id" in CodeChecker.
1.Start CodeChecker
Display the test page in the launched browser.
https:selenium-world.net/page/test_cntl_click1.html
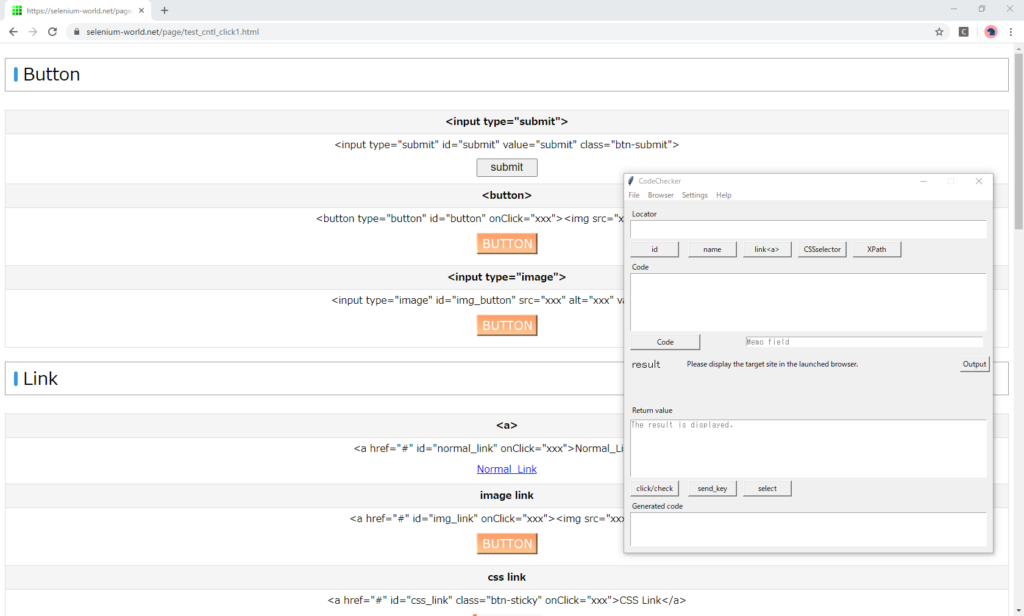
Get the element
In the locator field, enter the id of the element and press the “id" button.
If successful, it will appear “OK", the tag name and innerHTML of the element ,and Python code to retrieve the element.
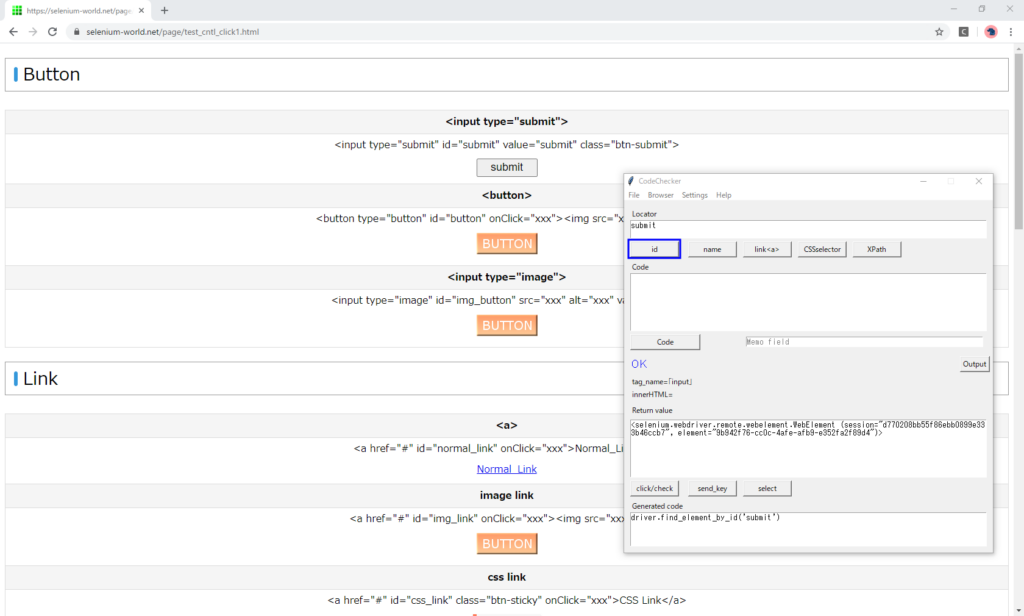
Operate control
After getting the element, press the button for the operation.
If successful, the code executed is displayed.
Click
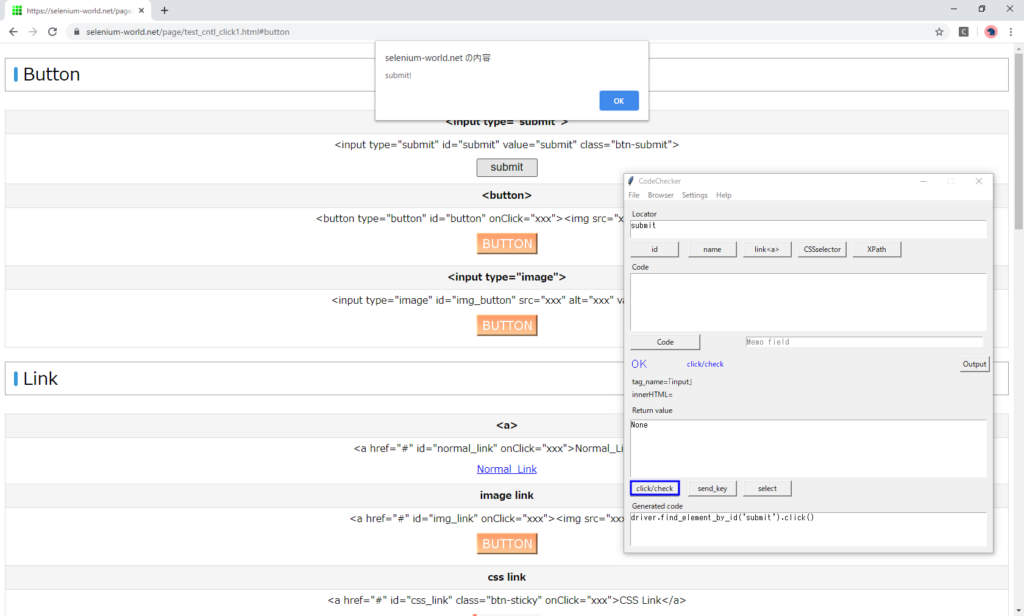
Send_key
“hoge" is entered.
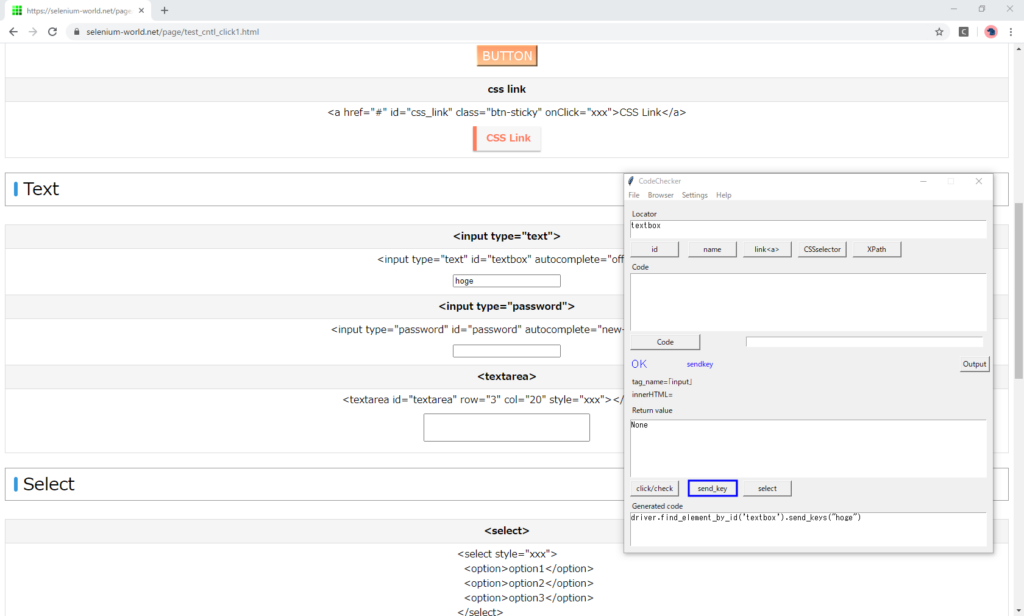
Select
The second item is selected.
Usually, the first item is selected by default, so the second item will be selected .
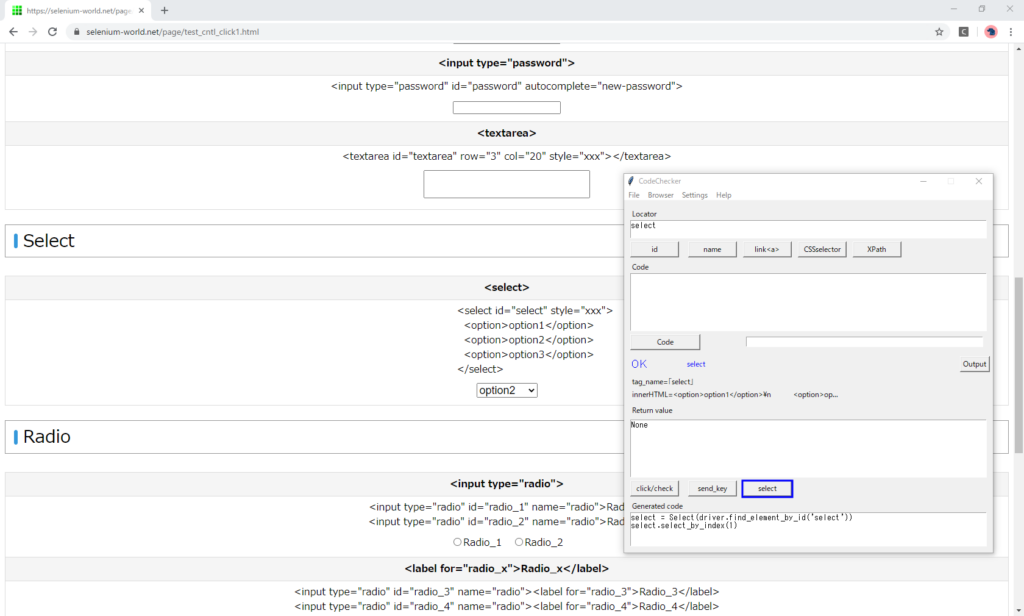
Code execute
If you want to run python code, type it in the code field and press the “Code" button.
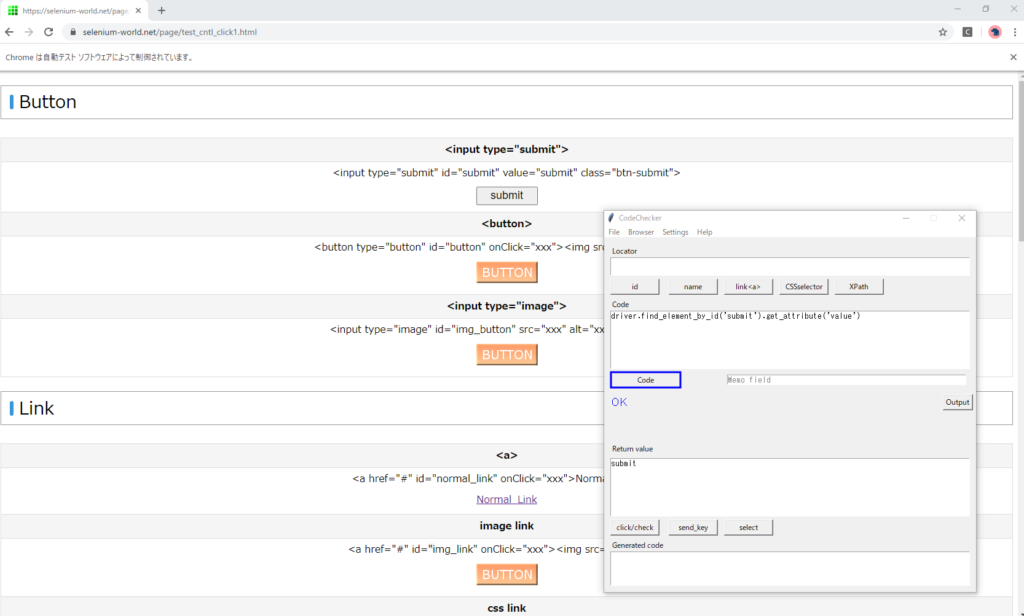
In a development editor, you will need to restart the script every time you enter code. You’ll need to launch a new Selenium and to display the target page.
CodeChecker allows you to run code as many times as you like while keeping the target screen open in your browser.
Switch browser
Open the setting window.
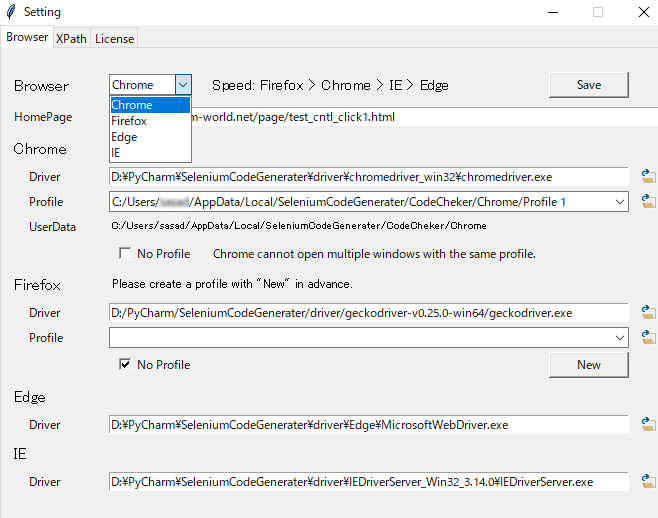
Perform operation
Google Chrome
Button
First, enter the id of the element in the locator field and press the “id" button.
Next, press the “click" button.
If successful, the executed code is displayed.
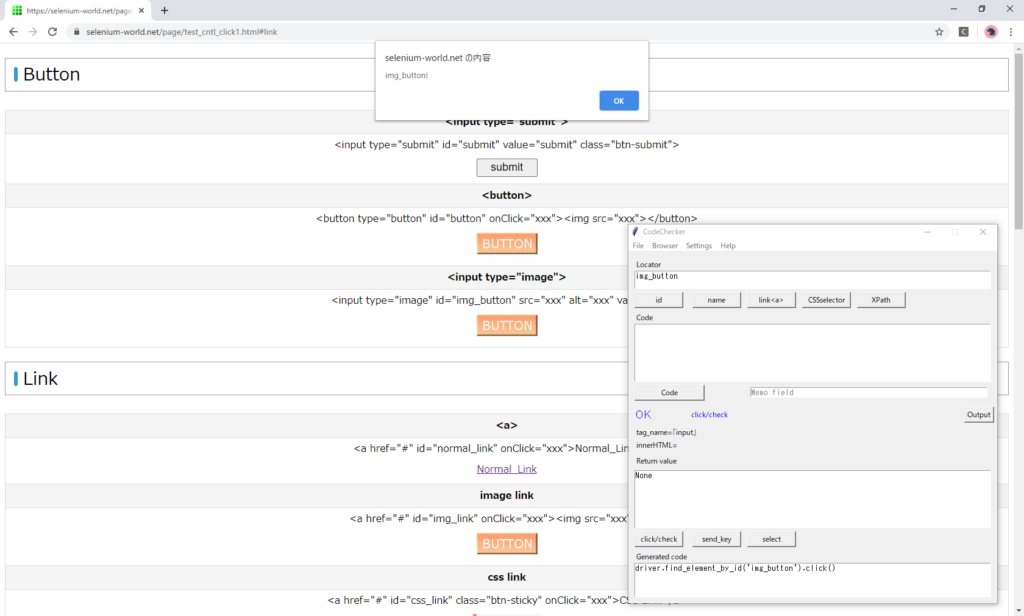
Link
After getting the element, press the “click" button.
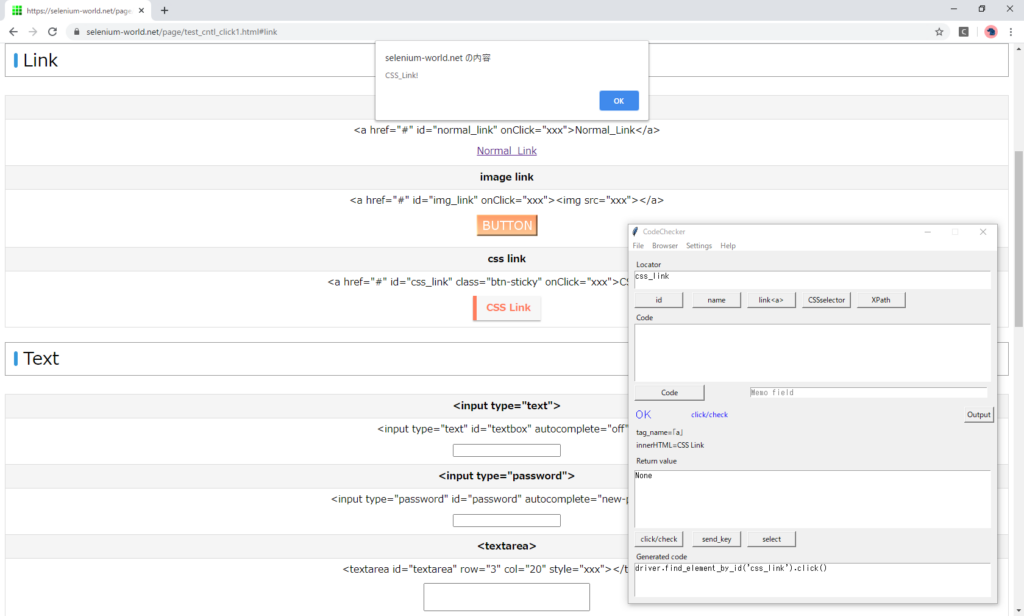
Text
After getting the element, press the “send_key" button. “hoge" is entered in the specified element.
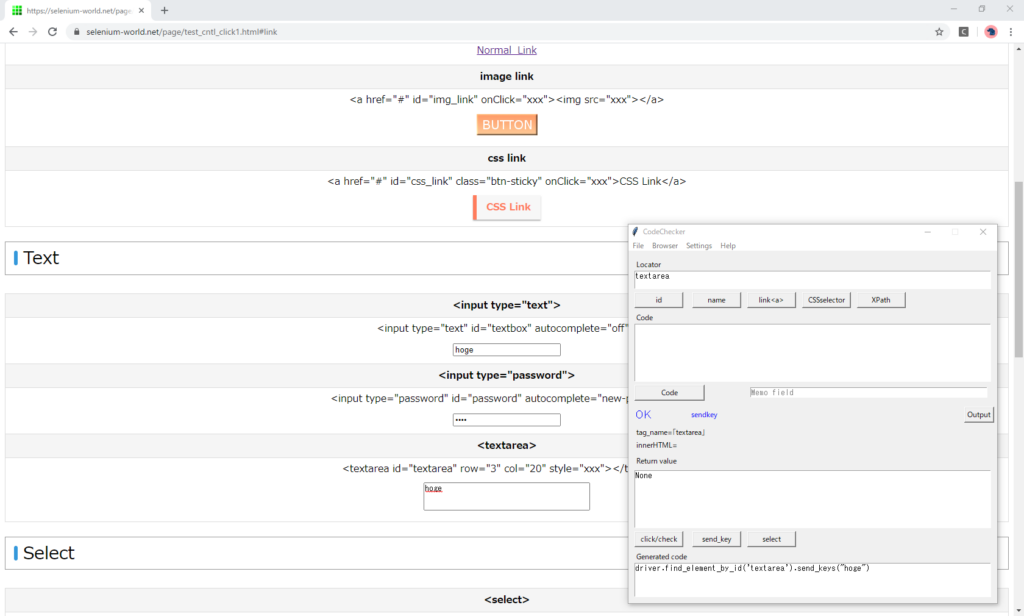
Get the text value.
Type “get_attribute('value’)" in the code field and press the “Code" button.
“hoge" is returned.
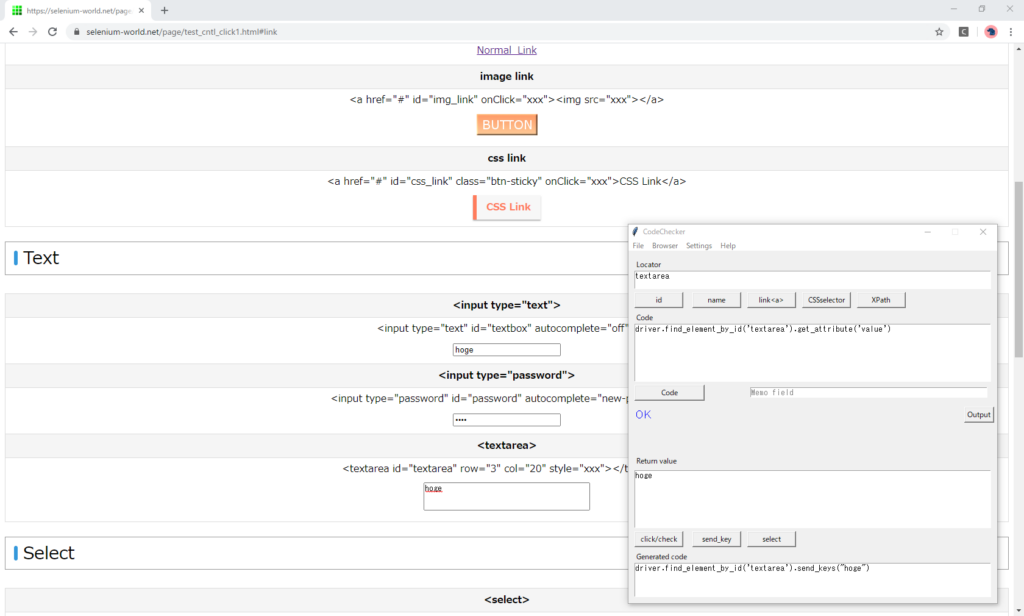
On the screen, the password character is hidden, but the value is returned.
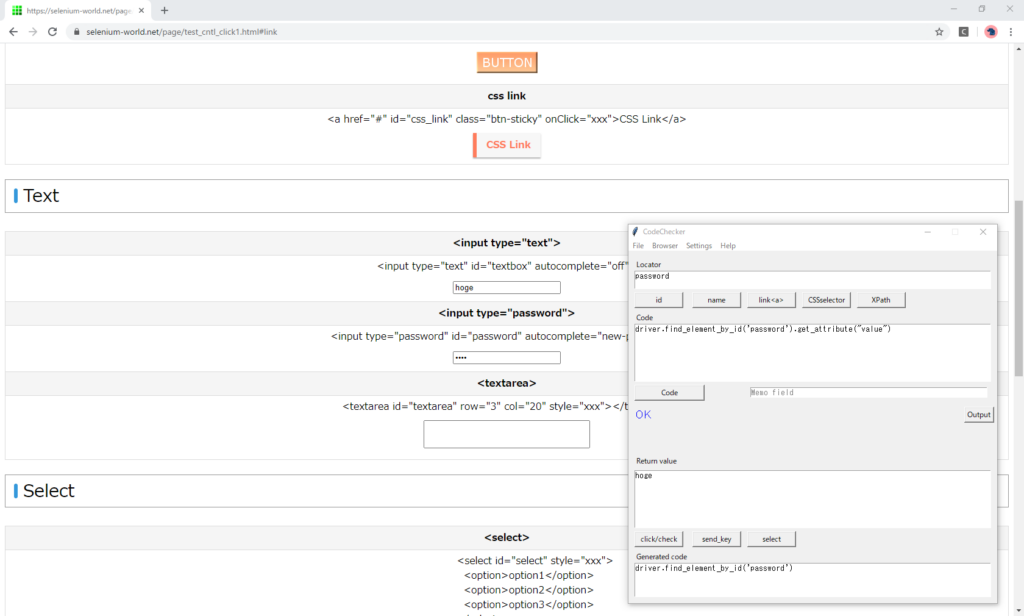
Select
Select item
Press the “select" button. The second item is selected.
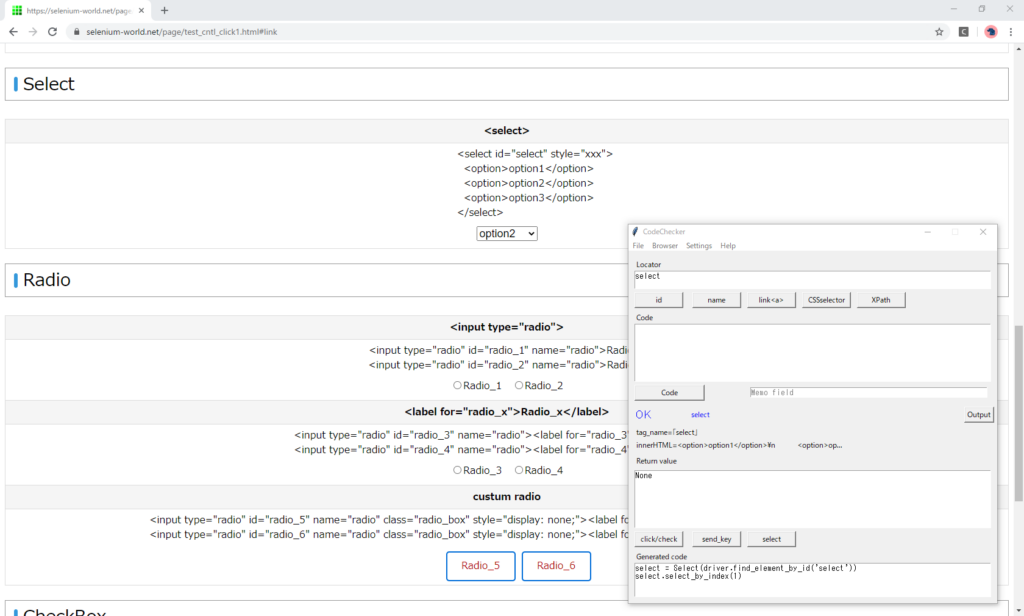
Get the selected item
Input code
select.first_selected_option()
Exception occurred.
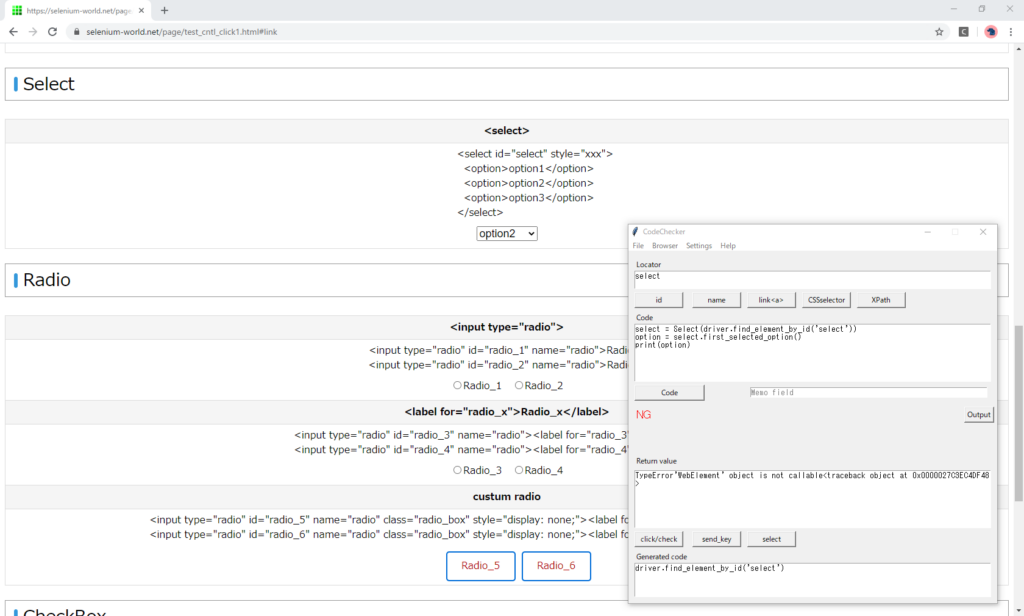
Message
TypeError'WebElement' object is not callable<traceback object at 0x000001C12191C048>
“first_selected_option" is not Python method, but property.
Remove the “()".
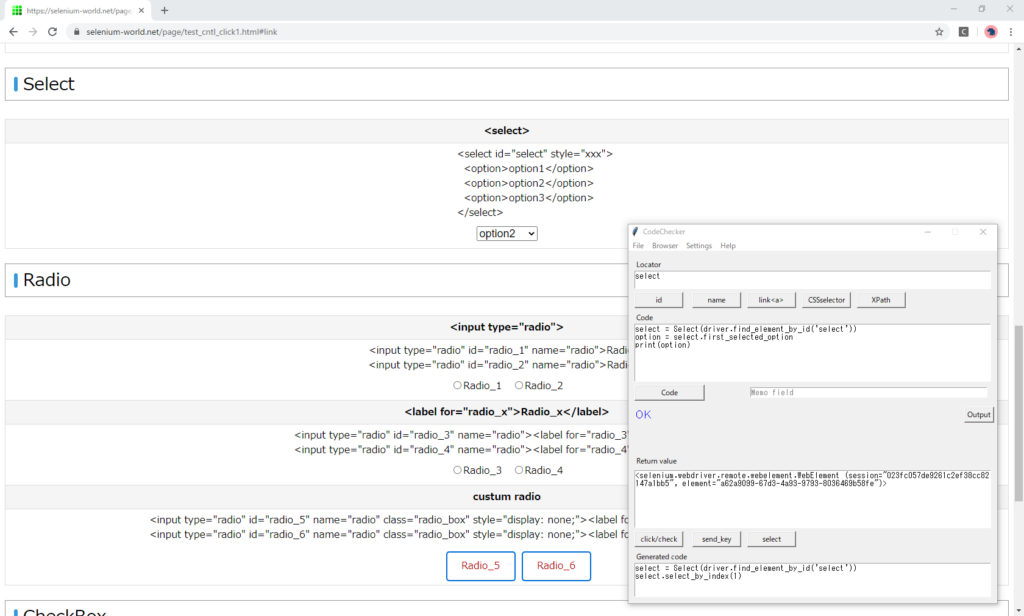
“First_selected_option" returns a “WebElement" object. Then specify the “text" property.
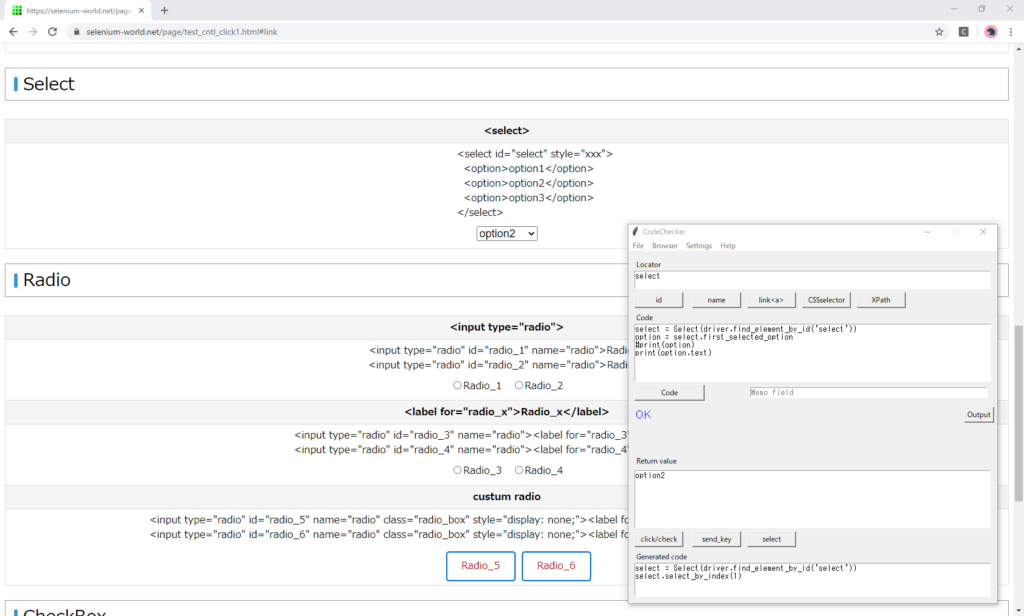
Using CodeChecker, if an exception occurs, the Selenium instance is retained and the browser screen does not close.
You can try and run the fix code as many times as you like.
Radio
Press the “click/check" button.
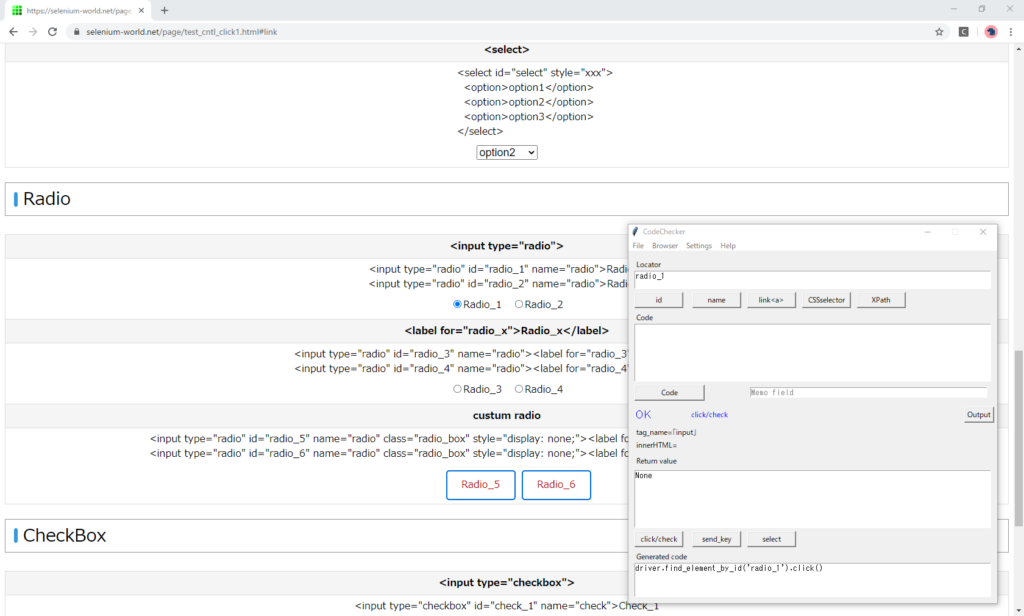
Get a selection status of radio.
Type “is_selected()" in the code field and press the “Code" button.
“True" is returned.
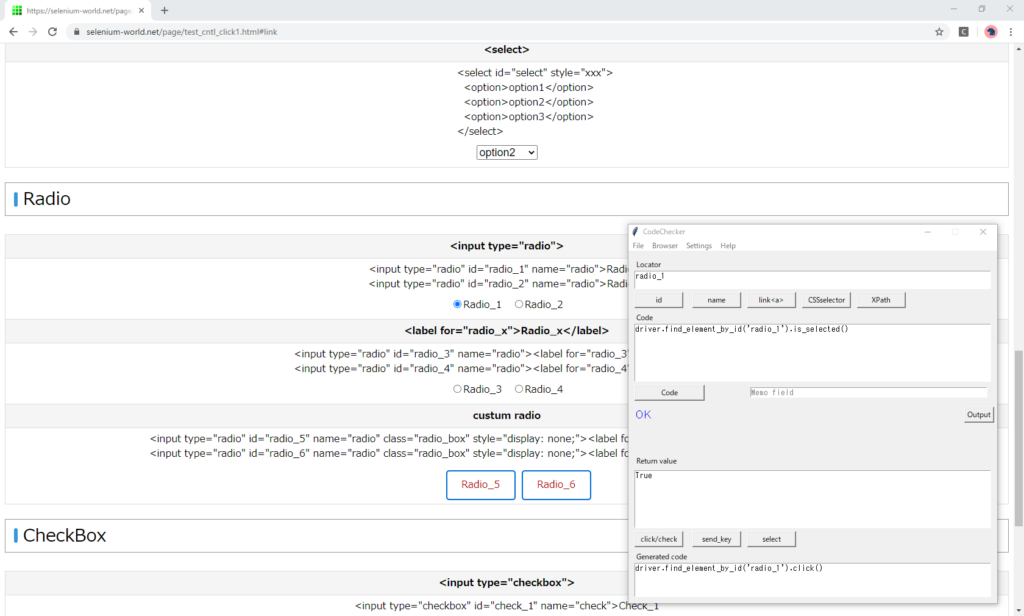
Custom Radio
After getting the element, press the “click" button.
Exception occurred.
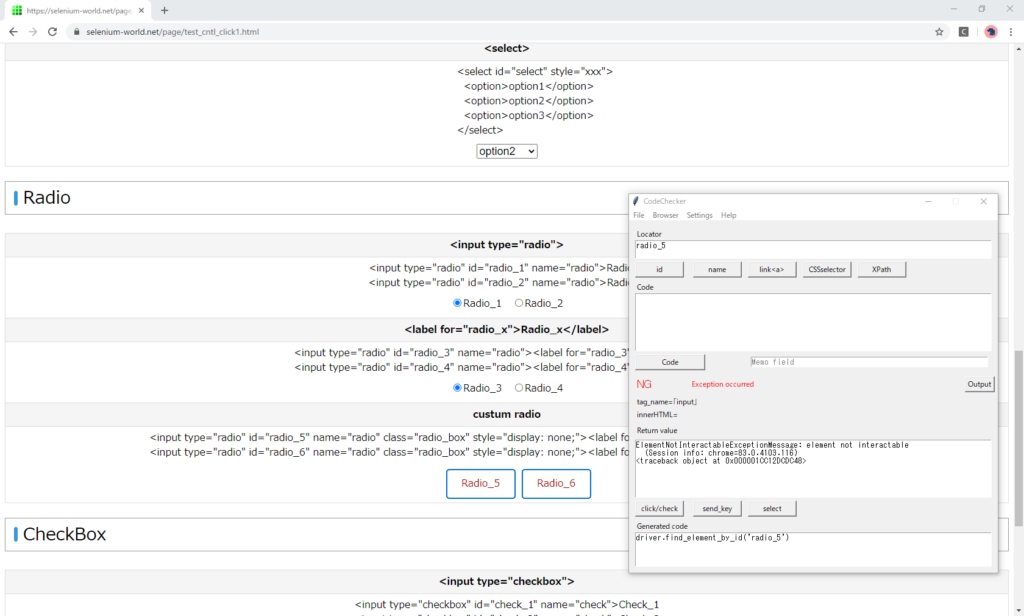
Message
ElementNotInteractableExceptionMessage: element not interactable
(Session info: chrome=83.0.4103.116)
<traceback object at 0x000001CC12E07248>
HTML
<div>
<input type="radio" id="radio_5" name="radio" class="radio_box" style="display: none;">
<label for="radio_5" class="radio_box">Radio_5</label>
</div>
This radio is hidden by “display:none" and the button is created by the stylesheet of label.
Get the XPath
Get the XPath of the label element by XPathGetter.
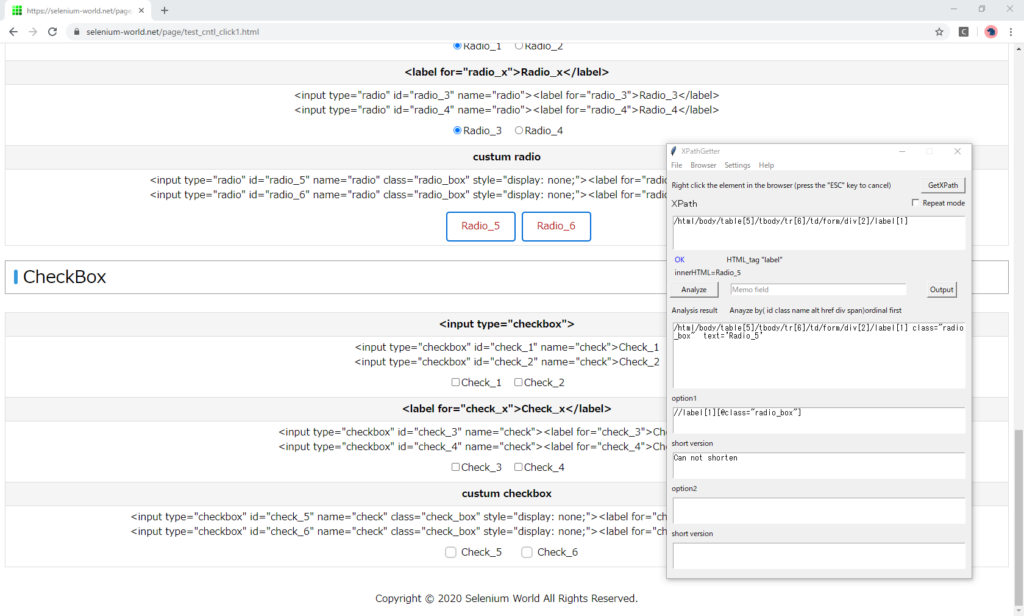
In the locator field, enter the XPath of the label element and press the “XPath" button.
After getting the element, press the “click" button.
Radio_5 is selected.
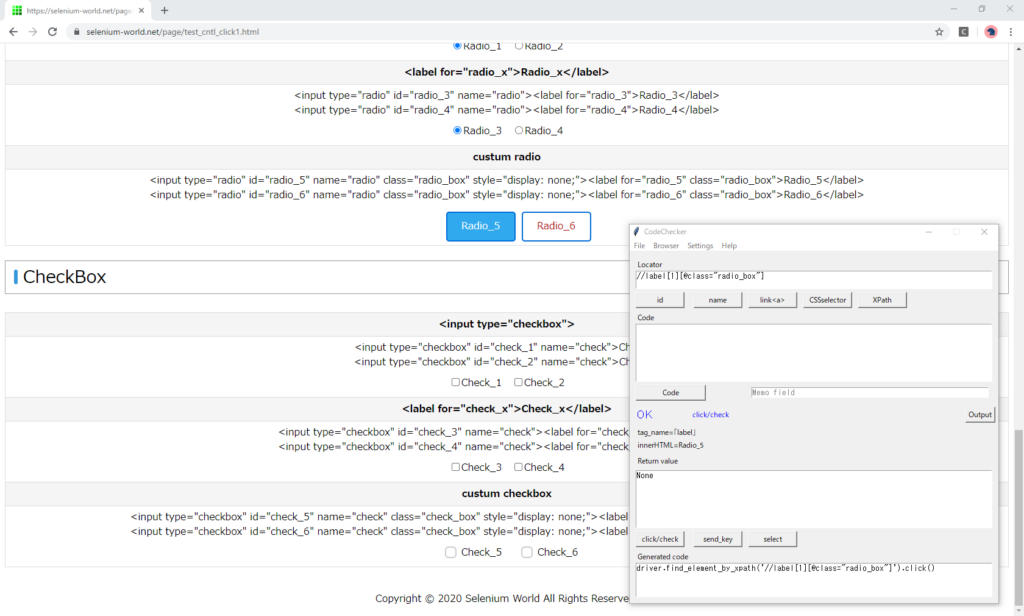
Get a selection status
Get the selected state of the label element with “is_selected()".
“False" is returned.
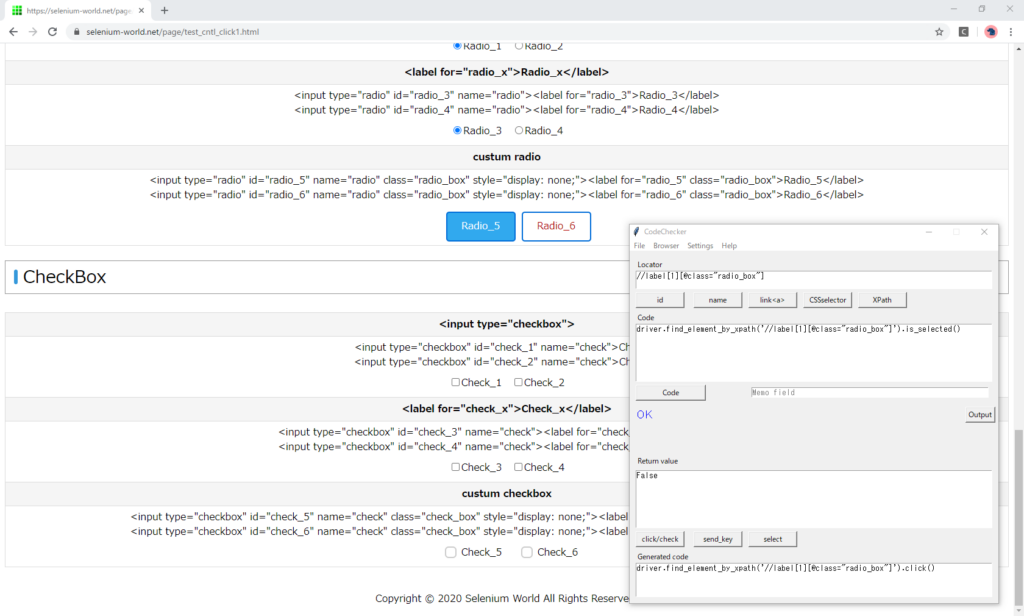
The label object has no a selected state.
Get the selected state of the radio element.
“True" is returned.
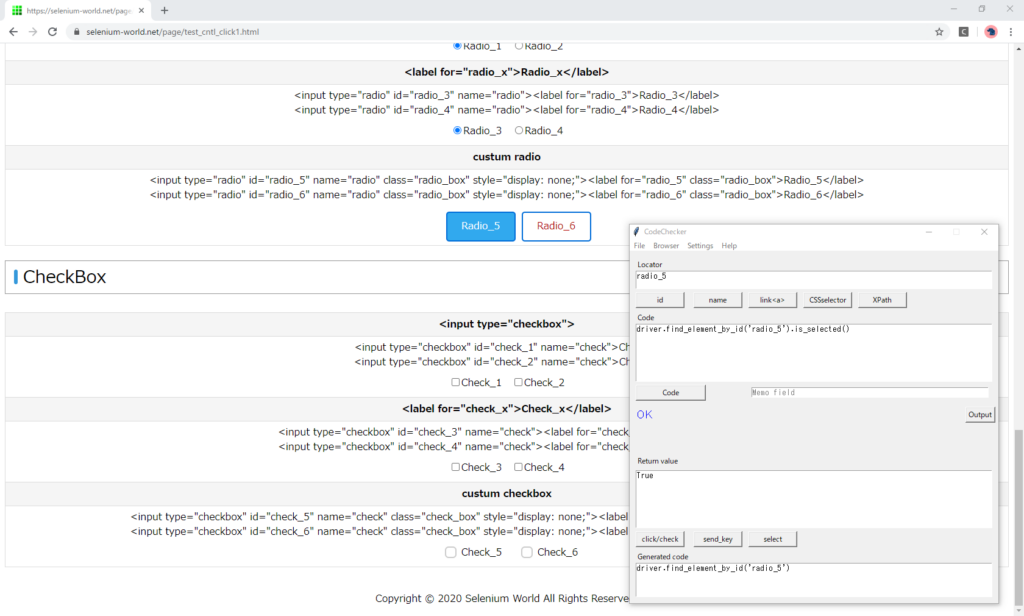
Conclusion
From the above, the Radio button
- If “display:none", Selenium cannot click.
- To select, click the element displaying the button. (label in the case above)
- Selection state must be retrieved from radio object.
After this, let’s see how it works in other browsers.
CheckBox
Press the “click/check" button.
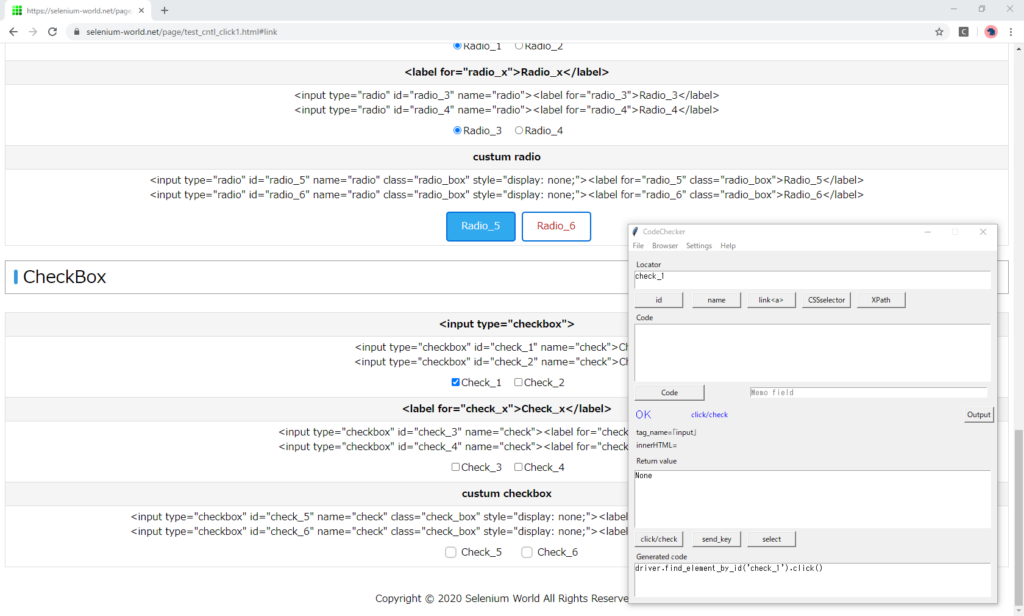
Get a selection status.
Type “is_selected()" in the code field and press the “Code" button.
“True" is returned.
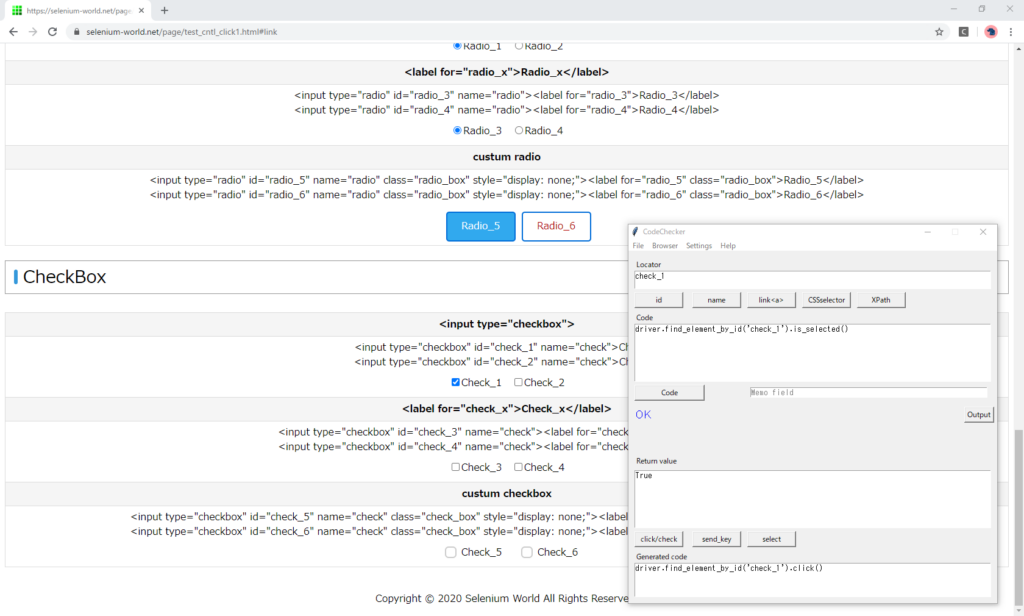
Custom CheckBox
After getting the element, press the “click" button.
Exception occurred like the Radio.
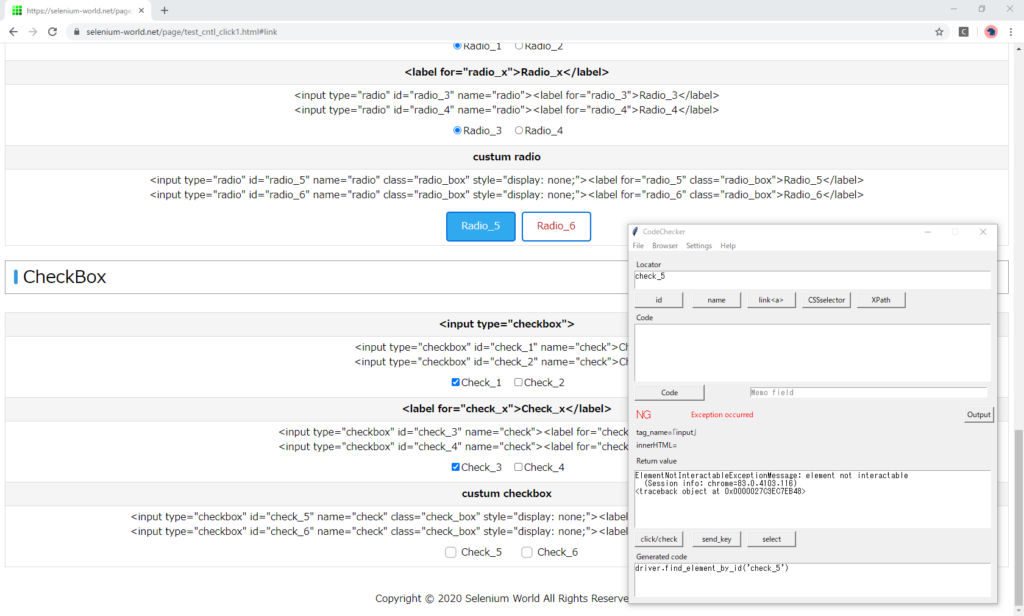
ElementNotInteractableExceptionMessage: element not interactable
(Session info: chrome=83.0.4103.116)
<traceback object at 0x000001CC12E07C88>
Like the Radio, this checkbox is hidden by “display:none" and the button is displayed in the stylesheet of label.
<div>
<input type="checkbox" id="check_5" name="check" class="check_box" style="display: none;">
<label for="check_5" class="check_box">Check_5</label>
</div>
You can select with label clicking.
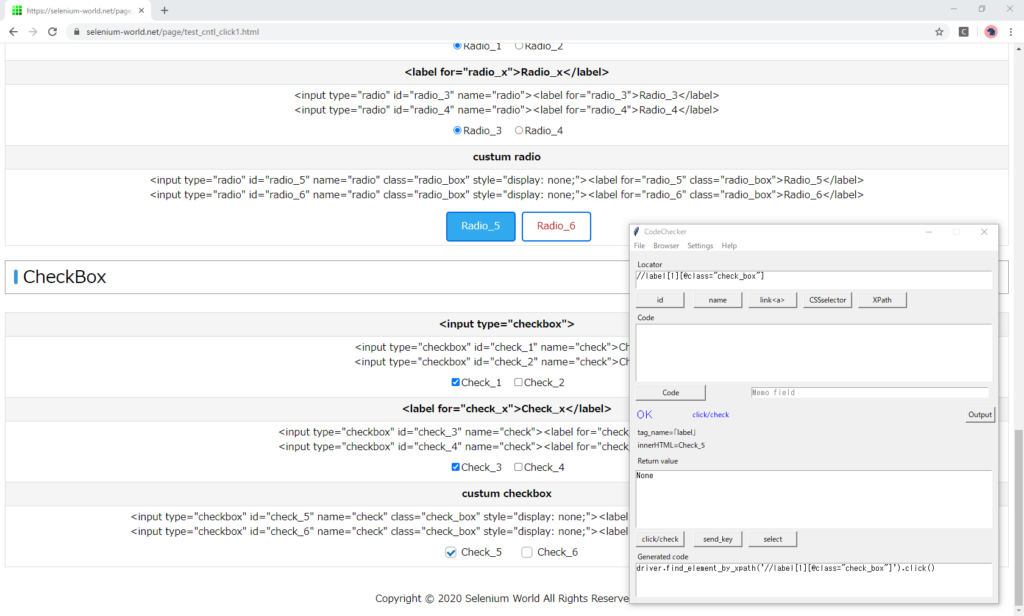
The label object has no a selected state.
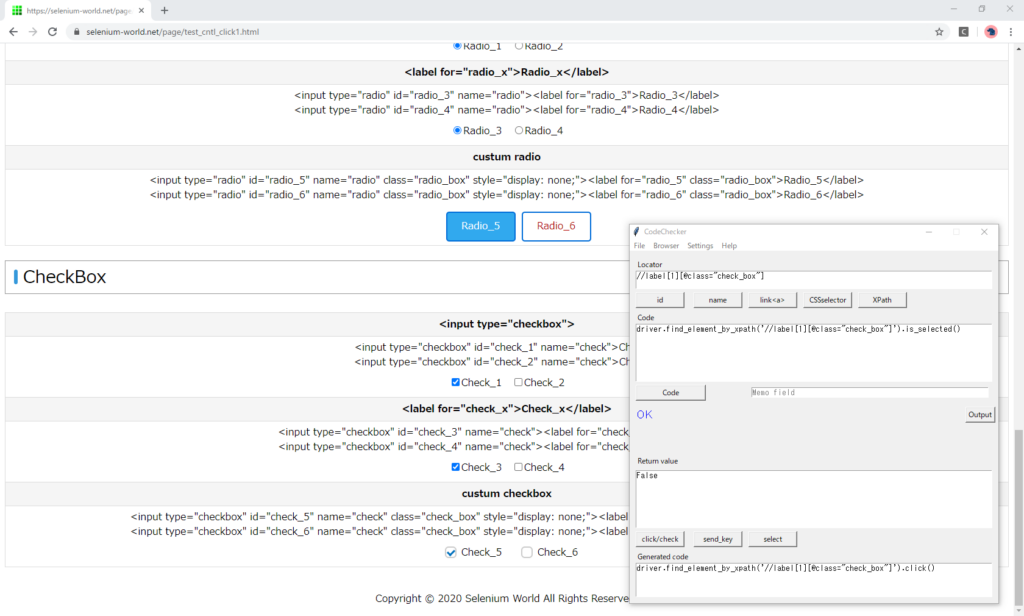
Get the selected state of the checkbox element.
“True" is returned.
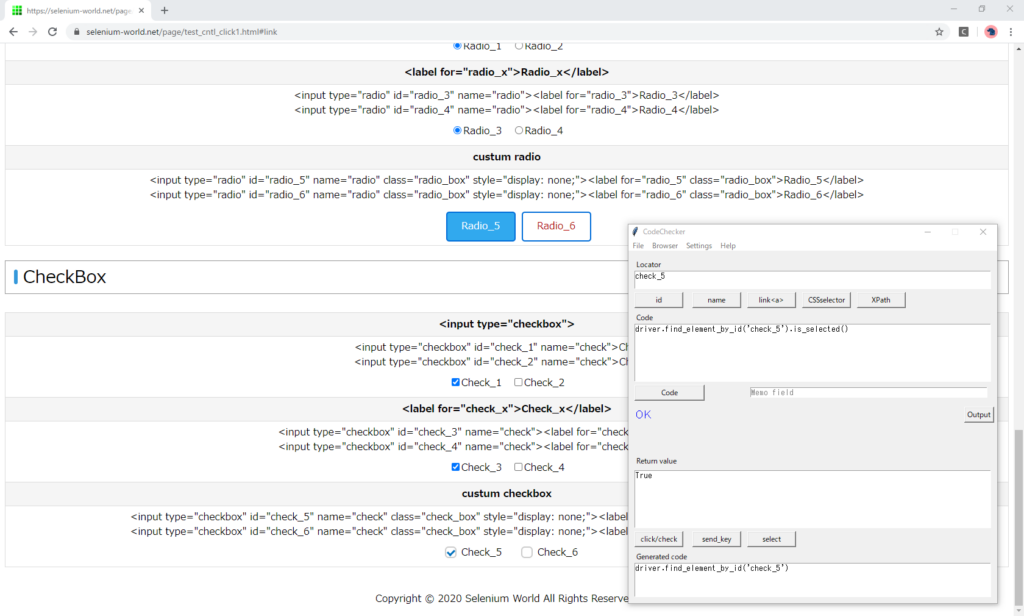
Firefox
Button, Link
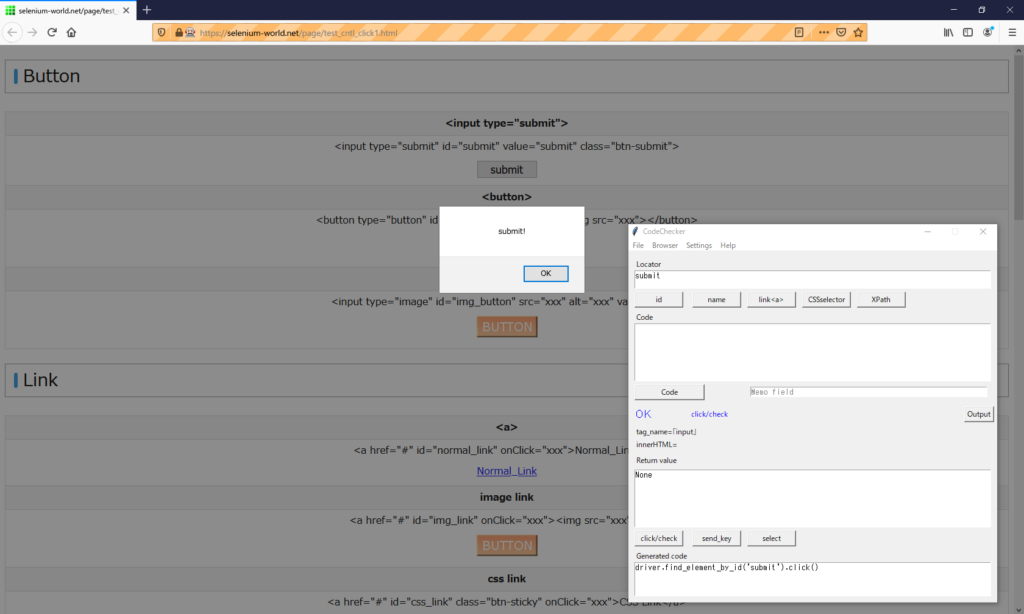
Text
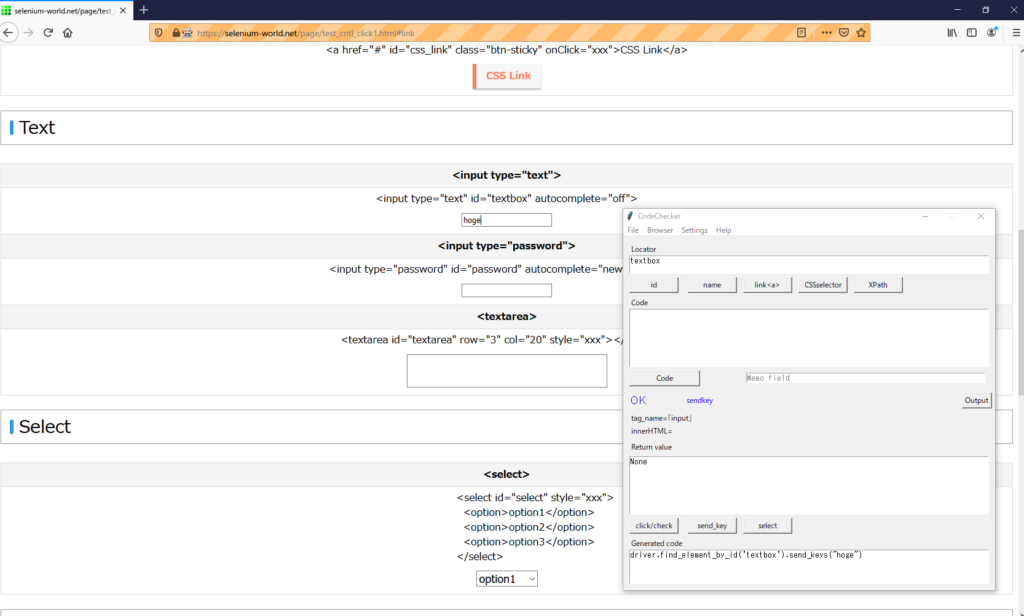
Select
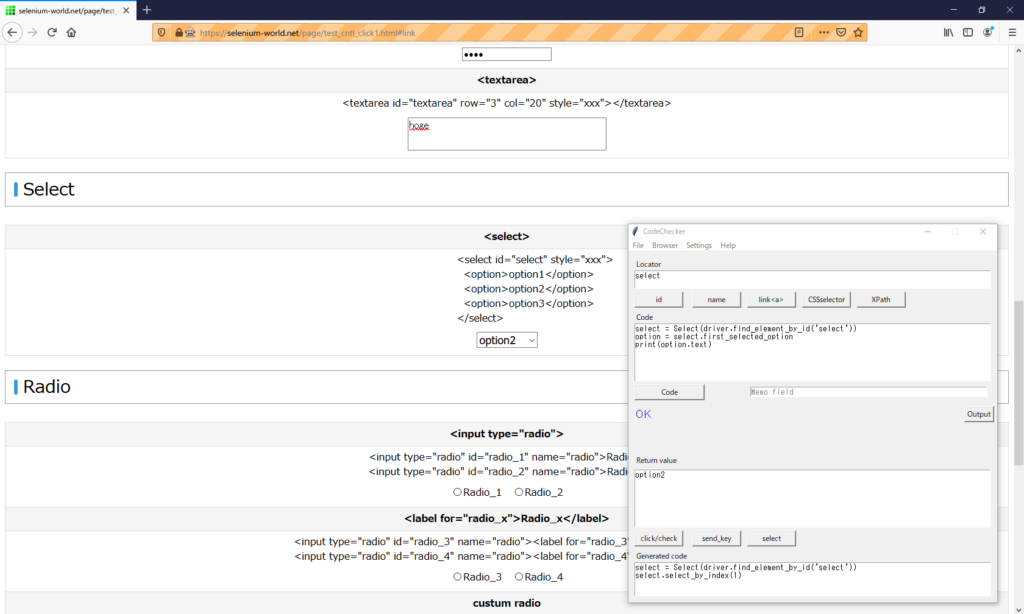
Radio
Exception occurred on Radio_5.
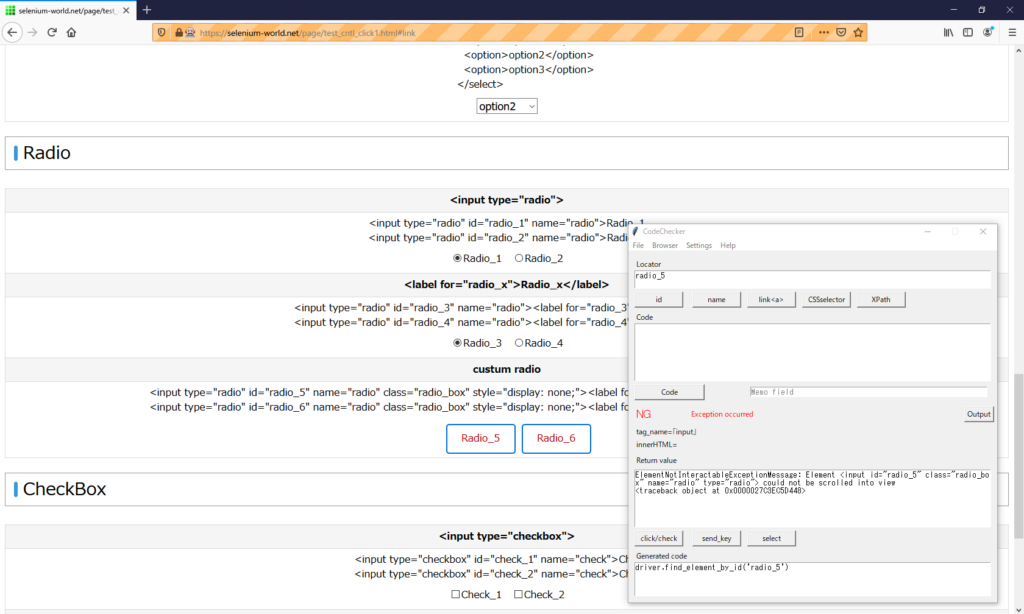
The message is different from Chrome.
ElementNotInteractableExceptionMessage: Element <input id="check_5" class="check_box" name="check" type="checkbox"> could not be scrolled into view
<traceback object at 0x000001ADAE2305C8>
To select, click the label element.
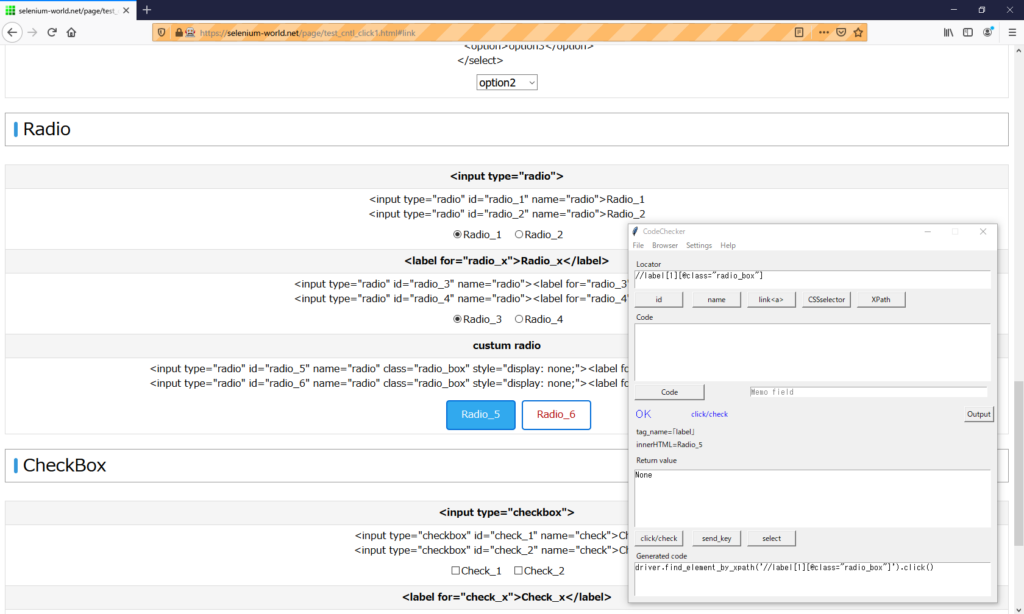
Get the selected state of the radio element.
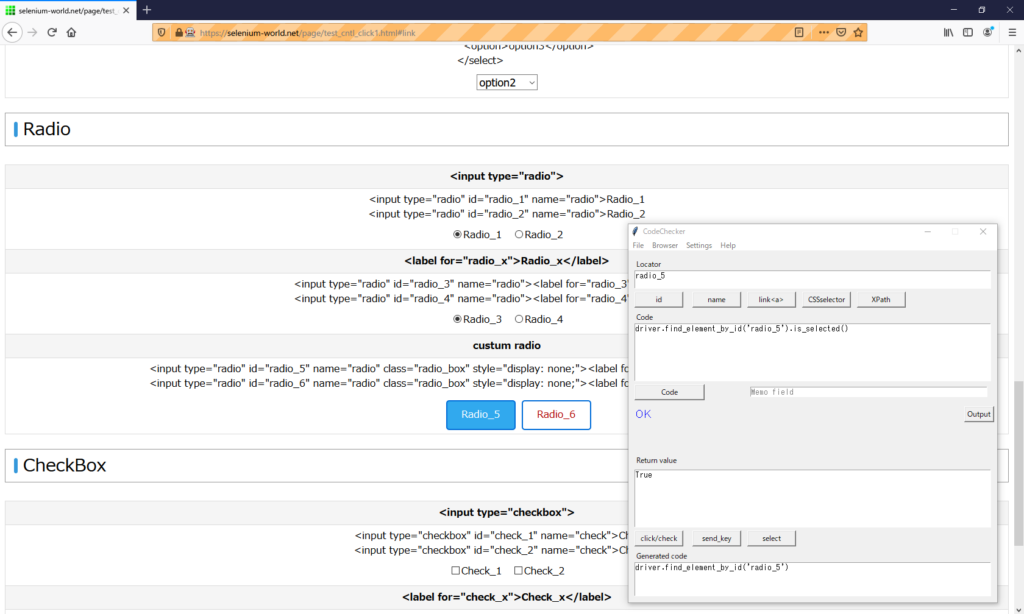
CheckBox
Exception occurred on Check_5.
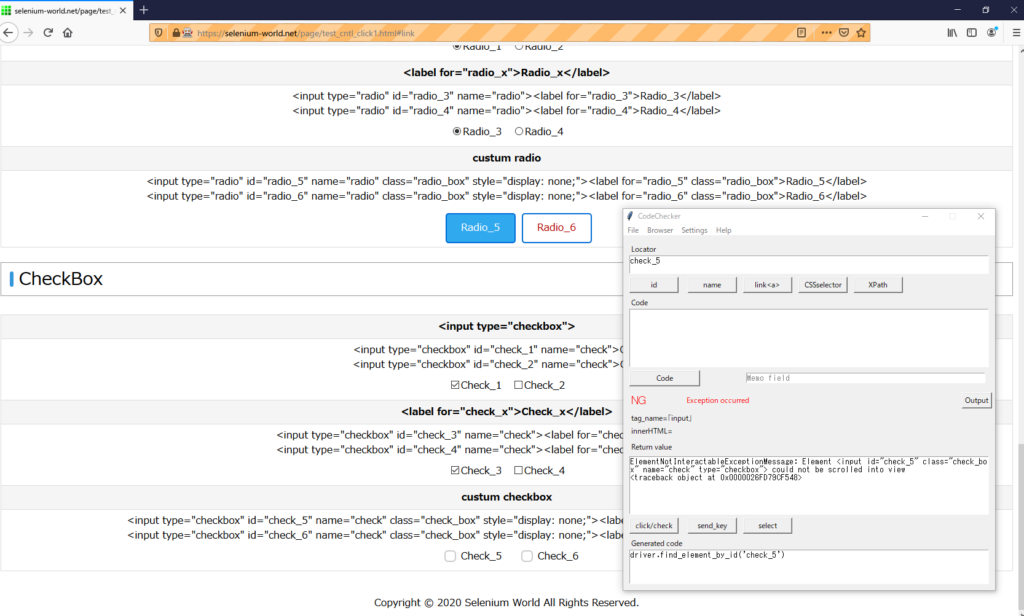
To select, click the label element.
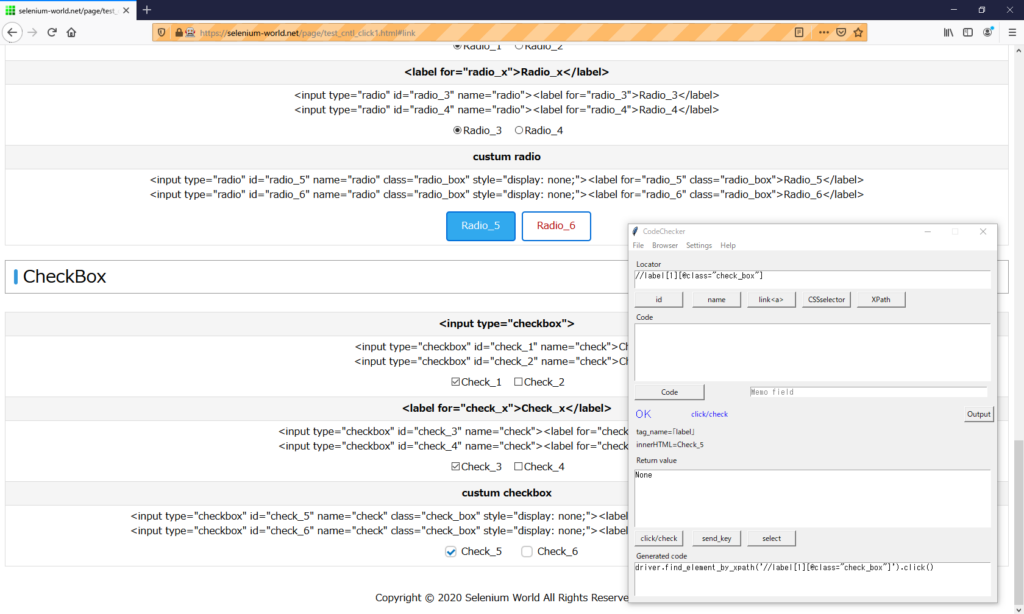
Get the selected state of the radio element.
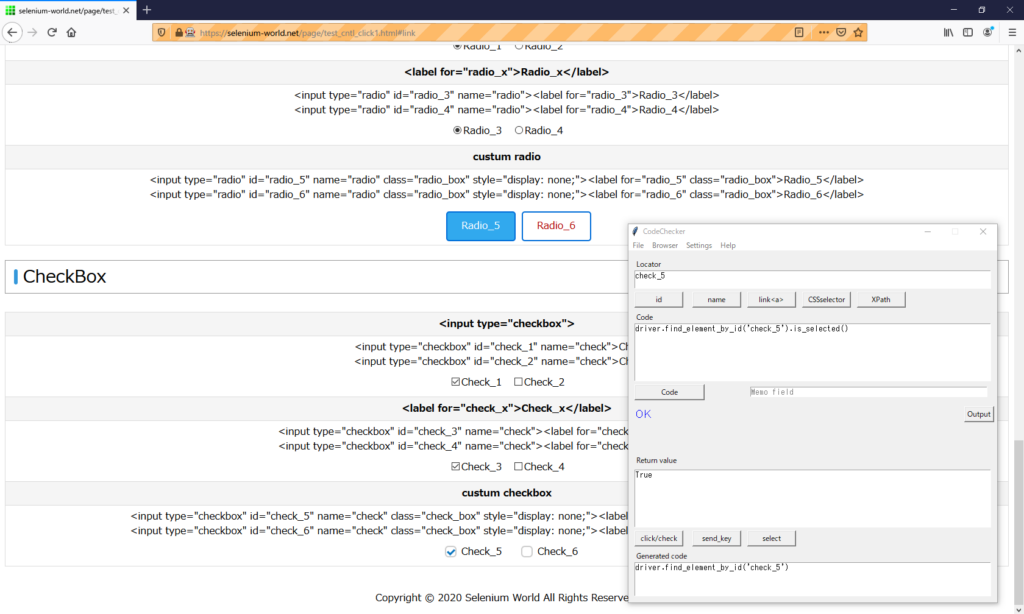
Microsoft Edge
Button, Link
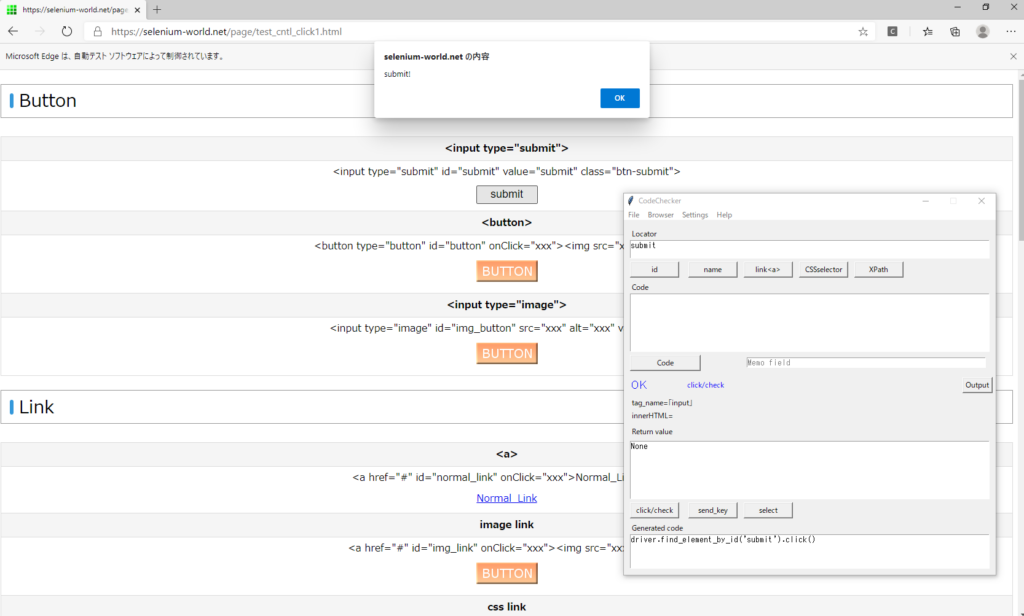
Text
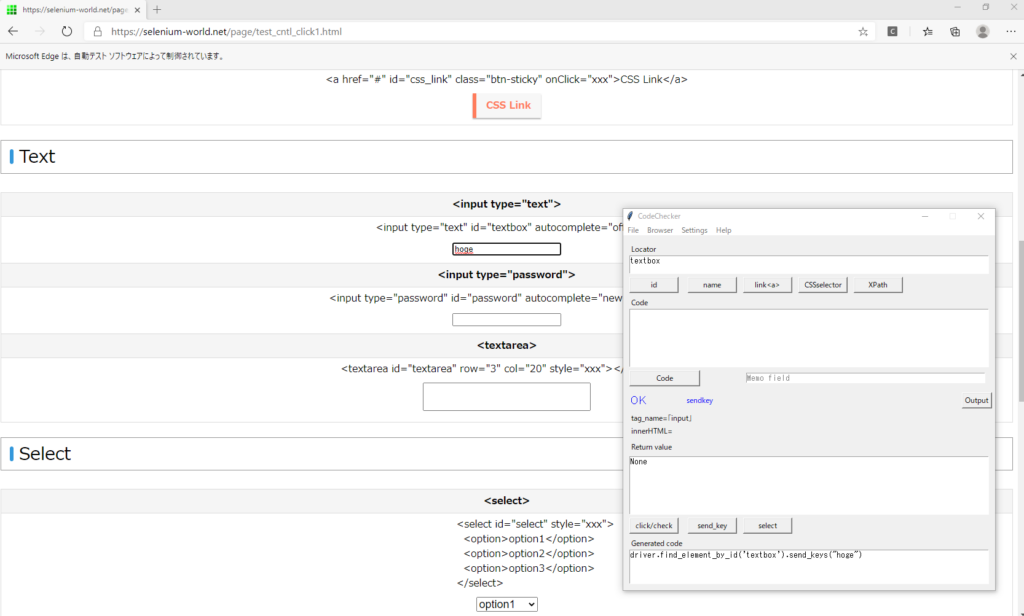
Select
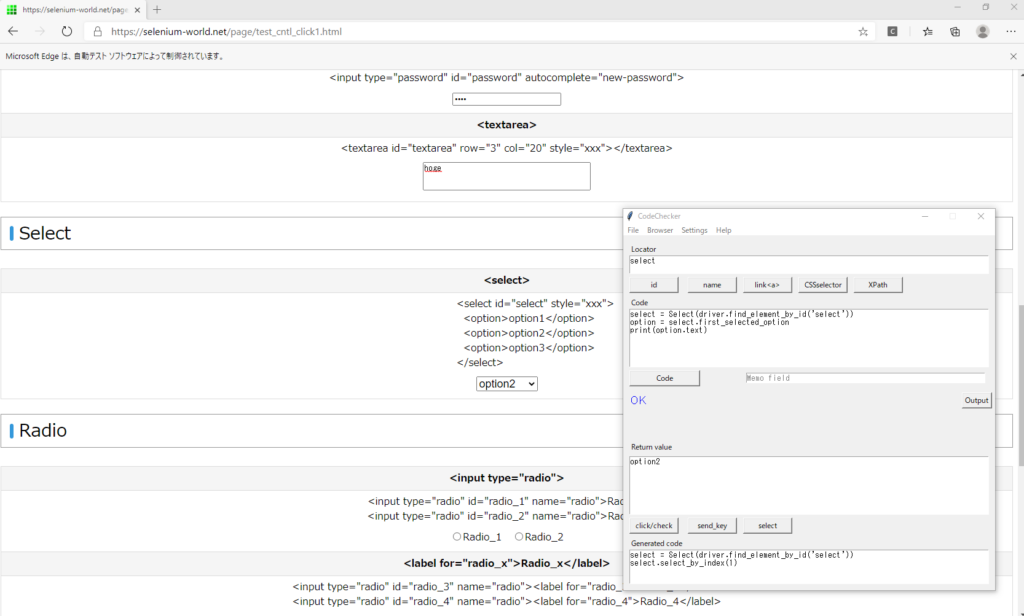
Radio, CheckBox
Exception occurred on Radio_5 and Check_5.
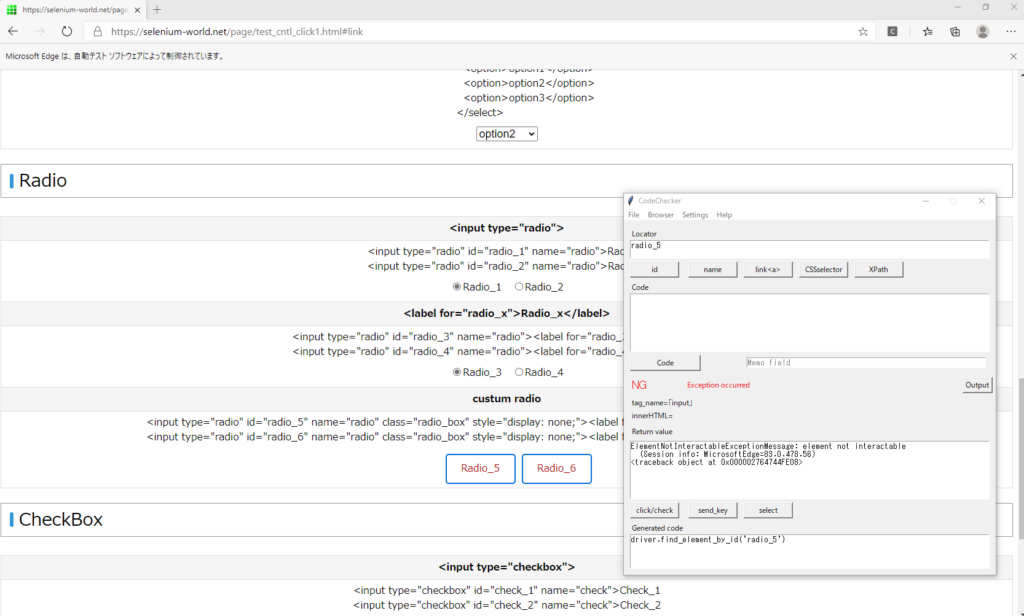
Message
ElementNotInteractableExceptionMessage: element not interactable
(Session info: MicrosoftEdge=83.0.478.56)
<traceback object at 0x000001ADAE237D48>
Get the XPath
Get the XPath of the label element to click by XPathGetter.
XPathGetter allows you to get the XPath in Internet Explorer and Microsoft Edge.
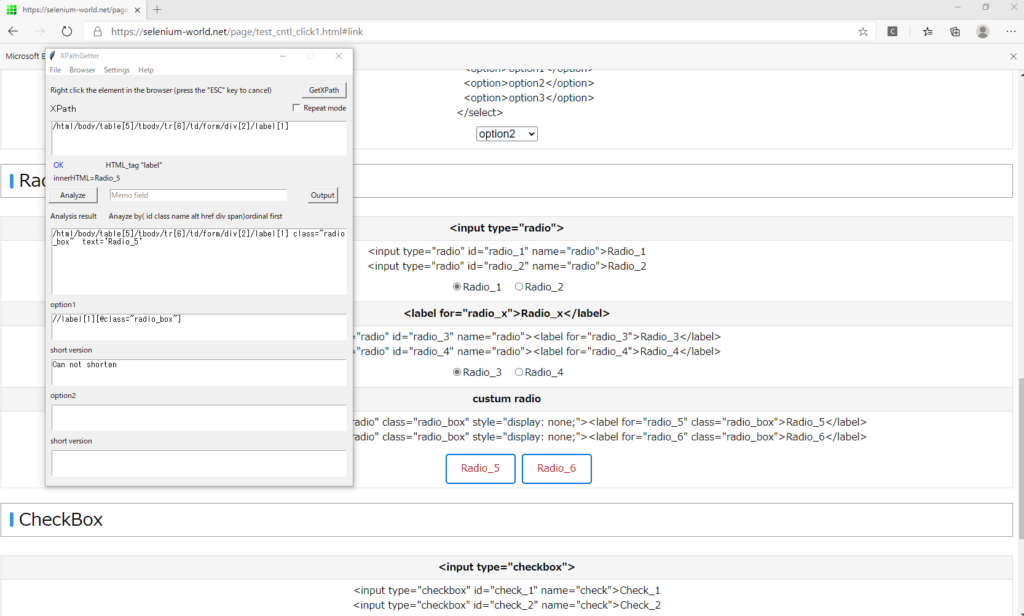
To select, click the label element.
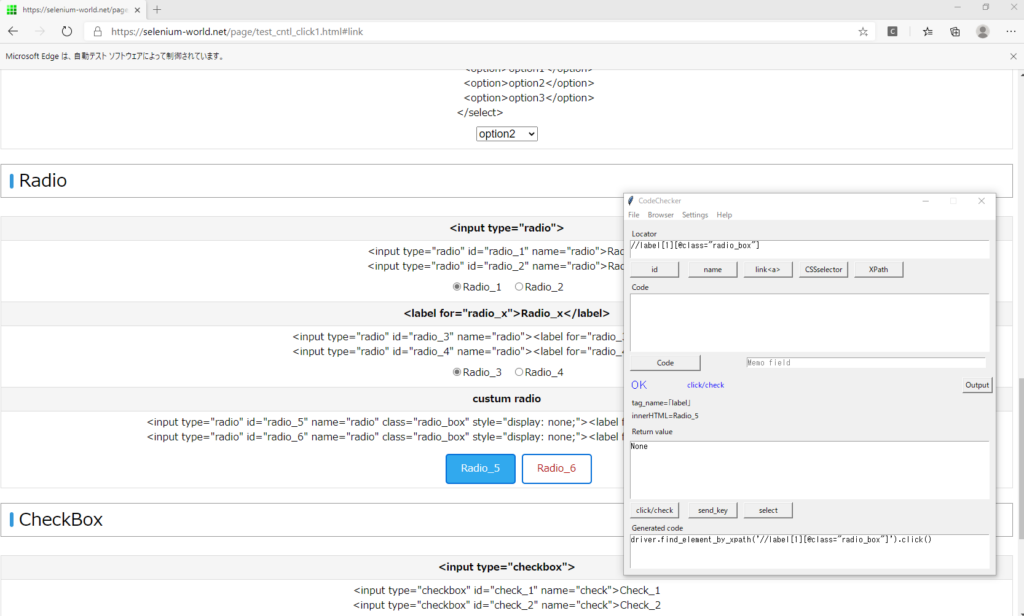
The selection state must be retrieved from radio or checkbox.
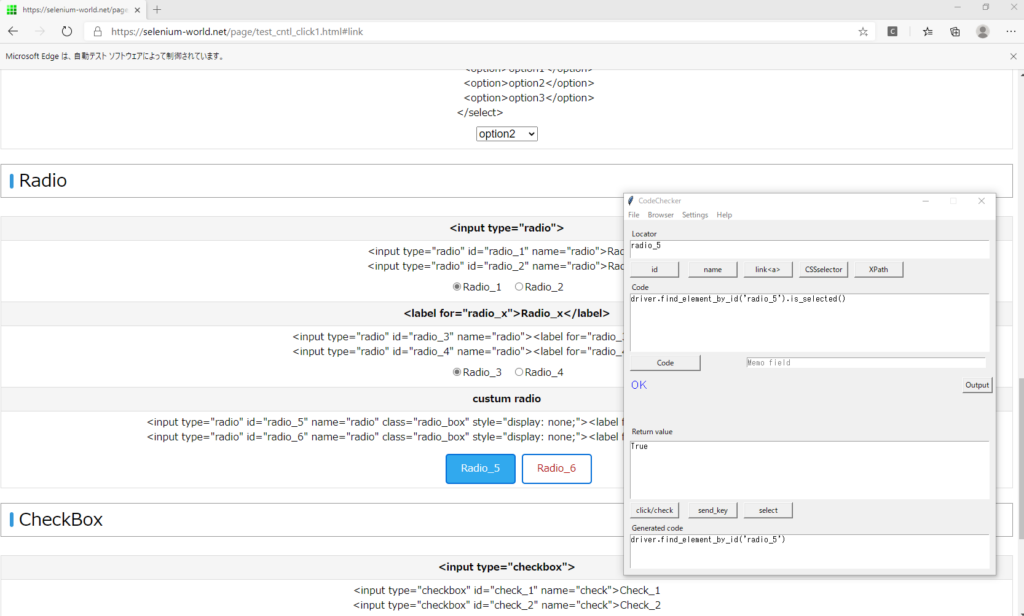
Internet Explorer
Button
In IE, the click method does not work simply.
The first click sets the focus to the control, and the second click raises the click event.
First
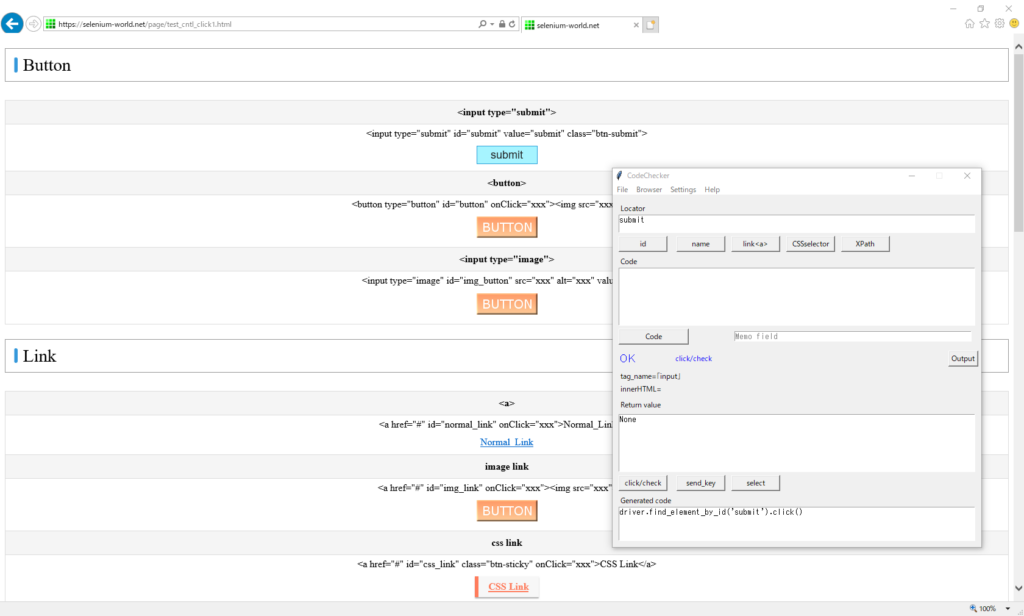
Second
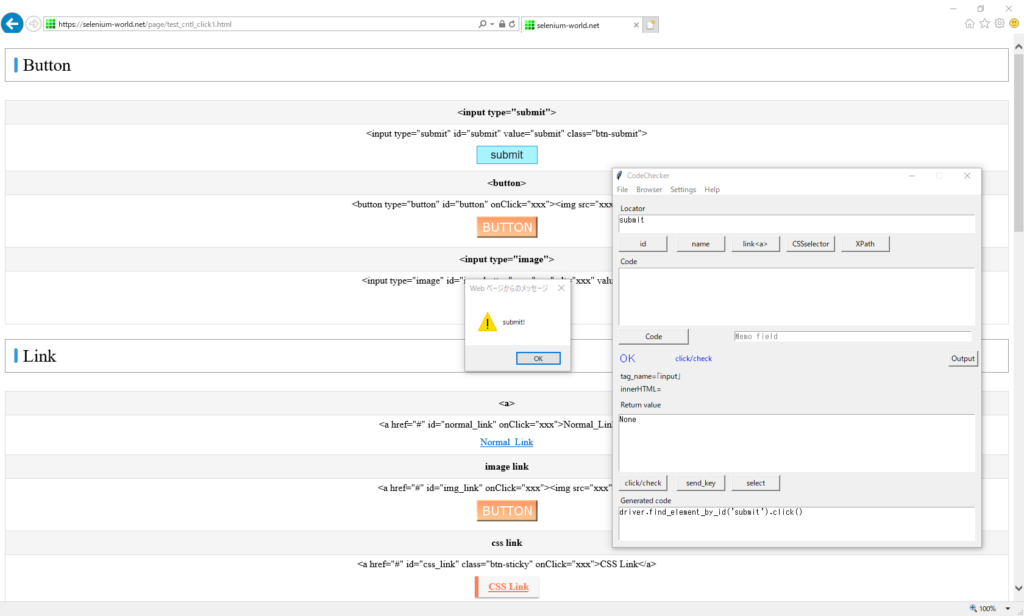
Link
The second click will cause the event.
First
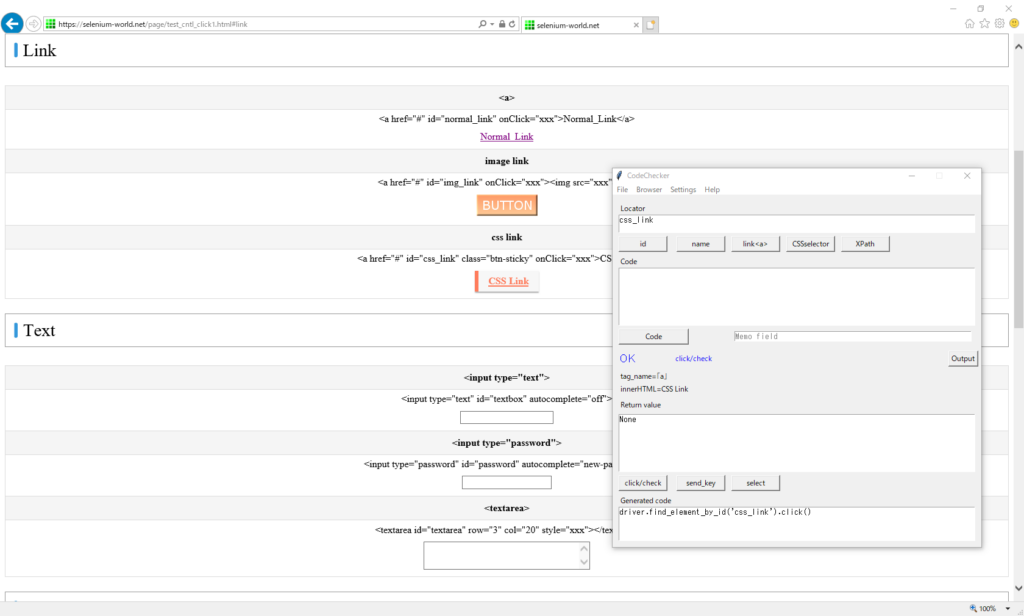
Second
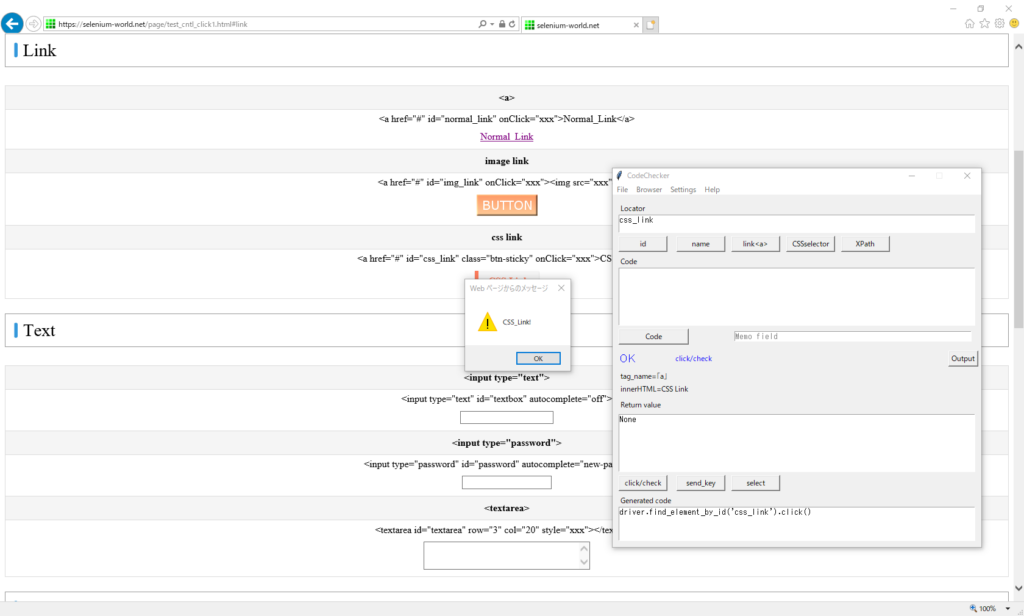
In addition, if the focus is on the screen in advance, such as by typing in a textbox, Selenium can click at once.
Click after entering text.
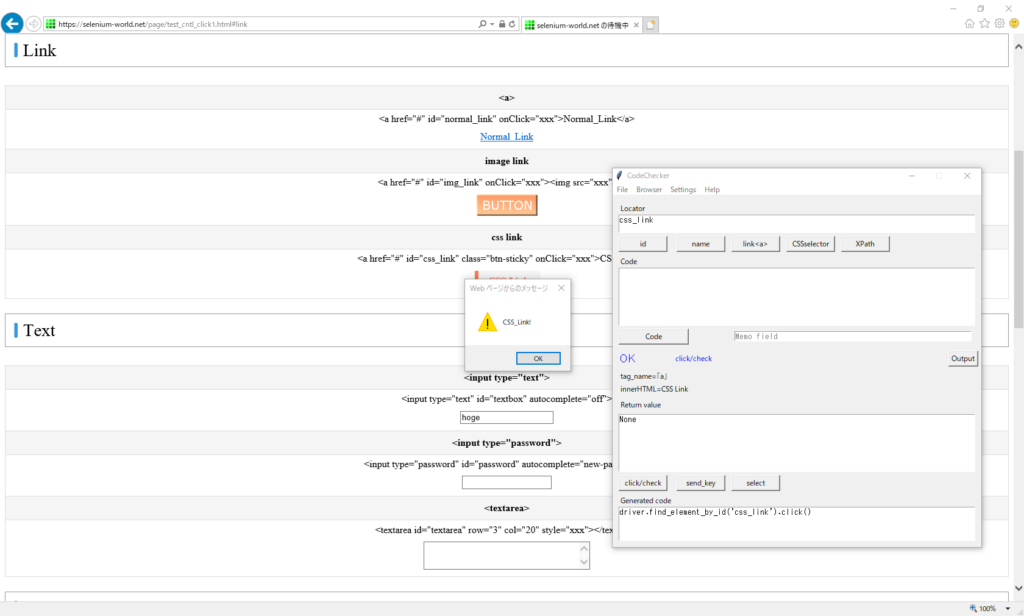
Text
Unlike click, send_key works fine.
Character input is activated the first time.
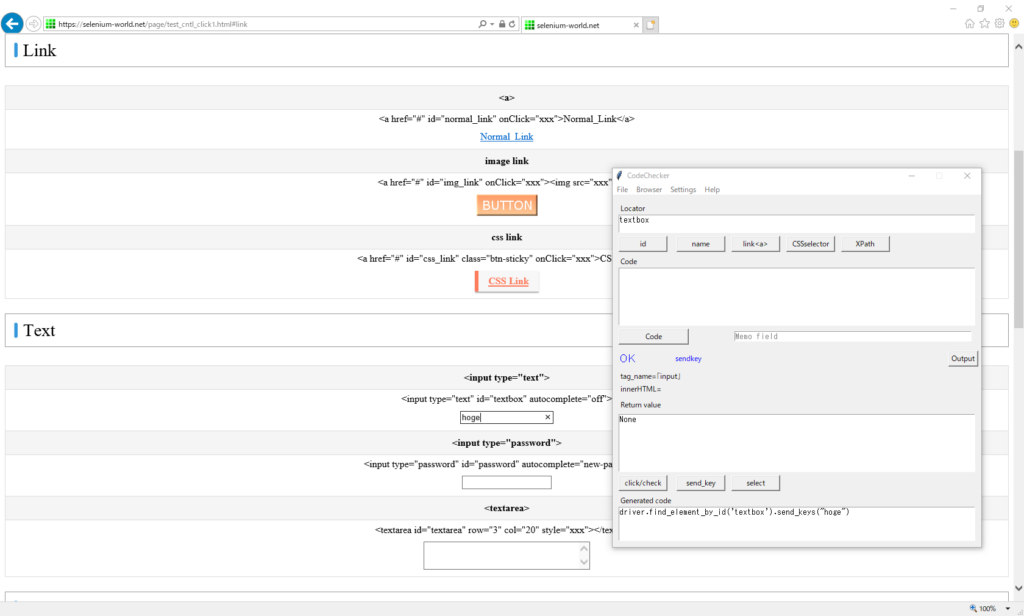
Just like a Button, if no foucus in the text box, the click event does not occur at the first time.
Select
It works fine.
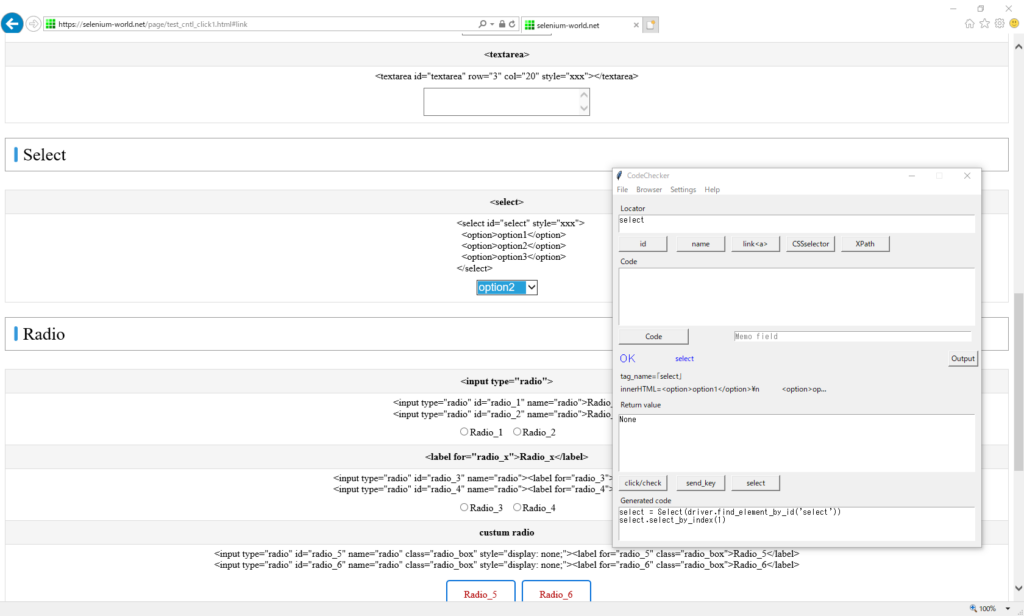
Get the selected item.
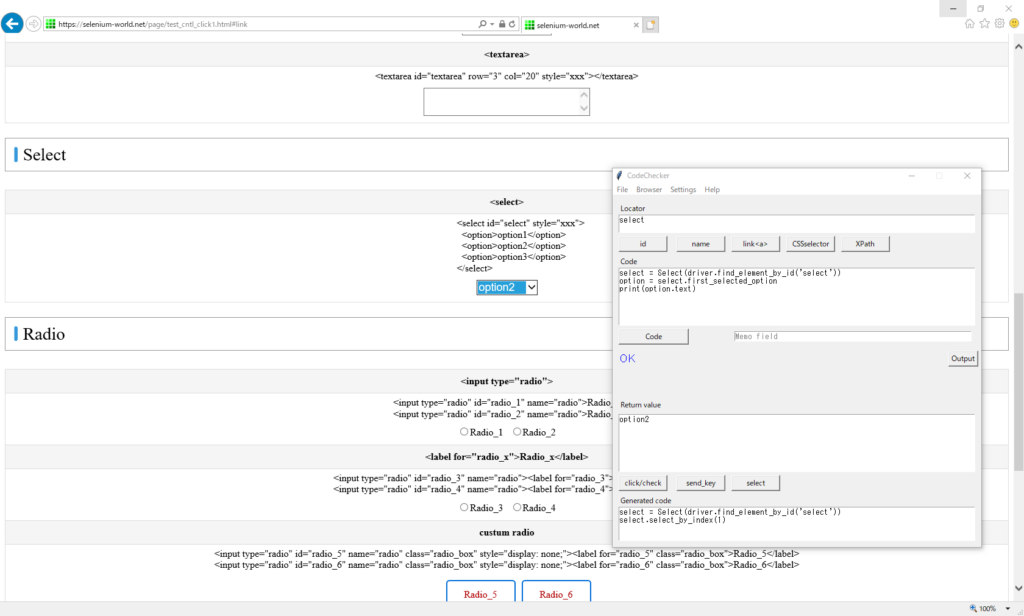
Radio
To select, click the element twice.
First
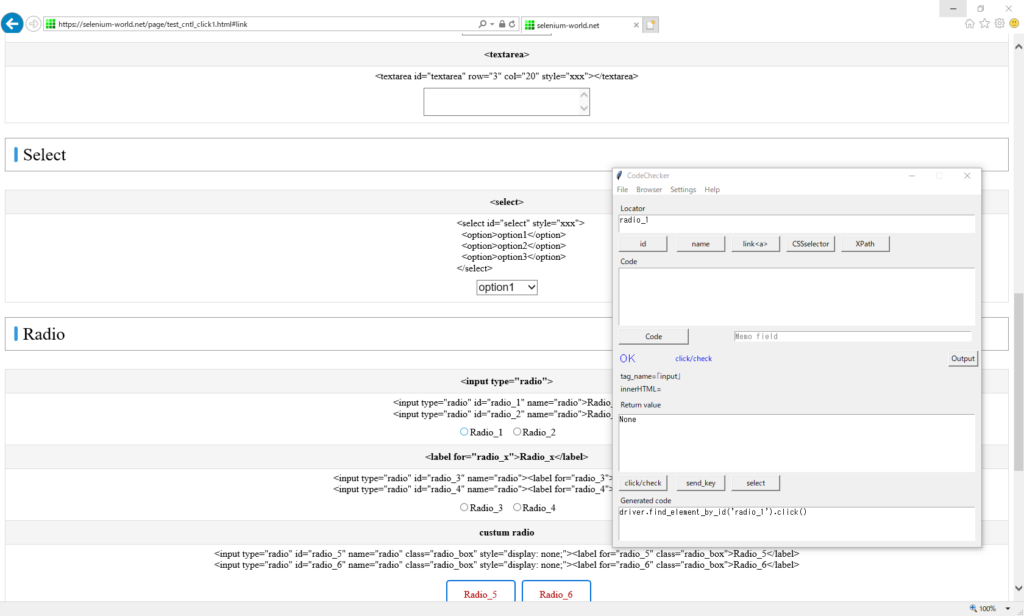
Second
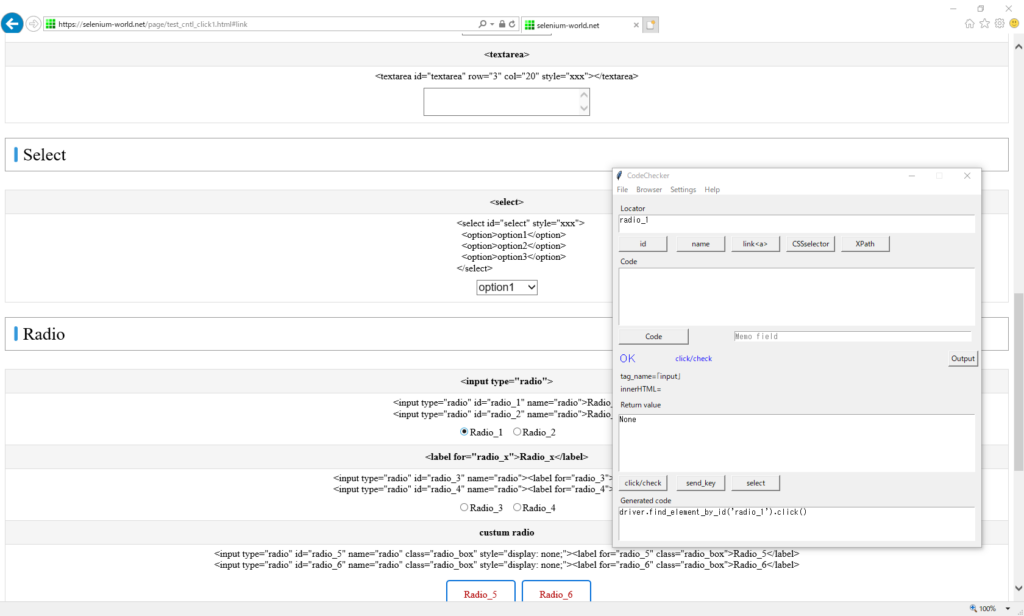
Custom Radio
It is the same as other browsers except to select it twice with a click.
Exception occurred with click the radio element.
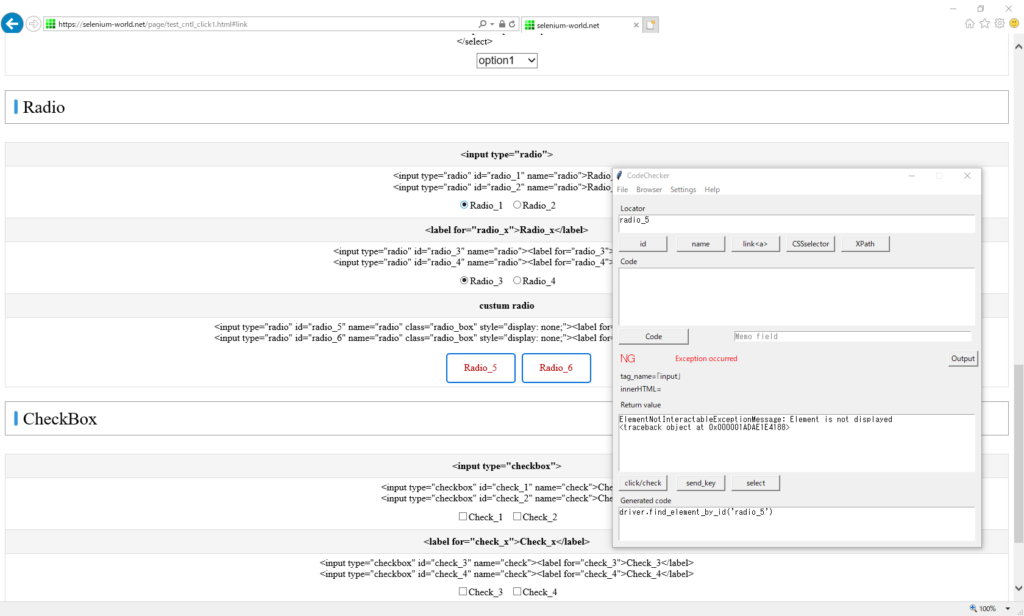
Message
ElementNotInteractableExceptionMessage: Element is not displayed
<traceback object at 0x000001ADAE1F1CC8>
Get the XPath
XPathGetter allows you to get the XPath in Internet Explorer and Microsoft Edge.
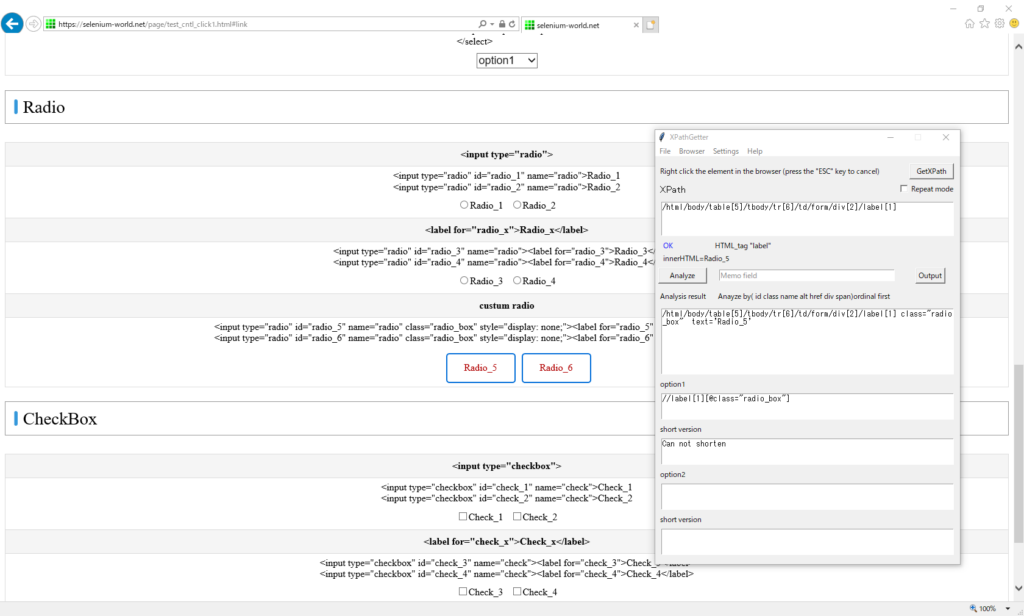
To select, click the label element twice.
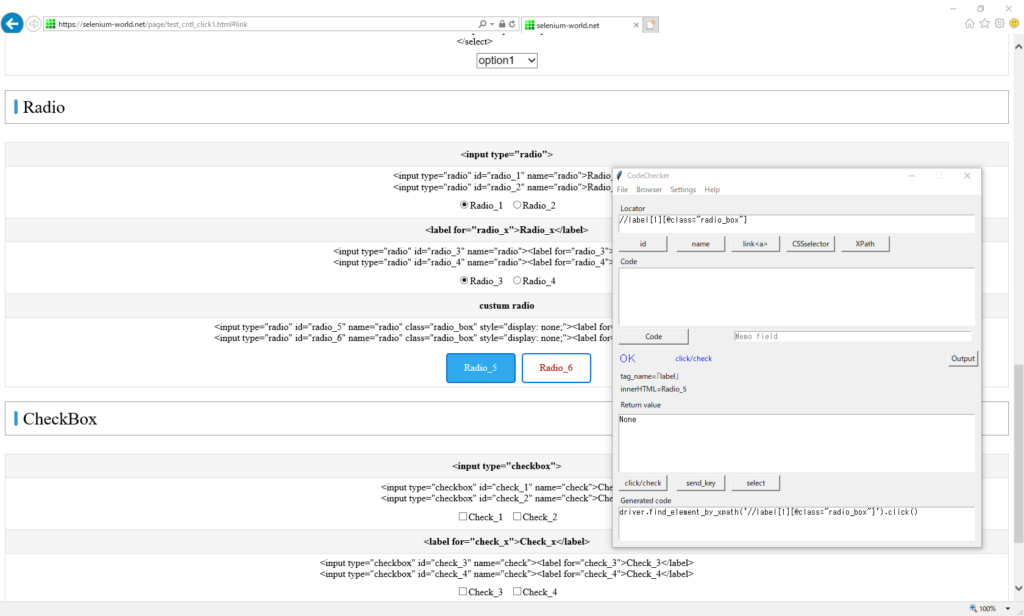
The label object has no a selected state.
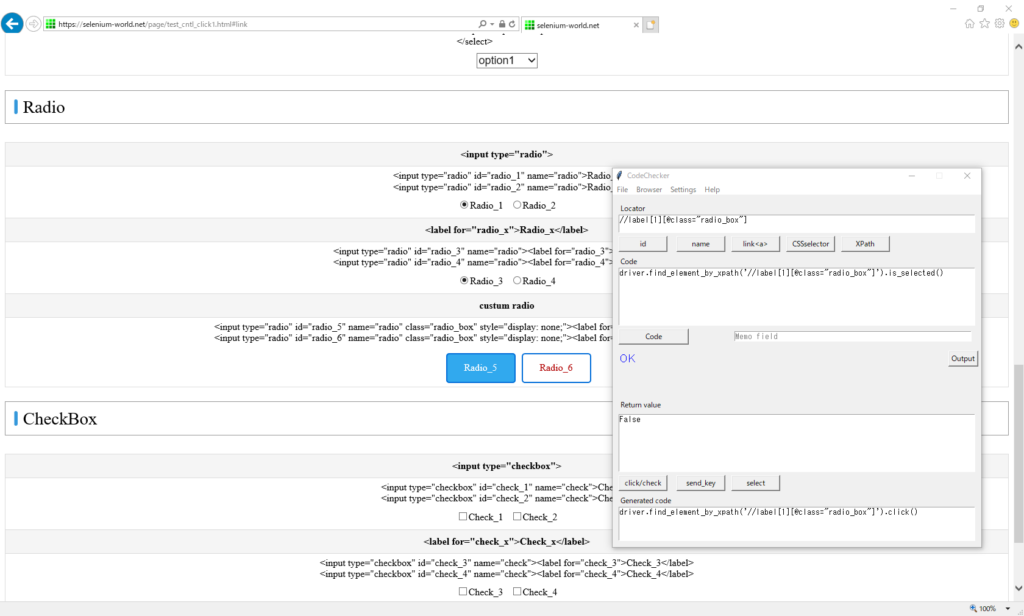
Get the selected state of the radio element.
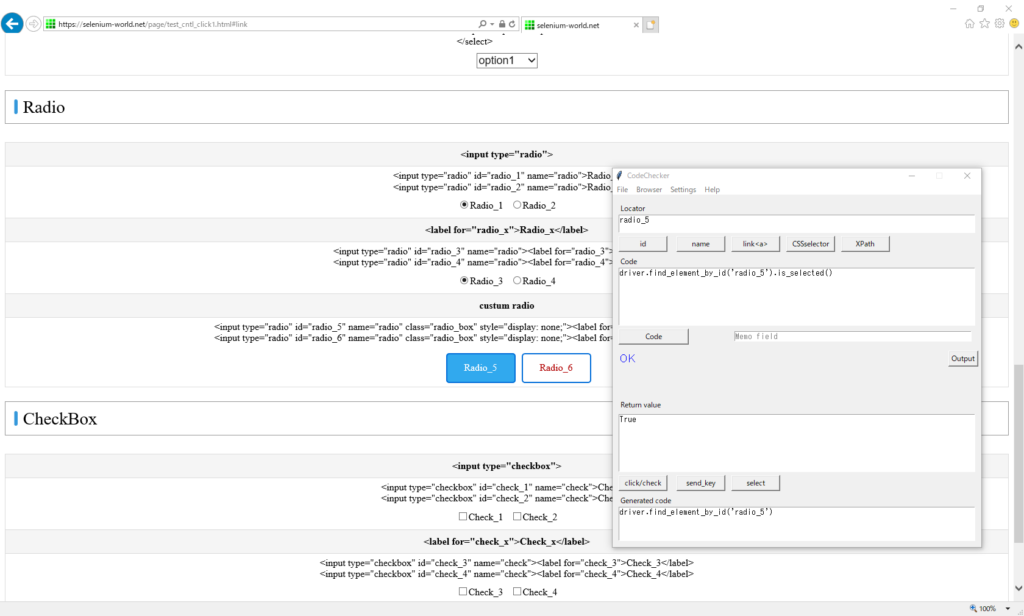
CheckBox
To select, click the element twice.
First
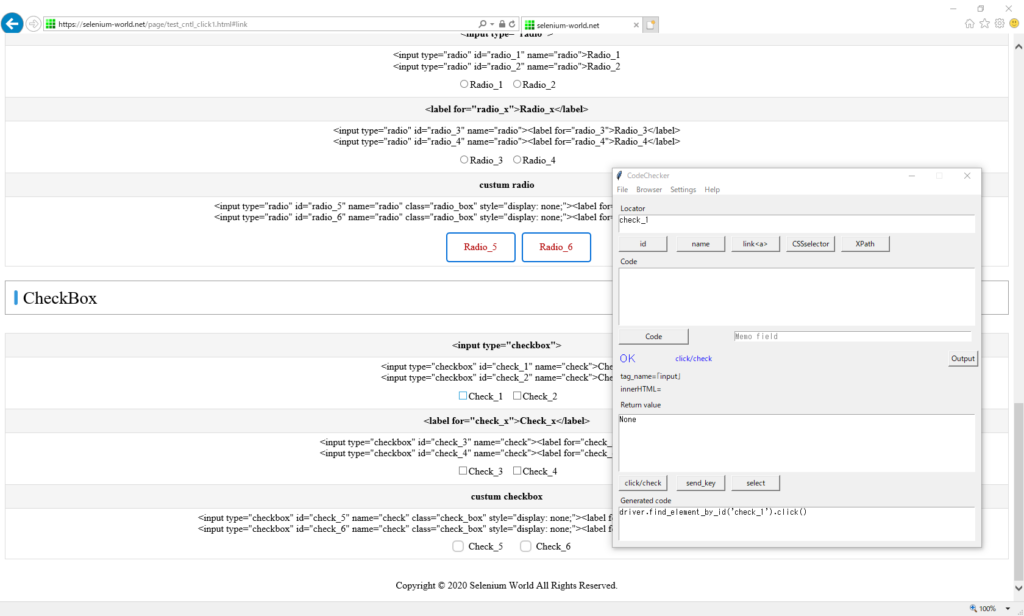
Second
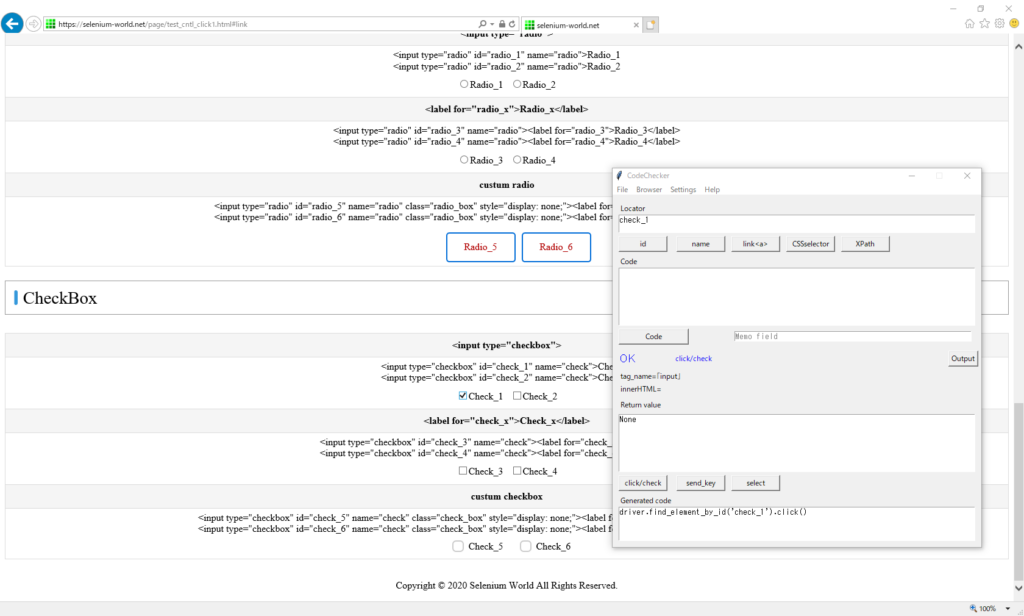
Custom CheckBox
Exception occurred on clicking the checkbox.
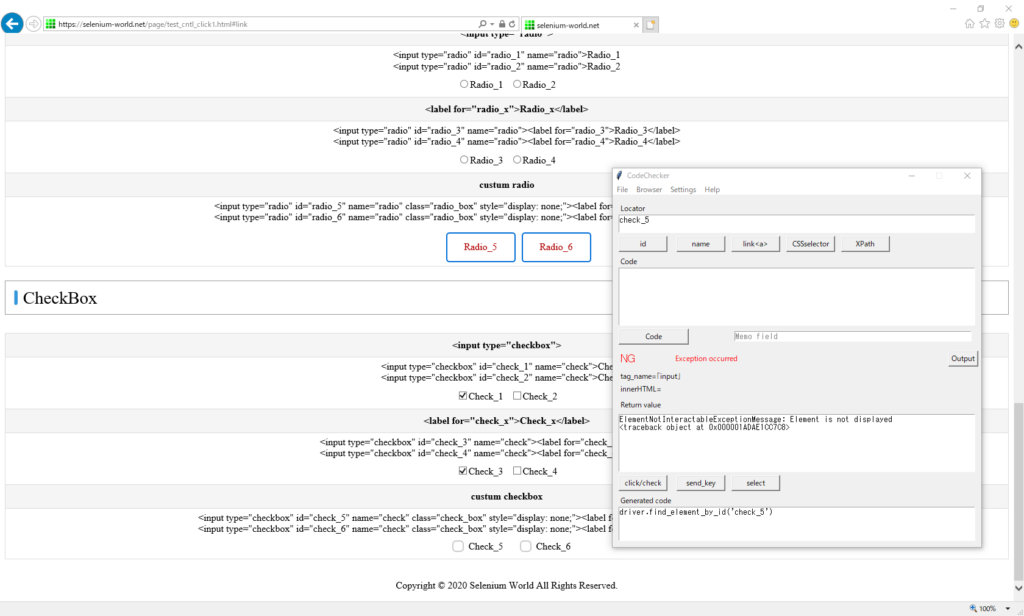
To select, click the label twice.
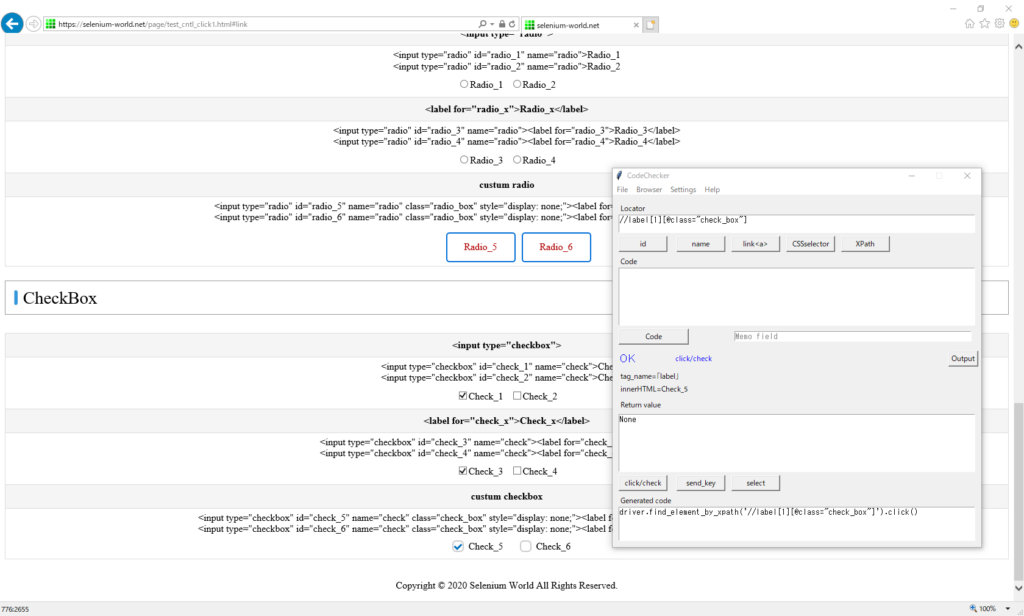
Get the selected state of the checkbox element.
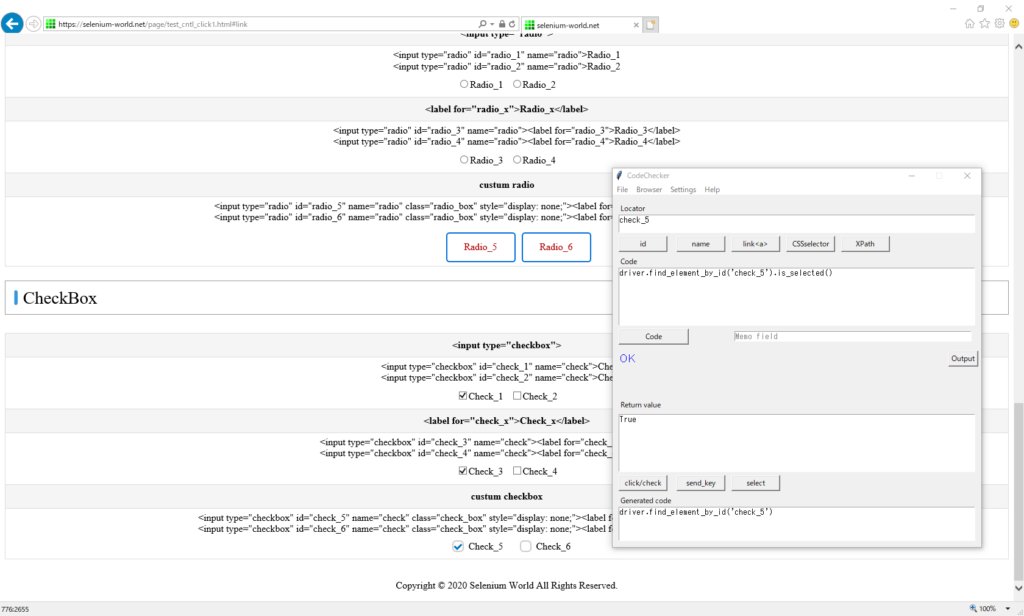
Execution result
- In each browser, the hidden element (display:none) could not be clicked.
- To click the element in IE, the focus need be on the screen in advance.
Button
id | operation | Ch | Fx | Ed | IE |
---|---|---|---|---|---|
submit | click() | OK | OK | OK | OK* |
button | click() | OK | OK | OK | OK* |
img_button | click() | OK | OK | OK | OK* |
*To click the element in IE, the focus need be on the screen in advance.
Link
id | operation | Ch | Fx | Ed | IE |
---|---|---|---|---|---|
normal_link | click() | OK | OK | OK | OK* |
img_link | click() | OK | OK | OK | OK* |
css_link | click() | OK | OK | OK | OK* |
*To click the element in IE, the focus need be on the screen in advance.
Text
id | operation | Ch | Fx | Ed | IE |
---|---|---|---|---|---|
textbox | send_keys(“hoge") | OK | OK | OK | OK |
get_attribute(“value") | OK | OK | OK | OK | |
password | send_keys(“hoge") | OK | OK | OK | OK |
get_attribute(“value") | OK | OK | OK | OK | |
textarea | send_keys(“hoge") | OK | OK | OK | OK |
get_attribute(“value") | OK | OK | OK | OK |
Select
id | operation | Ch | Fx | Ed | IE |
---|---|---|---|---|---|
select | select_by_index(1) | OK | OK | OK | OK |
first_selected_option | OK | OK | OK | OK |
Radio
id | operation | Ch | Fx | Ed | IE |
---|---|---|---|---|---|
radio_1 | click() | OK | OK | OK | OK* |
is_selected() | OK | OK | OK | OK | |
radio_3 | click() | OK | OK | OK | OK* |
is_selected() | OK | OK | OK | OK | |
radio_5 (display:none) |
click() | NG | NG | NG | NG |
is_selected() | OK | OK | OK | OK |
*To click the element in IE, the focus need be on the screen in advance.
CheckBox
id | operation | Ch | Fx | Ed | IE |
---|---|---|---|---|---|
check_1 | click() | OK | OK | OK | OK* |
is_selected() | OK | OK | OK | OK | |
check_3 | click() | OK | OK | OK | OK* |
is_selected() | OK | OK | OK | OK | |
check_5 (display:none) |
click() | NG | NG | NG | NG |
is_selected() | OK | OK | OK | OK |
*To click the element in IE, the focus need be on the screen in advance.
Discussion
New Comments
No comments yet. Be the first one!